Google Cloud Functions is the serverless compute service that runs our code in response to events. The resources needed to run the code are automatically managed and scaled. At the time of writing this recipe, Google Cloud Functions is in beta. The functions can be written in JavaScript on a Node.js runtime. The functions can be invoked with an HTTP trigger, file events on Cloud Storage buckets, and messages on Cloud Pub/Sub topic.
We'll create a simple calculator using an HTTP trigger that will take the input parameters via the HTTP POST method and provide the result.
The following are the initial setup verification steps to be taken before the recipe can be executed:
- Create or select a GCP project
- Enable billing and enable the default APIs (some APIs such as BigQuery, storage, monitoring, and a few others are enabled automatically)
- Verify that Google Cloud SDK is installed on your development machine
- Verify that the default project is set properly
We'll use the simple calculator JavaScript code available on the book's GitHub repository and deploy it to Cloud Functions:
- Navigate to the
/Chapter01/calculator
folder. The application code is present inindex.js
and the dependencies in thepackage.json
file. As there are no dependencies for this function, thepackage.json
file is a basic skeleton needed for the deployment. - The
main
function receives the input via the request object, validates the inputs and performs the calculation. The calculated result is then sent back to the requester via the response object and an appropriate HTTP status code. In the following code, theswitch
statement does the core processing of the calculator, do spend some time on it to understand the gist of this function:
/** * Responds to any HTTP request that provides the below JSON message in the body. * # Example input JSON : {"number1": 1, "operand": "mul", "number2": 2 } * @param {!Object} req Cloud Function request context. * @param {!Object} res Cloud Function response context. */ exports.calculator = function calculator(req, res) { if (req.body.operand === undefined) { res.status(400).send('No operand defined!'); } else { // Everything is okay console.log("Received number1",req.body.number1); console.log("Received operand",req.body.operand); console.log("Received number2",req.body.number2); var error, result; if (isNaN(req.body.number1) || isNaN(req.body.number2)) { console.error("Invalid Numbers"); // different logging error = "Invalid Numbers!"; res.status(400).send(error); } switch(req.body.operand) { case "+": case "add": result = req.body.number1 + req.body.number2; break; case "-": case "sub": result = req.body.number1 - req.body.number2; break; case "*": case "mul": result = req.body.number1 * req.body.number2; break; case "/": case "div": if(req.body.number2 === 0){ console.error("The divisor cannot be 0"); error = "The divisor cannot be 0"; res.status(400).send(error); } else{ result = req.body.number1/req.body.number2; } break; default: res.status(400).send("Invalid operand"); break; } console.log("The Result is: " + result); res.status(200).send('The result is: ' + result); } };
- We'll deploy the calculator function using the following command:
$ gcloud beta functions deploy calculator --trigger-http
The entry point for the function will be automatically taken as the calculator function. If you choose to use another name, index.js
, the deploy
command should be updated appropriately:
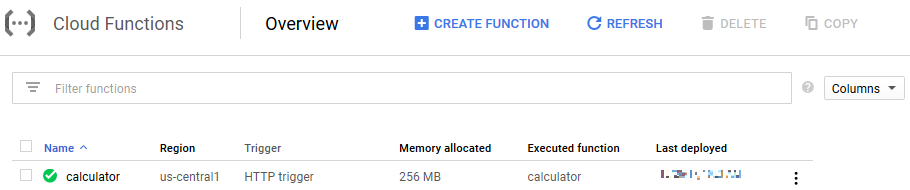
Input JSON : {"number1": 1, "operand": "mul", "number2": 2 } $ curl -X POST https://us-central1-<ProjectID>.cloudfunctions.net/calculator -d '{"number1": 1, "operand": "mul", "number2": 2 }' -H "Content-Type: application/json" The result is: 2
- You can also click on the
VIEW LOGS
button in the Cloud Functions interface to view the logs of the function execution:
