Cryptography is the science of converting plain simple text into secret, hidden, meaningful text, and vice-versa. It also helps in transmitting and storing data that cannot be easily deciphered using owned keys.
There are the following two types of cryptography in computing:
- Symmetric
- Asymmetric
Symmetric cryptography refers to the process of using a single key for both encryption and decryption. It means the same key should be available to multiple people if they want to exchange messages using this form of cryptography.
Asymmetric cryptography refers to the process of using two keys for encryption and decryption. Any key can be used for encryption and decryption. Message encryption with a public key can be decrypted using a private key and messages encrypted by a private key can be decrypted using a public key. Let's understand this with the help of an example. Tom uses Alice's public key to encrypt messages and sends it to Alice. Alice can use her private key to decrypt the message and extract contents out of it. Messages encrypted with Alice's public key can only be decrypted by Alice as only she holds her private key and no one else. This is the general use case of asymmetric keys. There is another use which we will see while discussing digital signatures.
Hashing is the process of transforming any input data into fixed length random character data, and it is not possible to regenerate or identify the original data from the resultant string data. Hashes are also known as fingerprint of input data. It is next to impossible to derive input data based on its hash value. Hashing ensures that even a slight change in input data will completely change the output data, and no one can ascertain the change in the original data. Another important property of hashing is that no matter the size of input string data, the length of its output is always fixed. For example, using the SHA256 hashing algorithm and function with any length of input will always generate 256 bit output data. This can especially become useful when large amounts of data can be stored as 256 bit output data. Ethereum uses the hashing technique quite extensively. It hashes every transaction, hashes the hash of two transactions at a time, and ultimately generates a single root transaction hash for every transaction within a block.
