Throughout this book, we will be working with different frameworks, packages, and libraries to create RESTful Web APIs and microservices, and therefore, it is convenient to work with Python virtual environments to isolate each development environment. Python 3.3 introduced lightweight virtual environments and they were improved in subsequent Python versions. We will work with these virtual environments and, therefore, you will need Python 3.7.1 or higher. You can read more about the PEP 405 Python virtual environment, which introduced the venv
module, at https://www.python.org/dev/peps/pep-0405. All the examples for this book were tested on Python 3.7.1 on Linux, macOS, and Windows.
Note
In case you decide to use the popular virtualenv
(https://pypi.python.org/pypi/virtualenv) third-party virtual environment builder or the virtual environment options provided by your Python IDE, you just have to make sure that you activate your virtual environment with the appropriate mechanism whenever it is necessary to do so, instead of following the step explained to activate the virtual environment generated with the venv
module integrated in Python. However, make sure you work with a virtual environment.
Each virtual environment we create with venv
is an isolated environment and it will have its own independent set of installed Python packages in its site directories (folders). When we create a virtual environment with venv
in Python 3.7 or higher, pip
is included in the new virtual environment. Notice that the instructions provided are compatible with Python 3.7.1 or greater. The following commands assume that you have Python 3.7.1 installed on Linux, macOS, or Windows.
First, we have to select the target folder or directory for our lightweight virtual environment. The following is the path we will use in the example for Linux and macOS:
~/HillarPythonREST2/Flask01
The target folder for the virtual environment will be the HillarPythonREST2/Flask01
folder within our home directory. For example, if our home directory in macOS or Linux is /Users/gaston
, the virtual environment will be created within /Users/gaston/HillarPythonREST2/Flask01
. You can replace the specified path with your desired path in each command.
The following is the path we will use in the example for Windows:
%USERPROFILE%\HillarPythonREST2\Flask01
The target folder for the virtual environment will be the HillarPythonREST2\Flask01
folder within our user profile folder. For example, if our user profile folder is C:\Users\gaston
, the virtual environment will be created within C:\Users\gaston\HillarPythonREST2\Flask01
. Of course, you can replace the specified path with your desired path in each command.
In Windows PowerShell, the previous path would be:
$env:userprofile\HillarPythonREST2\Flask01
Now, we have to use the -m
option, followed by the venv
module name and the desired path, to make Python run this module as a script and create a virtual environment in the specified path. The instructions vary depending on the platform in which we are creating the virtual environment. Thus, make sure you follow the instructions for your operating system.
Open a Terminal in Linux or macOS and execute the following command to create a virtual environment:
python3 -m venv ~/HillarPythonREST2/Flask01
In Windows, in the Command Prompt, execute the following command to create a virtual environment:
python -m venv %USERPROFILE%\HillarPythonREST2\Flask01
In case you want to work with Windows PowerShell, execute the following command to create a virtual environment:
python -m venv $env:userprofile\HillarPythonREST2\Flask01
The previous commands don't produce any output. Any of the previous scripts created the specified target folder and installed pip
by invoking ensurepip
because we didn't specify the --without-pip
option.
Now, we will analyze the directory structure for a virtual environment. The specified target folder has a new directory tree that contains Python executable files and other files that indicate it is a PEP 405 virtual environment.
