Scripts are simply files with a .ps1
file extension, which contain PowerShell code. It is possible to parameterize a script file in the same way that you would a function using a Param()
statement at the beginning of the file. If we were to store the following code in a file called Get-PowerShellVersionMessage.ps1
, it would be roughly equivalent to the Get-PowerShellVersionMessage
function in the previous section:
param($name) $PowerShellVersion=$PSVersionTable.PSVersion return "We're using $PowerShellVersion, $name!"
A security feature of PowerShell is that it won't run a script in the current directory without specifically referring to the directory, so calling this script would look like this:
.\get-powershellversionmessage –name Mike
The following screenshot shows the aforementioned code being stored in a file:
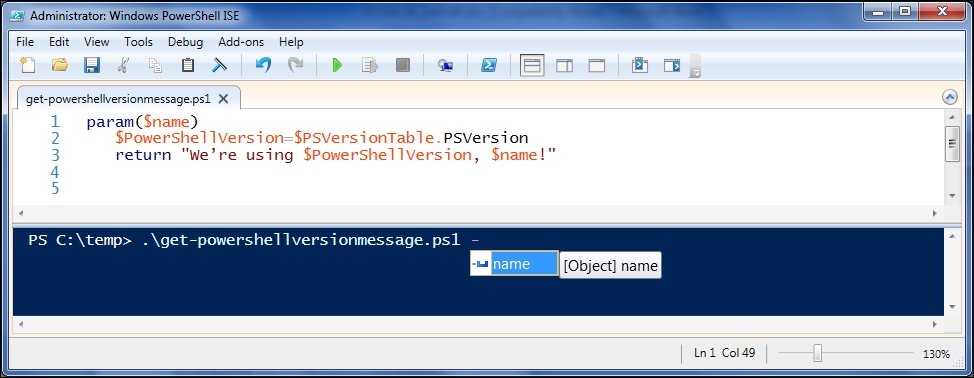
And the output would be (on the computer I'm using): We're using PowerShell 4.0, Mike.
Note
Depending on your environment, you might not be able to run scripts until you change the execution policy. The execution policy dictates whether scripts are allowed to be executed, where those scripts can be located, and whether they need to be digitally signed. Typically, I use set-executionpolicy RemoteSigned
to allow local scripts without requiring signatures. For more information about execution policies, refer to about_execution_policies
.
It is also possible to define multiple functions in a script. However, when doing so, it is important to understand the concept of scope. When a script or function is executed, PowerShell creates a new memory area for definitions (for example, variables and functions) that are created during the execution. When the script or function exits, that memory is destroyed, thereby removing the new definitions. Executing a script with multiple functions will not export those functions into the current scope. Instead, the script executes in its own scope and defines the functions in that scope. When the script execution is finished, the newly created scope is exited, removing the function definitions. To overcome this situation, the dot-source operator was created. To dot-source a file means to run the file, without creating a new scope in which to run. If there was a script file with function definitions called myFuncs.ps1
, dot-sourcing the file would use the following syntax:
. .\myFuncs.ps1
Note that there is a space after the first dot, and that since the script is in the current directory explicit use of the directory is required.