Let's configure our hello world Lambda function and triggers using the awscli utility
command.
The AWS CLI supports all available AWS services. You can see a detailed description of the aws
command using aws help
and it will also list all the available services.
We are interested in Lambda, as we are going to create a Lambda function with a simple hello world context:
$ aws lambda help
This will list a complete description of the Lambda service and all the available commands that are required to manage the AWS Lambda service.
Here, we are going to create a new Lambda function with the aws lambda create-function
command. To run this command, we need to pass the required and optional arguments.
Make sure you have a role with permission for the lambda:CreateFunction
action.
Previously, in the AWS Lambda console, we chose the code entry point as inline editing. Now, we will be using a ZIP file as a deployment package.
Before creating the Lambda function, we should create a Lambda function deployment package.
This deployment package will be a ZIP file consisting of your code and any dependencies.
If your project has some dependencies, then you must install the dependencies in the root directive of the project. For example:
$ pip install requests -t <project-dir> OR $ pip install -r requirements.txt -t <project-dir>
Here, the -t
option indicates the target directory.
Create a simple lambda_handler
function in a file named as handler.py
, as shown in the following screenshot:
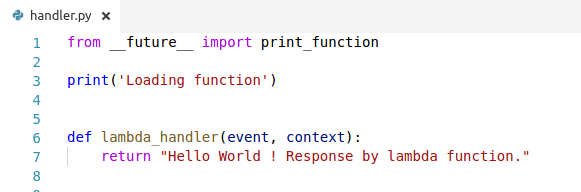
Now, let's make a deployment package as a ZIP file consisting of the preceding code:
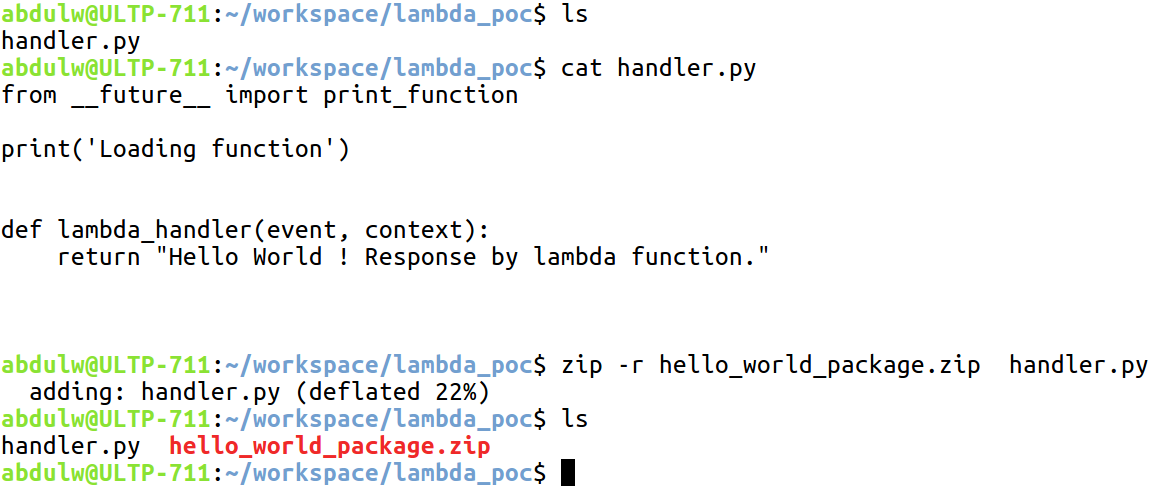
Now, we are ready to create the Lambda function. The following screenshot describes the command execution:
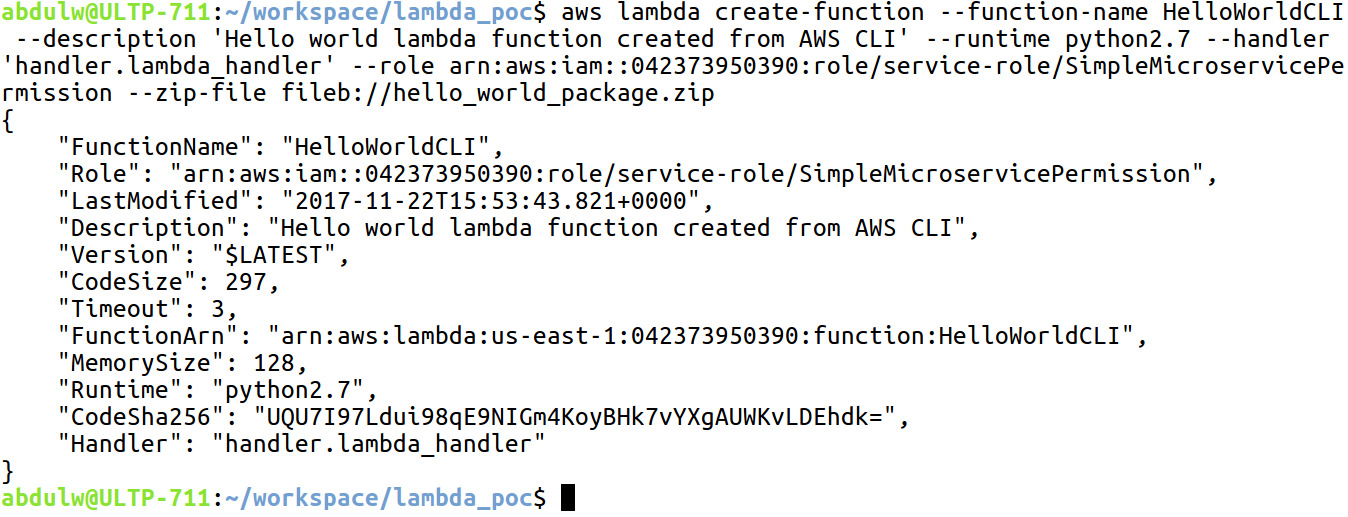
You can see that, in the AWS Lambda console, the Lambda function immediately got created:
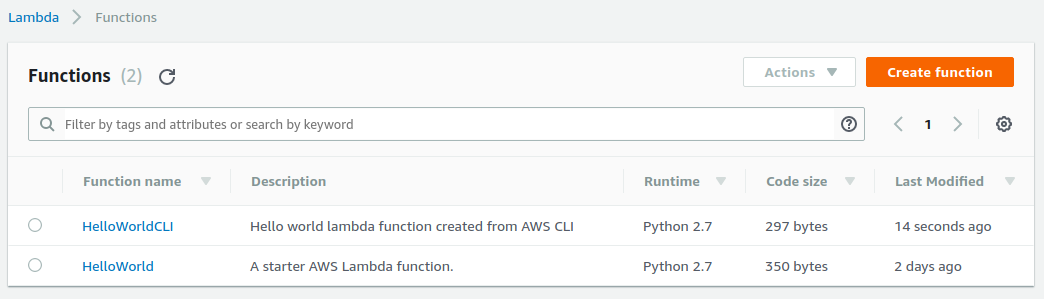
Let's discuss the required and optional parameters that we used with the aws lambda create-function
command:
--function-name
(required): The name is self-explanatory. We need to pass the Lambda function name that we are intending to create.--role
(required): This is a required parameter where we need to use the AWS role ARN as a value. Make sure this role has permissions to create the Lambda function.--runtime