In the root directory for the virtual environment, the pyenv.cfg
configuration file specifies different options for the virtual environment and its existence is an indicator that we are in the root folder for a virtual environment. In Linux and macOS, the folder will have the following main subfolders:
bin
include
lib
lib/python3.7
lib/python3.7/site-packages
The following diagram shows the folders and files in the directory trees generated for the Flask01
virtual environment in macOS:
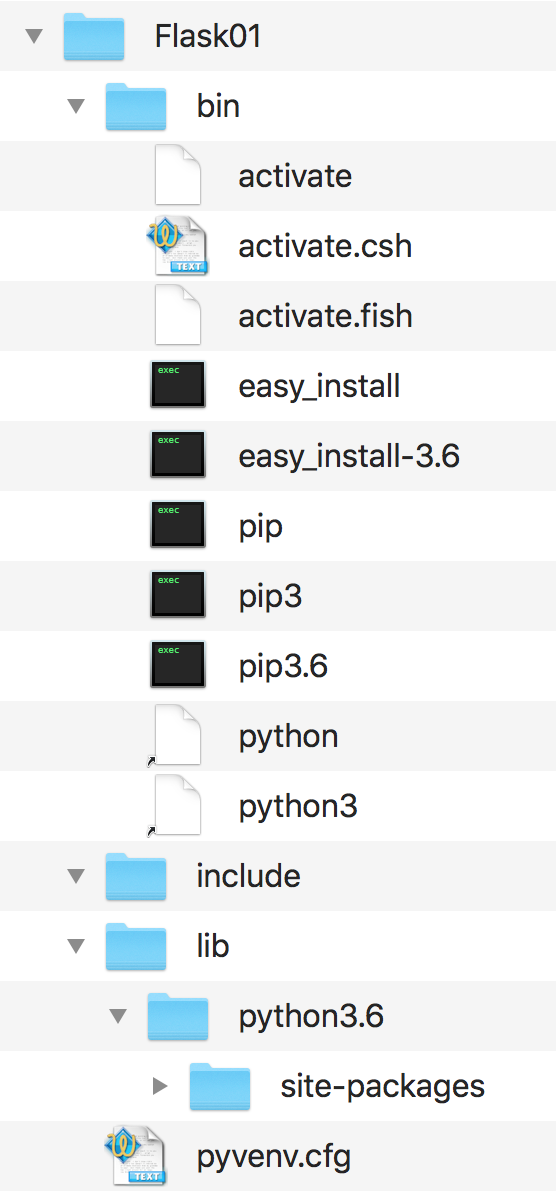
In Windows, the folder will have the following main subfolders:
Include
Lib
Lib\site-packages
Scripts
The directory trees for the virtual environment in each platform are the same as the layout of the Python installation in these platforms.
The following diagram shows the main folders in the directory trees generated for the virtual environment in Windows:
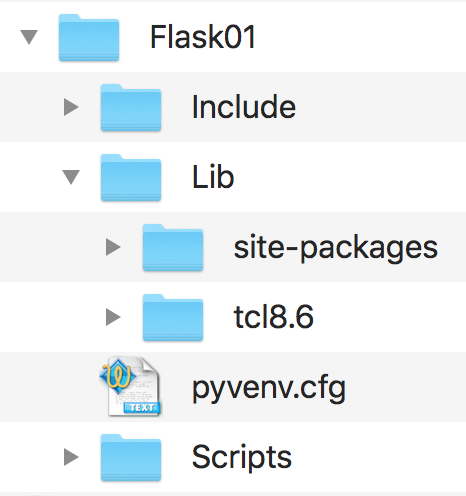
Note
After we activate the virtual environment, we will install third-party packages in the virtual environment and the modules will be located within the lib/python3.7/site-packages
or Lib\site-packages
folder, depending on the platform. The executables will be copied in the bin
or Scripts
folder, based on the platform. The packages we install won't make changes to other virtual environments or our base Python environment.
Now that we have created a virtual environment, we will run a platform-specific script to activate it. After we activate the virtual environment, we will install packages that will only be available in this virtual environment. This way, we will work with an isolated environment in which all the packages we install won't affect our main Python environment.
Run the following command in the Terminal in Linux or macOS. Notice that the results of this command will be accurate if you don't start a shell different to than the default shell in the Terminal session. In case you have doubts, check your Terminal configuration and preferences:
echo $SHELL
The command will display the name of the shell you are using in the Terminal. In macOS, the default is /bin/bash
, and this means you are working with the bash shell. Depending on the shell, you must run a different command to activate the virtual environment in Linux or macOS.
