Windows Forms are a lightweight alternative to MFC, and in this recipe, we'll show you how to create a Windows Forms application that uses Ogre to render a 3D robot model.
To follow along with this recipe, open the solution located in the Recipes/Chapter01/OgreInWinForms
folder in the code bundle available on the Packt website.
First, we'll create a new Windows Forms application using the New Project wizard.
1. Create a new project by clicking File | New | Project. In the Project Types pane, expand Visual C++, select CLR, then select Windows Forms Application in the Templates pane. Name the project
OgreInWinForms
. For Location, browse to yourRecipes
folder, append\Chapter_01_Examples
, and click on OK.The Windows Forms Designer will appear showing Form1 of the Windows Forms application that we just created.
2. Next, in the Solution Explorer pane, right-click
Form1.h
, and click View Code.In
Form1.h
, add a newCEngine
member instance variable.public: CEngine *m_Engine;
3. In the constructor, create an instance of our
CEngine
class, and pass it our window handle.OgreForm(void) : m_Engine(NULL){ InitializeComponent(); m_Engine = new CEngine((HWND)this->Handle.ToPointer()); }
4. Next, we add a
PaintEventHandler
function andResize EventHandler
function to theInitializeComponent()
method, and set the default window state tomaximized
.this->WindowState = System::Windows::Forms::FormWindowState::Maximized; this->Paint += gcnew System::Windows::Forms::PaintEventHandler(this, &OgreForm::Ogre_Paint); this->Resize += gcnew System::EventHandler(this, &OgreForm::OgreForm_Resize);
5. Create the functions for the event handlers we just added.
private: System::Void Ogre_Paint(System::Object^ sender, System::Windows::Forms::PaintEventArgs^ e) { m_Engine->m_Root->renderOneFrame(); } private: System::Void OgreForm_Resize(System::Object^ sender, System::EventArgs^ e) { if (m_Engine != NULL) { m_Engine->m_Root->renderOneFrame(); } }
In the Ogre_Paint()
and OgreForm_Resize()
methods, we call renderOneFrame()
, instructing Ogre to render to our form surface.
Windows Forms is a smart client technology for the .NET framework, a set of managed libraries that simplify common application tasks. In Windows Forms, a form is a visual surface on which you display information to the user. You ordinarily build Windows Forms applications by adding controls to forms and those controls respond to user actions, such as mouse clicks or key presses. A control is a discrete User Interface (UI) element that displays data or accepts data input. In this basic Windows Forms application, we have Ogre draw the contents of our 3D scene on a form surface.
The following is a screenshot of our Ogre Windows Forms application in action:
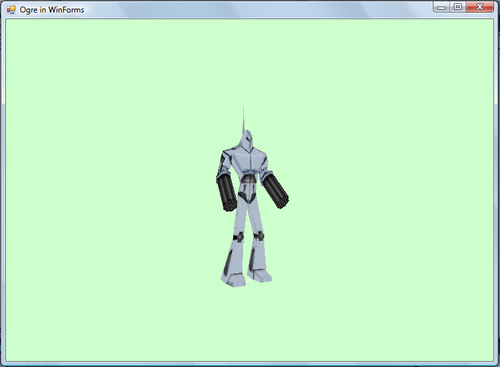
It's easy to add controls to our form. In the Toolbox, click on the control you want to add. Then, on the form, click where you want the upper-left corner of the control to be located, and drag to where you want the lower-right corner of the control to be. When you let go, the control will be added to the form.
You can also add a control to the form, programmatically, at runtime.