AJAX is an acronym for Asynchronous JavaScript and XML. The key element here is Asynchronous. Simply put, AJAX offers a technique to make background server calls via JavaScript and retrieve additional data as needed, updating portions of the page without causing full page reloads. Figure 1-4 offers a visual representation of what happens when a typical AJAX-enabled web page is requested by a visitor:
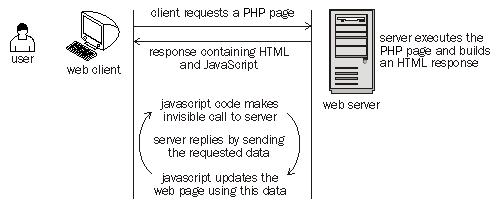
Figure 1-4: A typical AJAX call
AJAX solves the balance between the client and server by allowing them to communicate in the background while the user is working on the page.
Consider web registration forms where the user is asked to enter data (such as name, email address, password, credit card number, and so on) that must be validated before proceeding to the next step of the registration process. There are three possible ways to implement this:
Let the user type all the required data, submit the page, and then perform the validation on the server. If the validation doesn't succeed, the server sends back the (sometimes empty) form, asking the visitor to correct the invalid entries. In this scenario, the user experiences dead time (a delay) between submitting and waiting for response.
Do the validation at the client side by using JavaScript. The user is warned about invalid data and corrects the invalid entries before submitting the form. This technique only works for very simple validation that doesn't require additional data from the server. This technique also doesn't work when using proprietary or secret validation algorithms that can't be transferred to the client in the form of JavaScript code.
Use AJAX form validation so that the web application can validate the entered data in the background, while the user fills the form. For example, after the user types the first letter of the city, the web browser calls the server to load "on-the-fly" a list of cities that start with that letter.
When we wrote the first edition of this book, there were only a few AJAX-enabled applications on the Web. Now, the majority of modern websites have implemented AJAX features. Here are a few of the most popular:
Bing Maps (http://www.bing.com/maps/), Google Maps (http://maps.google.com), and Yahoo! Maps (http://maps.yahoo.com).
Flickr (http://flickr.com/) and Picasa Web Albums (http://picasaweb.google.com/home).
The Google (http://www.google.com) and Yahoo! (http://search.yahoo.com) search engines with their query autocompletion feature. See the Google version in the following screenshot (yes, the results can be funny sometimes).
Gmail (http://www.gmail.com), which is very popular by now and doesn't need any introduction. Other web-based email services such as Yahoo! Mail and Hotmail have followed the trend and offer AJAX-based interfaces.
Digg (http://www.digg.com), a hugely popular social bookmarking website featuring community‑powered content.
Figre 1-5 displays the Google autocompletion feature:
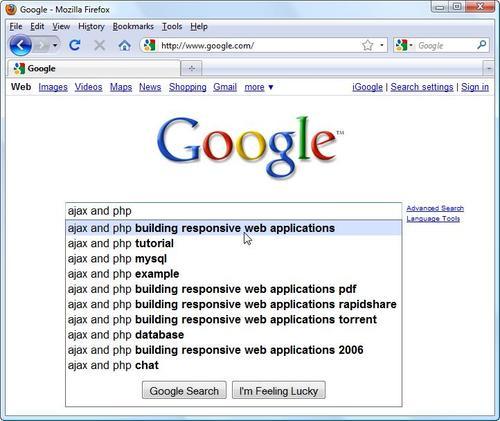
Figure 1-5: Google autocompletion feature
In conclusion, AJAX is about creating smarter web applications (that behave better than traditional web applications when interacting with humans) by enabling web pages to make asynchronous calls to the server transparently while the user is working.
The technologies AJAX is made of are already implemented in all modern web browsers, so the client doesn't need to install any extra modules to run an AJAX website. AJAX is made up of the following:
JavaScript, the essential ingredient of AJAX, allows you to build the client-side functionality. In the JavaScript functions, we'll use the Document Object Model (DOM) to manipulate parts of the HTML page.
The XMLHttpRequest object, the component that enables JavaScript to access the server asynchronously in the background.
Except for the simplest applications, a server-side technology is required to handle requests that come from the JavaScript client. In this book, we'll use PHP to perform the server-side part of the job.
Note
None of the AJAX components are as new, or revolutionary (or at least evolutionary), as the buzz around AJAX might suggest. The most recent AJAX component is XMLHttpRequest
, which was released by Microsoft sometime in 1999. You can read more on the history of AJAX at http://en.wikipedia.org/wiki/AJAX.