(required): We need to mention the runtime environment for the Lambda function execution. As we mentioned earlier, AWS Lambda supports Python, Node.js, C#, and Java. So these are the possible values:python2.7
python3.6
nodejs
nodejs4.3
nodejs6.10
nodejs4.3-edge
dotnetcore1.0
java8
--handler
(required): Here, we mention the function path that will be an execution entry point by the AWS Lambda. In our case, we usedhandler.lambda_function
, where the handler is the file that contains thelambda_function
.--description
: This option lets you add some text description about your Lambda function.--zip-file
: This option is used to upload the deployment package file of your code from your local environment/machine. Here, you need to addfileb://
as a prefix to your ZIP file path.--code
: This option helps you upload the deployment package file from the AWS S3 bucket.
You should pass a string value with a pattern, such as the one shown here:
"S3Bucket=<bucket-name>,S3Key=<file-name>,S3ObjectVersion=<file-version-id>".
There are many other optional parameters available that you can see with the help
command, such as aws lambda create-function help
. You can use them as per your requirement.
Now we will see the Lambda function invocation using the command $ aws lambda invoke
.
The Lambda CLI provides a command to directly invoke the Lambda function:
$ aws lambda invoke --function-name <value> <outfile>
Let's look at the parameters:
--function-name
(required): This parameter asks for a Lambda function nameoutfile
(required): Here, you need to mention a filename where the returned output or response by the Lambda function will be stored
Here are other optional parameters available that you can list by the help
command.
Let's invoke our recently created HelloWorldCLI
function:
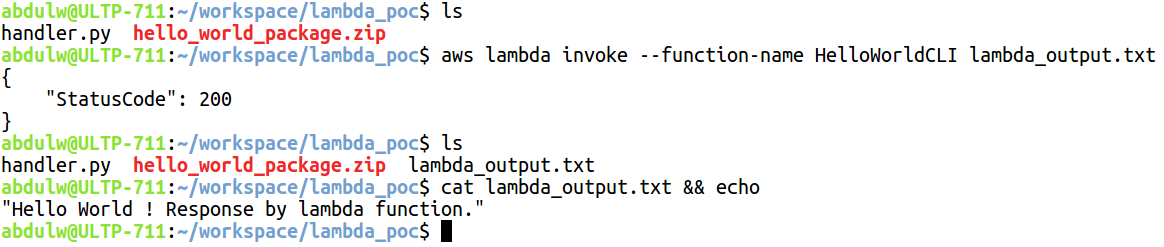
When we invoked the Lambda function, it immediately responded with a status code and the Lambda function returned the output data stored in the newly created lambda_output.txt
file by the lambda invoke
command.
This is a subcommand of the aws lambda
command and is used to create event mapping for your Lambda function. $ aws lambda create-event-source-mapping
supports only Amazon Kinesis stream and Amazon DynamoDB stream events mapping. We will discuss event mapping with the Amazon API Gateway and CloudWatch event using Zappa in the upcoming chapters.