Persisting employee details using Azure Table Storage output bindings
In the previous recipe, you created an HTTP trigger and accepted input parameters. Now, let's learn how to store input data in a persistent medium. Azure Functions supports many ways to store data. For this example, we'll store data in Azure Table storage, a NoSQL key-value persistent medium for storing semi-structured data. Learn more about it at https://azure.microsoft.com/services/storage/tables/.
The primary key of an Azure Table storage table has two parts:
- Partition key: Azure Table storage records are classified and organized into partitions. Each record located in a partition will have the same partition key (p1 in our example).
- Row key: A unique value should be assigned to each row.
Getting ready
This recipe showcases the ease of integrating an HTTP trigger and the Azure Table storage service using output bindings. The Azure HTTP trigger function receives data from multiple sources and stores user profile data in a storage table named tblUserProfile
. We'll follow the prerequisites listed here:
- For this recipe, we'll make use of the HTTP trigger that was created in the previous recipe.
- We'll also be using Azure Storage Explorer, a tool that helps us to work with data stored in an Azure storage account. Download it from http://storageexplorer.com/.
- Learn more about how to connect to a storage account using Azure Storage Explorer at https://docs.microsoft.com/azure/vs-azure-tools-storage-manage-with-storage-explorer.
- Learn more about output bindings at https://docs.microsoft.com/azure/azure-functions/functions-triggers-bindings.
How to do it…
Perform the following steps:
- Navigate to the Integrate tab of the
RegisterUser
HTTP trigger function. - Click on the New Output button, select Azure Table Storage, and then click on the Select button:
Figure 1.10: New output bindings
- If you are prompted to install the bindings, click on Install; this will take a few minutes. Once the bindings are installed, choose the following settings for the Azure Table Storage output bindings:
Table parameter name: This is the name of the parameter that will be used in the
Run
method of the Azure function. For this example, provideobjUserProfileTable
as the value.Table name: A new table in Azure Table storage will be created to persist the data. If the table doesn't exist already, Azure will automatically create one for you! For this example, provide
tblUserProfile
as the table name.Storage account connection: If the Storage account connection string is not displayed, click on new (as shown in Figure 1.11) to create a new one or to choose an existing storage account.
The Azure Table storage output bindings should be as shown in Figure 1.11:
Figure 1.11: Azure Table Storage output bindings settings
- Click on Save to save the changes.
- Navigate to the code editor by clicking on the function name.
Note
The following are the initial lines of the code for this recipe:
#r "Newtonsoft.json"
#r "Microsoft.WindowsAzure.Storage"
The preceding lines of code instruct the function runtime to include a reference to the specified library.
Paste the following code into the editor. The code will accept the input passed by the end user and save it in Table storage; click Save:
#r "Newtonsoft.Json" #r "Microsoft.WindowsAzure.Storage" using System.Net; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Primitives; using Newtonsoft.Json; using Microsoft.WindowsAzure.Storage.Table; public static async Task<IActionResult> Run( HttpRequest req, CloudTable objUserProfileTable, ILogger log) { log.LogInformation("C# HTTP trigger function processed a request."); string firstname=null,lastname = null; string requestBody = await new StreamReader(req.Body).ReadToEndAsync(); dynamic inputJson = JsonConvert.DeserializeObject(requestBody); firstname = firstname ?? inputJson?.firstname; lastname = inputJson?.lastname; UserProfile objUserProfile = new UserProfile(firstname, lastname); TableOperation objTblOperationInsert = TableOperation.Insert(objUserProfile); await objUserProfileTable.ExecuteAsync(objTblOperationInsert); return (lastname + firstname) != null ? (ActionResult)new OkObjectResult($"Hello, {firstname + " " + lastname}") : new BadRequestObjectResult("Please pass a name on the query" + "string or in the request body"); } class UserProfile : TableEntity { public UserProfile(string firstName,string lastName) { this.PartitionKey = "p1"; this.RowKey = Guid.NewGuid().ToString(); this.FirstName = firstName; this. LastName = lastName; } UserProfile() { } public string FirstName { get; set; } public string LastName { get; set; } }
- Execute the function by clicking on the Run button of the Test tab by passing the
firstname
andlastname
parameters to the Request body. - If there are no errors, you'll get a Status: 200 OK message as the output. Navigate to Azure Storage Explorer and view the Table storage to see whether a table named
tblUserProfile
was created successfully:
Figure 1.12: Viewing data in Storage Explorer
How it works…
Azure Functions allows us to easily integrate with other Azure services just by adding an output binding to a trigger. In this example, we have integrated an HTTP trigger with the Azure Table storage binding. We also configured an Azure storage account by providing a storage connection string and the Azure Table storage in which we would like to create a record for each of the HTTP requests received by the HTTP trigger.
We have also added an additional parameter to handle the Table storage, named objUserProfileTable
, of the CloudTable
type, to the Run
method. We can perform all the operations on Azure Table storage using objUserProfileTable
.
Note
The input parameters are not validated in the code sample. However, in a production environment, it's important to validate them before storing them in any kind of persistent medium.
We also created a UserProfile
object and filled it with the values received in the request object, and then passed it to the table operation.
Note
Learn more about handling operations on the Azure Table storage service at https://docs.microsoft.com/azure/cosmos-db/tutorial-develop-table-dotnet.
Understanding storage connections
When you create a new storage connection (refer to step 3 of the How to do it... section of this recipe), a new App settings application will be created:
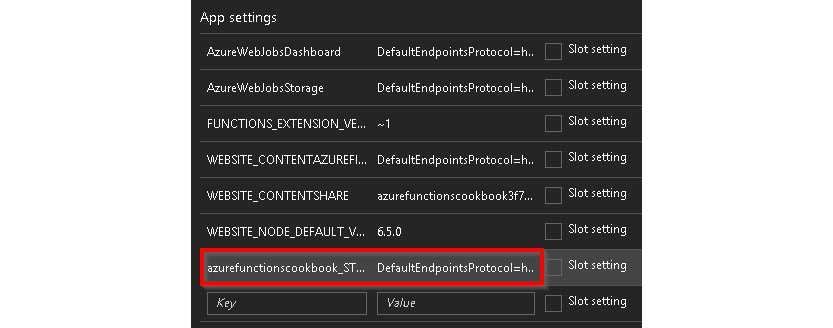
Figure 1.13: Application settings in the configuration blade
Navigate to App settings by clicking on the Configuration menu available in the General Settings section of the Platform features tab:
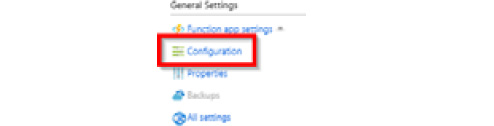
Figure 1.14: Configuration blade
You learned how to save data quickly using Azure Table storage bindings. In the next recipe, you'll learn how to save profile picture paths to queues.