Another important property of hashing is that it is not mathematically feasible to identify two different input strings that will output the same hash. Similarly, it is not possible to computationally and mathematically find the input from the hash itself.
Ethereum used Keccak256
as its hashing algorithm.
The following screenshot shows an example of hashing. The input Ritesh Modi
generates a hash, as shown in the following screenshot:
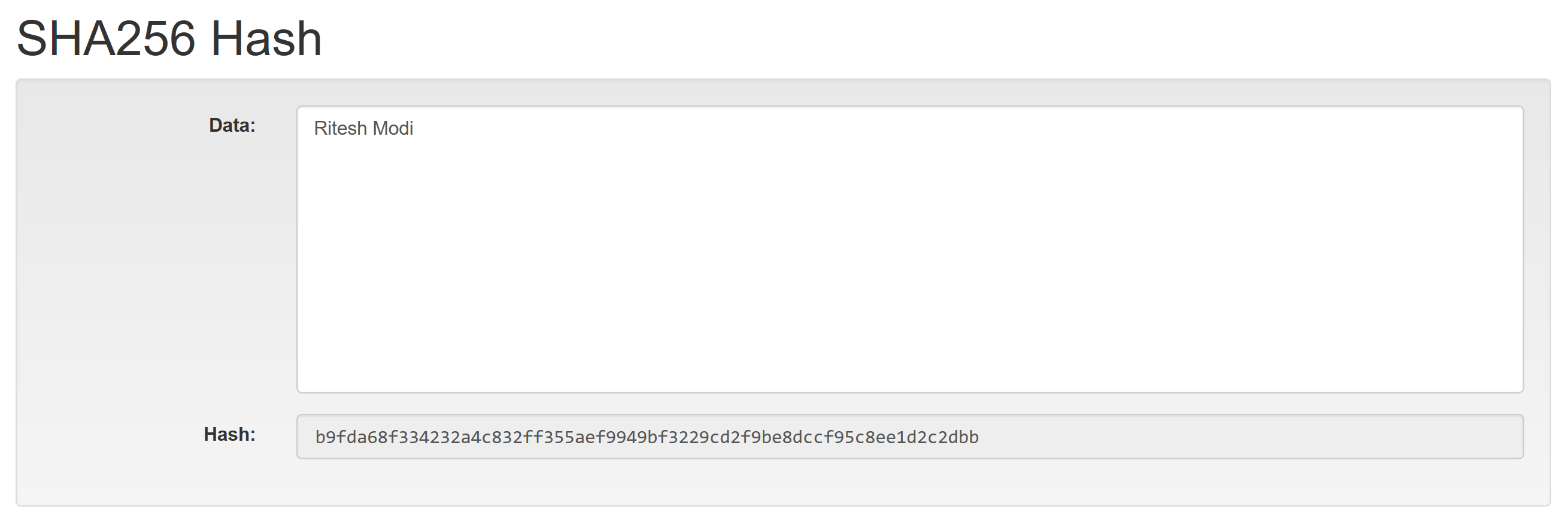
Even a small modification to input generates a completely different hash, as shown in the following screenshot:
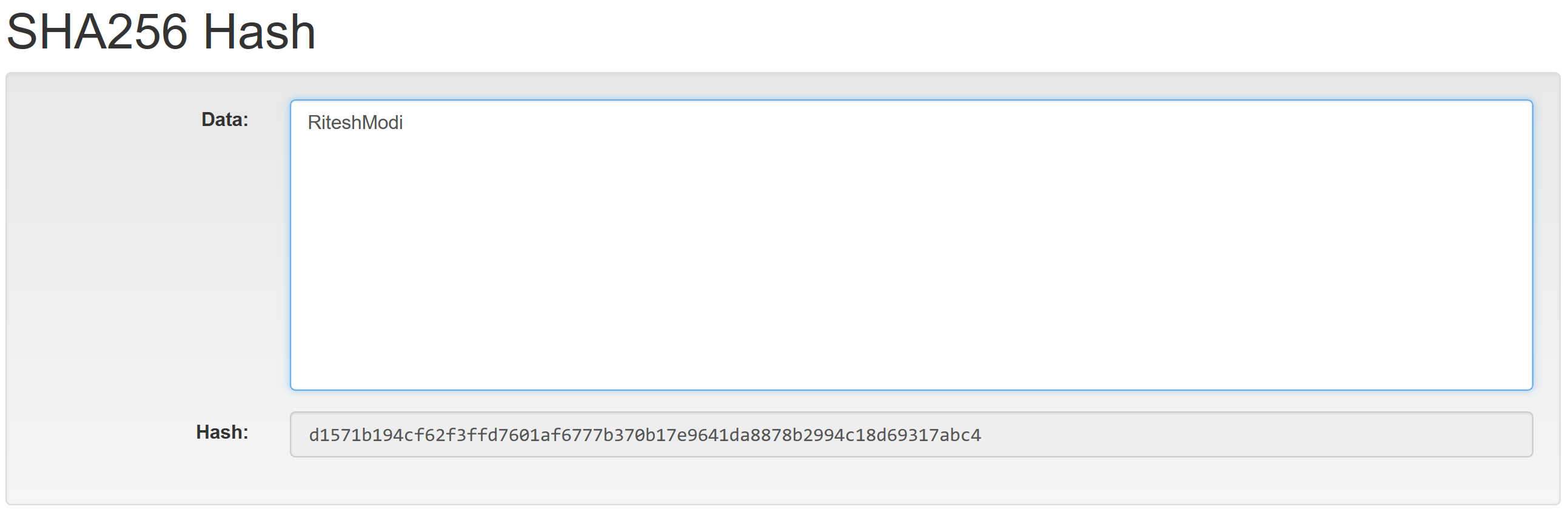
Earlier, we discussed cryptography using asymmetric keys. One of the important cases for using asymmetric keys is in the creation and verification of a digital signature. Digital signatures are very similar to a signature done by an individual on a piece of paper. Similar to a paper signature, a digital signature helps in identifying an individual. It also helps in ensuring that messages are not tampered with in transit. Let's understand digital signatures with the help of an example.
Alice wants to send a message to Tom. How can Tom identify and ensure that the message has come from Alice only and that the message has not been changed or tampered with in transit? Instead of sending a raw message/transaction, Alice creates a hash of the entire payload and encrypts the hash with her private key. She appends the resultant digital signature to the hash and transmits it to Tom. When the transaction reaches Tom, he extracts the digital signature and decrypts it using Alice's public key to find the original hash. He also extracts the original hash from the rest of the message and compares both the hashes. If the hashes match, it means that it actually originated from Alice and that it has not been tampered with.
Digital signatures are used to sign transaction data by the owner of the asset or cryptocurrency, such as Ether.