Variables are one of the most widely used containers in PowerShell due to their flexibility. A variable is a container that is used to store a single value or an object. Variables can contain a variety of data types including text (string), numbers (integers), or an object.
If you want to store a string, do the following:
$myString = "My String Has Multiple Words" $myString
The output of this is shown in the following screenshot:

The preceding variable will now contain the words My String Has Multiple Words
. When you output the $myString
variable, as shown in the preceding screenshot, you will see that the string doesn't contain the quotations. This is because the quotations tell the PowerShell command-line interpreter to store the value that is between the two positions or quotations.
Tip
You are able to reuse variables without deleting the content already inside the variable. The PowerShell interpreter will automatically overwrite the data for you.
Subsequently, if you want to store a number, do the following:
$myNumber = 1 $myNumber
The output of this is shown in the following screenshot:
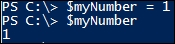
This method differentiates while storing a string as you do not use quotations. This will tell the PowerShell interpreter to always interpret the value as a number. It is important to not use quotations while using a number, as you can have errors in your script if the PowerShell interpreter mistakes a number for a string.
An example of what happens when you use strings instead of integers can be seen here:
$a = "1" $b = "2" $c = $a + $b $c
The output of this is shown in the following screenshot:
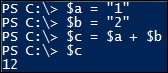
The $c
variable will contain the value of 12
. This is due to PowerShell interpreting your $a
string of 1
and $b
string of 2
and putting the characters together to make 12
.
The correct method to do the math would look like this:
$a = 1 $b = 2 $c = $a + $b $c
The output of this is shown in the following screenshot:
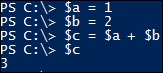
Since the $a
and $b
variables are stored as numbers, PowerShell will perform the math on the numbers appropriately. The $c
variable will contain the correct value of 3
.
Objects are vastly different than strings and numbers. Objects in PowerShell are data structures that contain different attributes such as properties and methods with which one can interact. Object properties are descriptors that typically contain data about that object or other related objects. Object methods are typically sections of code that allow you to interact with that object or other objects on a system. These objects can easily be placed in variables. You can simply place an object in a variable by declaring a variable and placing an object in it. To view all of the object's attributes, you can simply call the variable containing the object, use a pipe character |
, and use the get-member
cmdlet.
To place an object in a variable and retrieve its attributes, you need to do this:
$date = get-date $date $date | get-member
The output is shown in the following screenshot:
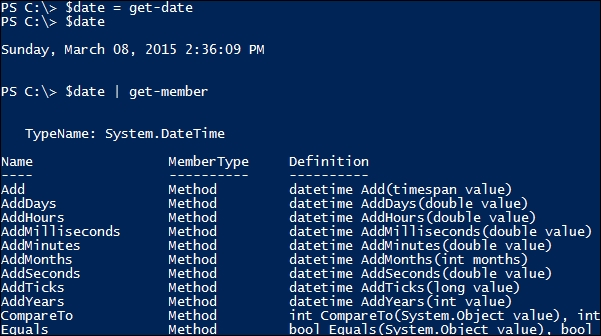
In this example, you will learn how to place an object into a variable. You first start by declaring the $date
variable and setting it equal to the output from the get-date
cmdlet. When you execute this, the get-date
cmdlet references the System.Date
class, and the $date
variable inherits all of that object's attributes. You then call the $date
variable and you see that the output is the date and time from when that command was run. In this instance, it is displaying the DateTime
ScriptProperty
attribute on the screen. To view all of the attributes of the System.Date
object in the $date
variable, you pipe those results to the get-member
cmdlet. You will see all of the attributes of that object displayed on the screen.
If you want to use the properties and method attributes of that object, you can simply call them using dot notation. This is done by calling the variable, followed by a period, and referencing the property or method.
To reference an object's properties and method attributes, you need to do this:
$date = get-date $date.Year $date.addyears("5")
The output of this is shown in the following screenshot:
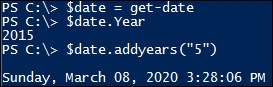
This example shows you how to reference an object's properties and method attributes using dot notation. You first start by declaring the $date
variable and setting it equal to the output from the get-date
cmdlet. When you execute this, the get-date
cmdlet references the System.Date
class, and the $date
variable inherits all of that object's attributes. You then leverage dot notation to reference the Year
property attribute by calling $date.Year
. The attribute will return 2015
as the Year
property. You then leverage dot notation to use the AddYears()
method to increase the years by 5
. After entering the $date.addyears("5")
command, you will see an output on the screen of the same month, day, and time; however, the year is incremented by 5
years.