Loops in PowerShell execute a series of commands or cmdlets as long as the condition to run them is true. Loops are helpful for running repetitive tasks inside a PowerShell script. For example, if we need to create five new users, we can use a loop, and inside the loop, we can add the logic to create a new user and execute the loop five times. Loops allow us to write business logic once and then run it repetitively as long as a certain condition is met. To implement loops in PowerShell, we can use the for
, foreach
, while
, do...while
, and do...until
loops.
In a for
loop, we run the command block based on a conditional test. In the following for
loop, we are running Write-Host
until the value of variable $i
is less than 5
. In the beginning, the value of variable $i
is 0
, and every time the loop is executed, we are incrementing the value of $i
by 1
. During the execution of the loop, when the value of variable $i
becomes 5
, the loop stops executing:
for ( $i=0; $i -lt 5; $i++) { Write-Host "Value of i is" $i }
The output of this for
loop is as follows:
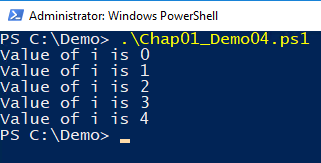
Using the while
, do...while
, and do...until
loops, we can run loops as long as a condition is true
(it is met).
The while
loops only use the while
keyword, followed by the condition and then the script block, as shown here:
$i=1 while ($i -le 10) { Write-Host "Value of i is" $i $i++ }
In this script, the script block inside the while
loop will run till the value of the variable $i
is less than 10
. The output of this while
loop is as follows:
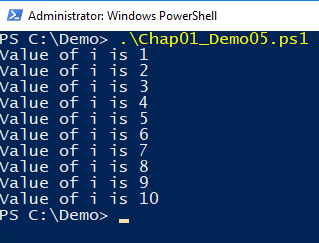
The do...while
and do...until
loops begin with the do
keyword, followed by the script block and then by the conditional keyword and the condition.
Here's an example of the do...while
loop:
$i=1 do { Write-Host "Value of i is" $i $i++ } while ($i -le 10)
Here's an example of the do...until
loop:
$i=1 do { Write-Host "Value of i is" $i $i++ } until ($i -gt 10)
Both the examples mentioned here basically implement the same business logic using loops, with slightly different comparison methods. In the do...while
loop, the script block will run until the value of the variable $i
is less than 10
, and in the do...until
loop, the script block will run until the value of the variable $i
becomes greater than 10
. The output of both the loops will be the same as, shown here:
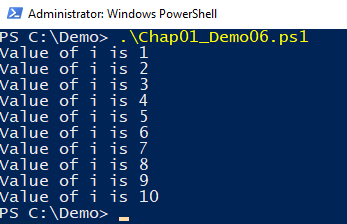