In this recipe, you will learn how to detect objects using colors in the HSV color space using OpenCV-Python. You need to specify a range of color values by means of which the object you are interested in will be identified and extracted. You can change the color of the object detected and even make the detected object transparent.
Object detection using color in HSV
Getting ready
In this recipe, the input image we will use will be an orange fish in an aquarium and the object of interest will be the fish. You will detect the fish, change its color, and make it transparent using the color range of the fish in HSV space. Let's start by importing the required libraries:
import cv2
import numpy as np
import matplotlib.pylab as plt
How to do it...
To do the recipe, the following steps need to be performed:
- Read the input and background image. Convert the input image from BGR into the HSV color space:
bck = cv2.imread("images/fish_bg.png")
img = cv2.imread("images/fish.png")
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
- Create a mask for the fish by selecting a possible range of HSV colors that the fish can have:
mask = cv2.inRange(hsv, (5, 75, 25), (25, 255, 255))
- Slice the orange fish using the mask:
imask = mask>0
orange = np.zeros_like(img, np.uint8)
orange[imask] = img[imask]
- Change the color of the orange fish to yellow by changing the hue channel value only (add 20) and converting the image back into the BGR space:
yellow = img.copy()
hsv[...,0] = hsv[...,0] + 20
yellow[imask] = cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)[imask]
yellow = np.clip(yellow, 0, 255)
- Finally, create the transparent fish image by first extracting the background without the input image with the fish, and then extracting the area corresponding to the foreground object (fish) from the background image and adding these two images:
bckfish = cv2.bitwise_and(bck, bck, mask=imask.astype(np.uint8))
nofish = img.copy()
nofish = cv2.bitwise_and(nofish, nofish, mask=(np.bitwise_not(imask)).astype(np.uint8))
nofish = nofish + bckfish
How it works...
The following screenshot shows an HSV colormap for fast color lookup. The x axis denotes hue, with values in (0,180), the y axis (1) denotes saturation with values in (0,255), and the y axis (2) corresponds to the hue values corresponding to S = 255 and V = 255. To locate a particular color in the colormap, just look up the corresponding H and S range, and then set the range of V as (25, 255). For example, the orange color of the fish we are interested in can be searched in the HSV range from (5, 75, 25) to (25, 255, 255), as observed here:
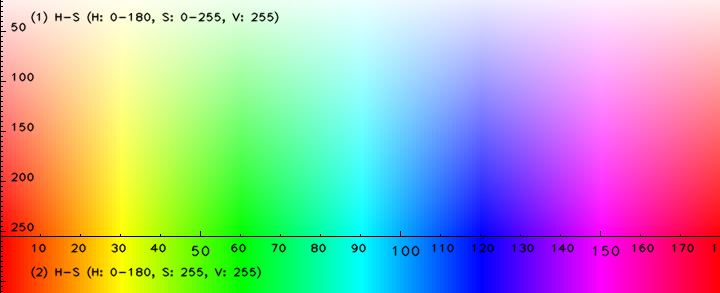
The inRange() function from OpenCV-Python was used for color detection. It accepts the HSV input image along with the color range (defined previously) as parameters.
cv2.inRange() accepts three parameters—the input image, and the lower and upper limits of the color to be detected, respectively. It returns a binary mask, where white pixels represent the pixels within the range and black pixels represent the one outside the range specified.
To change the color of the fish detected, it is sufficient to change the hue (color) channel value only; we don't need to touch the saturation and value channels.
The bitwise arithmetic with OpenCV-Python was used to extract the foreground/background.
Notice that the background image has slightly different colors from the fish image's background; otherwise, transparent fish would have literally disappeared (invisible cloaking!).
