In the Working with arrays recipe, you've learned how to deal with arrays, but working with an array has its limitations. You can't easily add or remove objects. The ArrayList data structure works in a way similar to a regular array of objects, but here you can add or remove objects in a very easy way.
You can start by saving your sketch as working_with_arraylists.pde
. The next thing you need to do is to add a new tab to your sketch and save it as MyObject
. You can do this by clicking the arrow icon on top of the PDE or by using the shortcut Shift + Cmd + N on the Mac, or Shift + Ctrl + N on Windows or Linux.
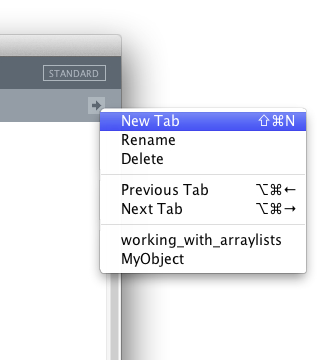
In this tab, we'll write a simple class with two methods. We'll use this class to create objects to fill our ArrayList
.
class MyObject { float x; float y; MyObject() { x = random( width ); y = random( height ); } void update() { y--; } void render() { ellipse( x, y, 60, 60 ); } }
Once you've written...