If your Terminal is configured to use the bash
shell in Linux or macOS, run the following command to activate the virtual environment. The command also works for the zsh
shell:
source ~/HillarPythonREST2/Flask01/bin/activate
If your Terminal is configured to use either the csh
or tcsh
shell, run the following command to activate the virtual environment:
source ~/HillarPythonREST2/Flask01/bin/activate.csh
If your Terminal is configured to use the fish
shell, run the following command to activate the virtual environment:
source ~/HillarPythonREST2/Flask01/bin/activate.fish
After you activate the virtual environment, the Command Prompt will display the virtual environment root folder name, enclosed in parentheses as a prefix of the default prompt, to remind us that we are working in the virtual environment. In this case, we will see (Flask01
) as a prefix for the Command Prompt because the root folder for the activated virtual environment is Flask01
.
The following screenshot shows the virtual environment activated in a macOS High Sierra Terminal with a bash
shell, after executing the commands shown previously:

As we can see from the previous screenshot, the prompt changed from Gastons-MacBook-Pro:~ gaston$
to (Flask01) Gastons-MacBook-Pro:~ gaston$
following activation of the virtual environment.
In Windows, you can run either a batch file in the Command Prompt or a Windows PowerShell script to activate the virtual environment.
If you prefer the Command Prompt, run the following command in the Windows command line to activate the virtual environment:
%USERPROFILE%\HillarPythonREST2\Flask01\Scripts\activate.bat
The following screenshot shows the virtual environment activated in a Windows 10 Command Prompt, after executing the commands shown previously:
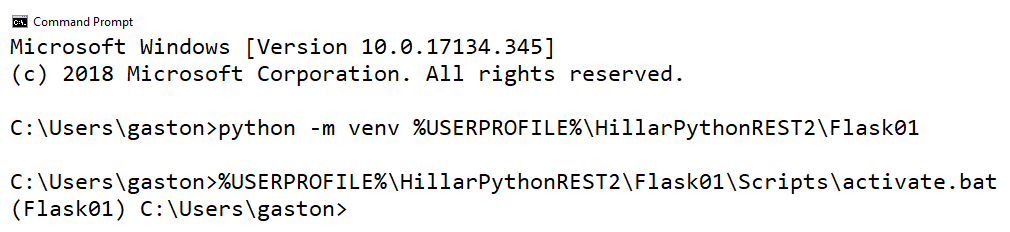
As we can see from the previous screenshot, the prompt changed from C:\Users\gaston\AppData\Local\Programs\Python\Python36
to (Flask01) C:\Users\gaston\AppData\Local\Programs\Python\Python36
following activation of the virtual environment.
If you prefer Windows PowerShell, launch it and run the following commands to activate the virtual environment. Notice that you must have script execution enabled in Windows PowerShell to be able to run the script:
cd $env:USERPROFILEHillarPythonREST2\Flask01\Scripts\Activate.ps1
The following screenshot shows the virtual environment activated in the Windows 10 PowerShell, after executing the commands shown previously:
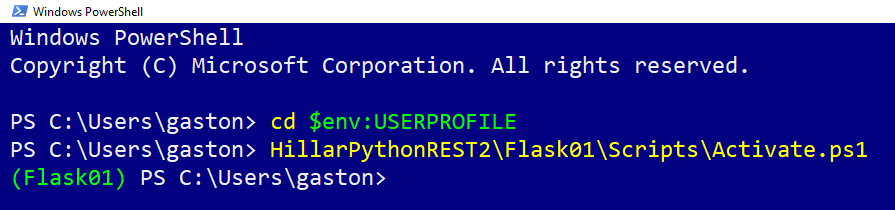
It is extremely easy to deactivate a virtual environment generated by means of the process explained previously. Deactivation will remove all the changes made in the environment variables and will change the prompt back to its default message.
In macOS or Linux, just type deactivate
and press Enter.
In the Windows Command Prompt, you have to run the deactivate.bat
batch file included in the Scripts
folder. In our example, the full path for this file is %USERPROFILE%\HillarPythonREST2\Flask01\Scripts\deactivate.bat
.
In Windows PowerShell, you have to run the Deactivate.ps1
script in the Scripts
folder.