Software development is not just writing code. We also need to test it, to confirm that it does what is expected. There are several kinds of tests, and unit tests are one of the most popular. In this chapter, we will set up the unit test framework that will accompany us throughout the book. Another important concept is that of mocking; by mocking a class (or interface), we can provide a dummy implementation of it that we can use instead of the real thing. This comes in handy in unit tests, because we do not always have access to real-life data and environments, and this way, we can pretend we do.
We will be using the NuGet Package Manager to install the Entity Framework Core 1 package, Microsoft.EntityFrameworkCore
. We will be using a SQL Server database for storing the data, so we will also need Microsoft.EntityFrameworkCore.SqlServer
.
To mock interfaces and base classes, we will use Moq
.
Finally, xunit
is the package we will be using for the unit tests and dotnet-text-xunit
adds tooling support for Visual Studio. Note that the UnitTests
project is a .NET Core App 1.0 (netcoreapp1.0), that Microsoft.EntityFrameworkCore.Design
is configured as a build dependency, and Microsoft.EntityFrameworkCore.Tools
is set as a tool.
Open Using EF Core Solution from the included source code examples.
Execute the database setup script from the code samples included for this recipe. This can be found in the DataAccess
project within the Database
folder.
Start by adding the required NuGet packages to the
UnitTests
project. We'll edit and add two dependencies, the mainxUnit
library and its runner for .NET Core, and then set therunner
command.Now, let's add a base class to the project; create a new C# class file and call it
BaseTests.cs
:using Microsoft.Extensions.Configuration; namespace UnitTests { public abstract class BaseTest { protected BaseTest() { var builder = new ConfigurationBuilder() .AddJsonFile("appsettings.json"); Configuration = builder.Build(); } protected IConfiguration Configuration{ get; private set; } } }
Now, for a quick test, add a new C# file, called
SimpleTest.cs
, to the project, with this content:using Moq; using Xunit; namespace UnitTests { public class SimpleTest : BaseTest { [Fact] public void CanReadFromConfiguration() { var connectionString = Configuration["Data:Blog:ConnectionString"]; Assert.NotNull(connectionString); Assert.NotEmpty(connectionString); } [Fact] public void CanMock() { var mock = new Mock<IConfiguration>(); mock.Setup(x => x[It.IsNotNull<string>()]).Returns("Dummy Value"); var configuration = mock.Object; var value = configuration["Dummy Key"]; Assert.NotNull(value); Assert.NotEmpty(value); } } }
If you want to have the xUnit runner running your unit tests automatically, you will need to set the test command as the profile to run in the project properties:
Project properties
We have a unit tests base class that loads configuration from an external file, in pretty much the same way as the ASP.NET Core template does. Any unit tests that we will define later on should inherit from this one.
When the runner executes, it will discover all unit tests in the project—those public concrete methods marked with the [Fact]
attribute. It will then try to execute them and evaluate any Assert calls within.
The Moq framework lets you define your own implementations for any abstract or interface methods that you wish to make testable. In this example, we are mocking the IConfiguration
class, and saying that any attempt to retrieve a configuration value should have a dummy value as the result.
If you run this project, you will get the following output:
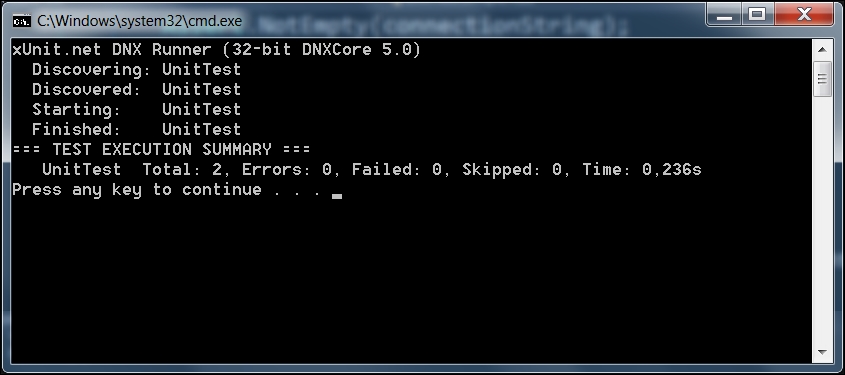
Running unit tests
Testing to the edges of an application requires that we adhere to certain practices that allow us to shrink the untestable sections of the code. This will allow us to unit test more code, and make our integration tests far more specific.
An important point to remember while performing unit testing is that we should only be testing a single class. The point of a unit test is to ensure that a single operation of this class performs the way we expect it to.
This is why simulating classes that are not under test is so important. We do not want the behavior of these supporting classes to affect the outcomes of unit tests for the class that is being tested.
Often, it is equally important to test the actual combination of your various classes to ensure they work properly together. These integration tests are valuable, but are almost always more brittle, require more setup, and are run slower than the unit tests. We certainly need integration tests on any project of a reasonable size, but we want unit tests first.
Most unit tests can be viewed as having three parts: Arrange, Act, and Assert. Arrange is where we prepare the environment to perform the test, for instance, mocking the IDBContext
with dummy data with the expectation that Set will be called. Act is where we perform the action under test, and is most often a singular line of code. Assert is where we ensure that the proper result was reached. Note the comments in the preceding examples that call out these sections. We will use them throughout the book to make it clear what the test is trying to do.
Mocking and stubbing—providing a pre-built implementation for methods to intercept—is a very interesting topic. There are numberless frameworks that can provide mocking capabilities for even the most challenging scenarios, such as static methods and properties. Mocking fits nicely with unit tests because we seldom have an environment that is identical to the one where we will be deploying, but we don't have "real" data. Also, data changes, and we need a way to be able to reproduce things consistently.