For client-server communication, the JavaScript client code and the PHP server-side code need a way to pass data and understand that data. Passing the data is the simple part. Using the XMLHttpRequest
object, the client script accessing the server can send name-value pairs using GET or POST. It's very simple to read these values with any server script.
The server script simply sends back the response via HTTP, but unlike a usual website, the response will be in a format that can be simply parsed by the JavaScript client code. The format can be simple text, but in practice, you'll need a data format that can be used to pass structured data. The two popular data exchange formats used in AJAX applications are XML and JavaScript Object Notation (JSON).
This book assumes that you have previous experience with the AJAX ingredients, except maybe the XMLHttpRequest
object. However, in order to make sure we're all on the same page, we'll have a look at how these pieces work, and how they work together, in Chapter 2, JavaScript and the AJAX Client. For the remainder of this chapter, we'll focus on the big picture, and for the joy of the most eager readers, we will also write an AJAX program.
As noted earlier, AJAX can improve your visitors' experience with your website, but it can also worsen it when used inappropriately. Unless your application has really special requirements, it's wise to let your users navigate your content using good old hyperlinks. Web browsers have a long history of dealing with content navigation, and web users have a long history of using these browsers. In the vast majority of cases, AJAX is best used in addition to the traditional web development paradigms, rather than changing or replacing them.
Let's quickly review the potential benefits that AJAX can bring to your projects:
It makes it possible to create responsive and intuitive web applications
It encourages the development of patterns and frameworks that reduce the development time of common tasks
It makes use of the existing technologies and features that are already supported by all modern web browsers
It makes use of many existing developer skills
Potential problems with AJAX are:
Adding AJAX to your site without forethought or reason can detract from your site's effectiveness. Increased awareness of usability, accessibility, web standards, and search engine optimization will help you make good decisions when designing and implementing websites.
Because search engines don't execute any JavaScript code when indexing a website, they cannot index any content generated with JavaScript. If search engine optimization is important for your website, you may need to forego using AJAX for content delivery and navigation and use it only sparingly in those parts of your site that won't impact search engine indexing.
JavaScript can be disabled at the client side, which renders the AJAX code non-functional.
Bookmarking AJAX-enabled pages requires planning. Typically AJAX applications run inside a web page whose URL doesn't change in response to user actions, in which case, you can only bookmark the entry page. To enable bookmarking, you must dynamically add page anchors by using your JavaScript code, such as in
http://www.example.com/my-ajax-app.html#Page2
. You also need to create supporting code that loads and saves the state of your application through theanchor
parameter.The Back and Forward buttons in browsers don't produce the same result as with classic websites, unless your AJAX application is programmed to support loading and saving states.
To enable AJAX page bookmarking, and the Back and Forward browser buttons, you can use frameworks such as Really Simple History by Brad Neuberg (http://codinginparadise.org/projects/dhtml_history/README.html).
Following the popularity of AJAX, a large number of AJAX-enabled frameworks and toolkits have been developed that include common and tested features. Let's take a look at a few.
AJAX enjoys an active community and a veritable plethora of resources, guides, toolkits, frameworks, forums, and tutorials. Whether you're a veteran developer or working with AJAX for the first time, it's well worth your time to peruse these resources.
We are listing a few places to get you started that may help you in your journey into the exciting world of AJAX. Some are server-agnostic, while others are specifically created for ASP.NET, Java, PHP, Coldfusion, Flash, and Perl backends. Among the most popular server-agnostic toolkits are Dojo (http://dojotoolkit.org), Prototype (http://prototypejs.org/), script.aculo.us (http://script.aculo.us), and jQuery (http://jquery.com/)—which you'll be using in this book as well.
For starters, here are a few useful generic AJAX resources:
http://www.ajaxian.com is the AJAX website of Ben Galbraith and Dion Almaer, the authors of Pragmatic Ajax (Pragmatic Bookshelf, 2006).
http://ajaxpatterns.org is an informational website about AJAX design patterns, and the home page of Ajax Design Patterns by Michael Mahemoff (O'Reilly, 2006).
http://www.fiftyfoureleven.com/resources/programming/xmlhttprequest is a comprehensive article collection about AJAX.
http://www.sitepoint.com/subcat/javascript is Sitepoint's AJAX home, featuring excellent articles.
http://developer.mozilla.org/en/docs/AJAX is Mozilla's page on AJAX.
http://en.wikipedia.org/wiki/Ajax is the Wikipedia page on AJAX.
The list is by no means complete. If you need more online resources, search engines will be of help.