When we start to build microservice architecture, we try to involve different services to deliver business solutions. We often build services as traditional API models, where each of the services can interact with other services. This is referred to as distributed architecture. If a distributed architecture is designed incorrectly, performance issues surface very quickly. It can be difficult to have numerous distributed services that work concurrently to deliver the intended performance. Companies that offer services requiring large data exchange (such as Netflix, Amazon, or Lightbend) have therefore seen a need for alternative paradigms, which can be used for systems with the following characteristics:
- Consisting of very loosely coupled components
- Responding to user inputs
- Resilient to varying load conditions
- Always available
In order to achieve the preceding characteristics, we need to build event-driven, modular services that communicate with each other by using notifications. In turn, we can respond to the system's flow of events. The modular services are more scalable, as we can add or remove service instances without halting the complete application. The complete architecture will be fault tolerant if we can isolate errors and take corrective actions. The preceding four characteristics are the basic principles of the Reactive Manifesto. The Reactive Manifesto states that each reactive system should consist of loosely coupled components that rely on asynchronous, message-driven architecture. They must remain responsive to user input and isolate failures to individual components. Replication must be done in order to respond to varying load conditions. The following is a diagram of the Reactive Manifesto:
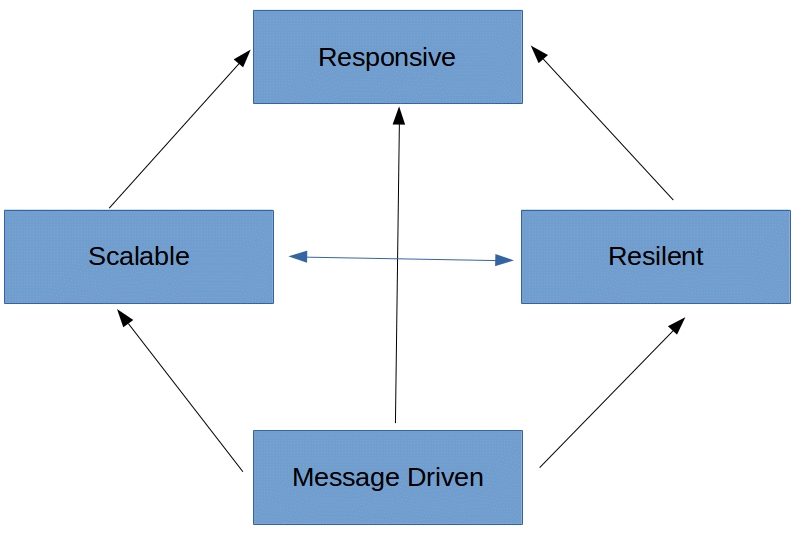
The Reactive Manifesto describes a reactive system. It does not required that the system be based on reactive programming, or any other reactive library. We can build a message-driven, resilient, scalable, and responsive application without using a reactive library, but it is easier to build an application based on reactive libraries.