A blockchain is essentially a decentralized distributed database or a ledger, as follows:
- Decentralization: In simple terms, it means that the application or service continues to be available and usable even if a server or a group of servers on a network crashes or is not available. The service or application is deployed on a network in a way that no server has absolute control over data and execution, rather each server has a current copy of data and execution logic.
- Distributed: This means that any server or node on a network is connected to every other node on the network. Rather than having one-to-one or one-to-many connectivity between servers, servers have many-to-many connections with other servers.
- Database: This refers to the location for storing durable data that can be accessed at any point in time. A database allows storage and retrieval of data as functionality and also provides management functionalities to manage data efficiently, such as export, import, backup, and restoration.
- Ledger: This is an accounting term. Think of it as specialized storage and retrieval of data. Think of ledgers that are available to banks. For example, when a transaction is executed with a bank—say, Tom deposits 100 dollars in his account, the bank enters this information in a ledger as a credit. At some point in the future Tom withdraws 25 dollars. The bank does not modify the existing entry and stored data from 100 to 75. Instead it adds another entry in the same ledger as a debit of 25 dollars. It means a ledger is a specialized database that does not allow modification of existing data. It allows you to create and append a new transaction to modify the current balance in the ledger. The blockchain is a database that has the same characteristics of a ledger. It allows newer transactions to be stored in an append-only pattern without any scope to modify past transactions. It is important here to understand that existing data can be modified by using a new transaction, but past transactions cannot be modified. A balance of 100 dollars can be modified at any time by executing a new debit or credit transaction, but previous transactions cannot be modified. Take a look at the following diagram for a better understanding:
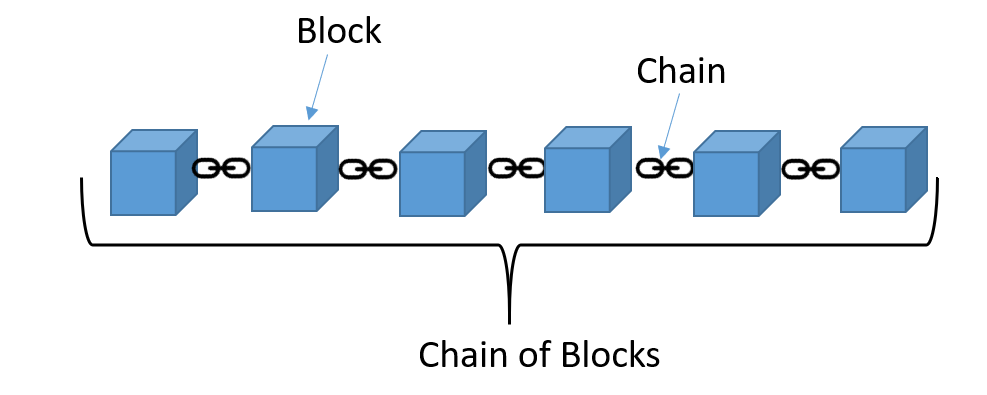
Blockchain means a chain of blocks. Blockchain means having multiple blocks chained together, with each block storing transactions in a way where it is not possible to change these transactions. We will discuss this in later sections when we talk about the storage of transactions and how immutability is achieved in a blockchain.
Because of being decentralized and distributed, blockchain solutions are stable, robust, durable, and highly available. There is no single point of failure. No single node or server is the owner of the data and solution, and everyone participates as a stakeholder.
Not being able to change and modify past transactions makes blockchain solutions highly trustworthy, transparent, and incorruptible.
Ethereum allows extending its functionality with the help of smart contracts. Smart contracts will be addressed in detail throughout this book.