The following code shows how we could implement the reentrant lock ourselves. The code shows the reentrancy semantics, too. We just show the lock and unlock method:
public class YetAnotherReentrantLock { private Thread lockedBy = null; private int lockCount = 0;
The class has two fields—a lockedBy
thread reference and a lockcount
—which are used to track how many times the thread recursively locked itself:
private boolean isLocked() { return lockedBy != null; } private boolean isLockedByMe() { return Thread.currentThread() == lockedBy; }
The preceding snippet shows two helper methods. Using such helpers help make the code more readable:
public synchronized void lock() throws InterruptedException { while (isLocked() && !isLockedByMe()) { this.wait(); } lockedBy = Thread.currentThread(); lockCount++; }
Here is a pictorial analysis of the lock()
method:
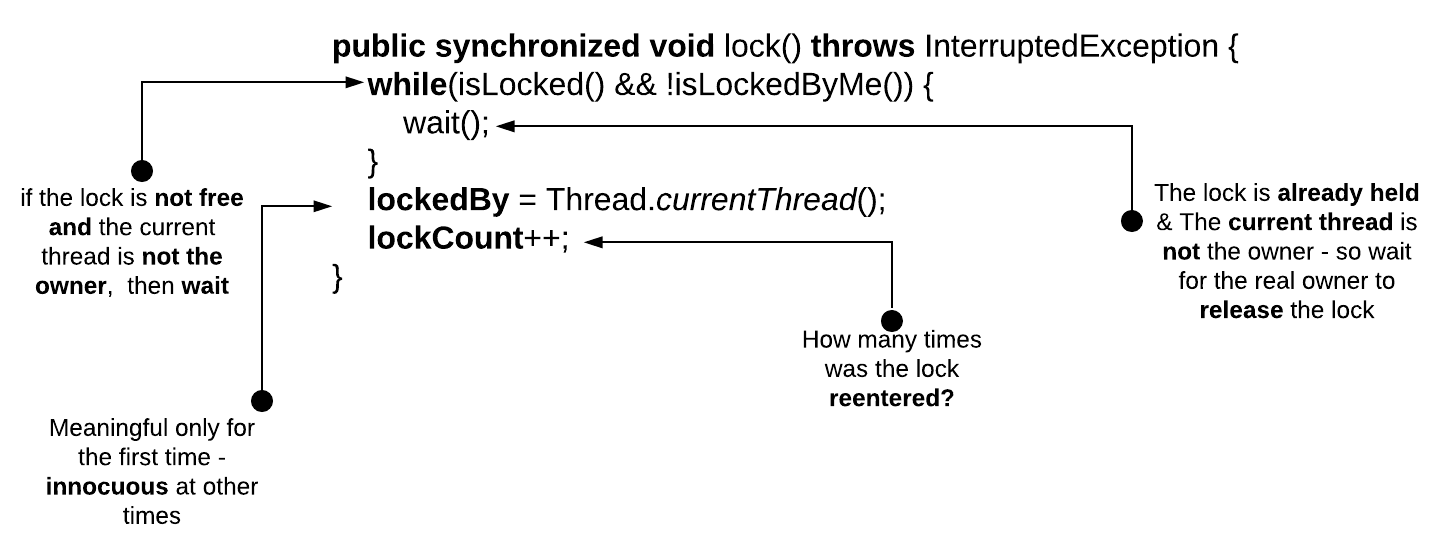
Keep in mind the lock semantics—the lock is granted if and only if it's in a released state! Otherwise, some other...