If you run the preceding code snippets and plot all of the images, you will get the following output:
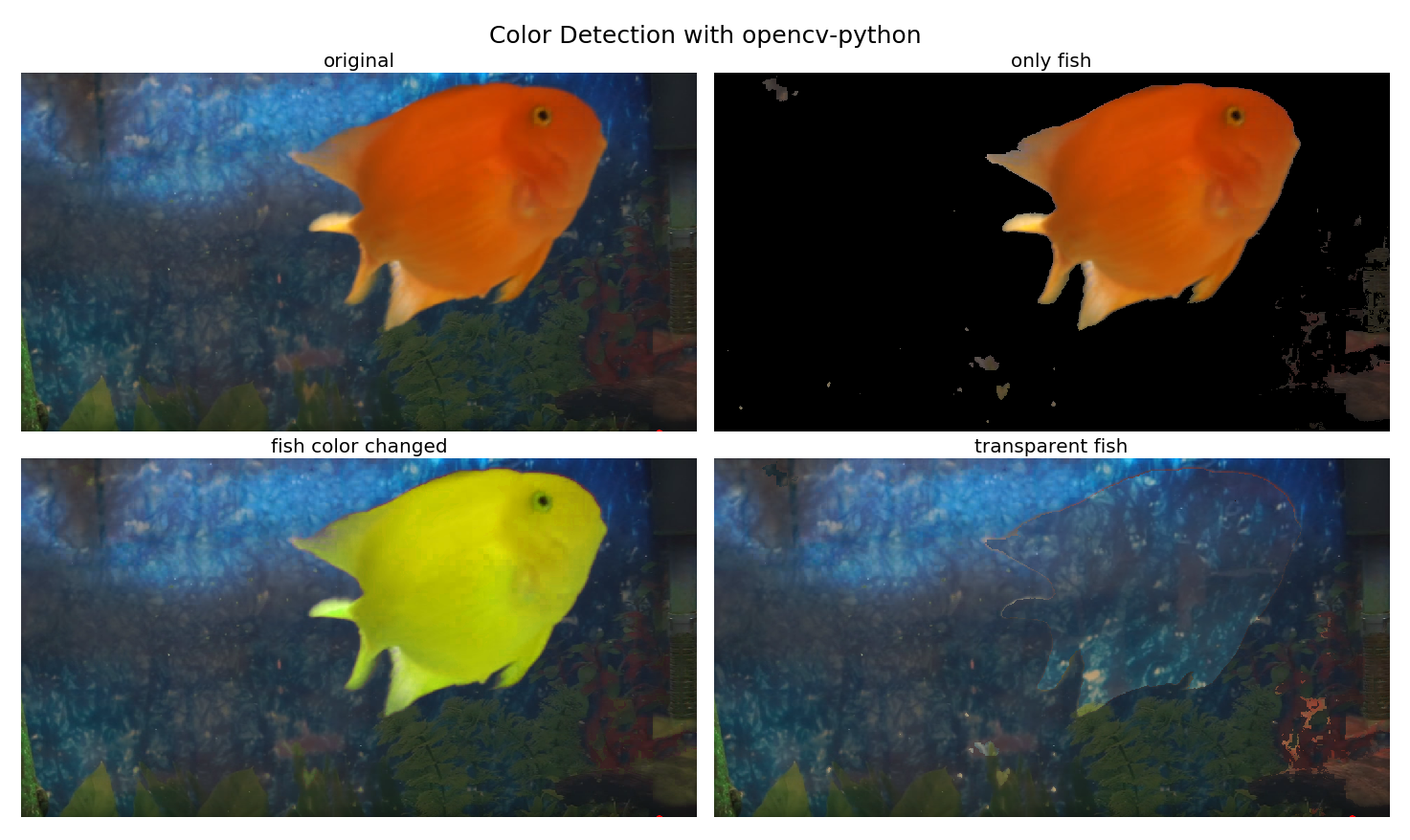
Note that, in OpenCV-Python, an image in the RGB color space is stored in BGR format. If we want to display the image in proper colors, before using imshow() from Matplotlib (which expects the image in RGB format instead), we must convert the image colors with cv2.cvtColor(image, cv2.COLOR_BGR2RGB).
See also
For more details, refer to the following links:
- https://opencv-python-tutroals.readthedocs.io/en/latest/py_tutorials/py_imgproc/py_colorspaces/py_colorspaces.html
- https://i.stack.imgur.com/gyuw4.png
- https://stackoverflow.com/questions/10948589/choosing-the-correct-upper-and-lower-hsv-boundaries-for-color-detection-withcv
- https://www.youtube.com/watch?v=lF0aOM3WJ74