If you are familiar with the build tool landscape, you might be in one of three camps—Maven, Gradle, or SBT (more likely if you are a Scala developer). I am not going to go into the details, but we will present the basics of Gradle, the modern open source polyglot build automation system, and leave it up to the curious to find out more from http://gradle.org. Before we continue, please make sure you have it installed and available in your classpath in order for it to be accessible from the Terminal. If you have SDKMAN, you can install it using this command:
$ sdk install gradle 3.0
The build system comes with some baked-in templates, albeit limited ones, and, in its latest 3.0 version, Kotlin is not yet included. Hopefully, this shortfall will be dealt with sooner rather than later; however, it takes very little effort to configure support for it. First, let's see how you can list the available templates by executing the following command:
$ gradle help --task :init
You should see the following being printed out on the Terminal:
Options --type Set type of build to create. Available values are: basic groovy-library java-library pom scala-library
Let's go and use the Java template and create our project structure by executing this bash command:
$ gradle init --type java-library
This template will generate a bunch of files and folders, as shown in the following screenshot. If you have been using Maven, you will see that this structure is similar:
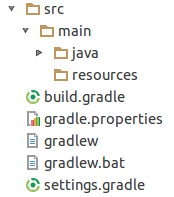
As it stands, the Gradle project is not ready for Kotlin. First, go ahead and delete Library.java and LibraryTest.java, and create a new folder named kotlin, a sibling of the Java one. Then, using a text editor, open the build.gradle file. We need to add the plugin enabling the Gradle system to compile Kotlin code for us. To do this, add the following snippet to the top of your file:
buildscript { ext.kotlin_version = '1.3.31' repositories { mavenCentral() } dependencies { classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" } }
The preceding instructions tell Gradle to use the plugin for Kotlin, and set the dependency Maven repository. Since Kotlin 1.1 is only at milestone 4, there is a specific repository to pick it from (refer to the last entry in repositories). We are not done yet—we still need to enable the plugin. The template generated will already have an applied plugin—java. Replace it with the following:
apply plugin: 'kotlin' apply plugin: 'application' mainClassName = 'com.programming.kotlin.chapter01.ProgramKt'
Now, Kotlin plugin support is enabled. You may have noticed that we have also added the application plugin and set the class containing the program entry point. The reason we have done this is to allow the program to run directly, as we will see shortly.
We are not quite done. We still need to link to the Kotlin standard library. Replace the repositories and dependencies sections with the following:
repositories { mavenCentral() }
dependencies { compile "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version" testCompile 'io.kotlintest:kotlintest-runner-junit5:3.3.2' }
Now, let's create the file named HelloWorld.Kt. This time, we will set a namespace and avoid having our class as part of the default one. If you are not yet familiar with the term, don't worry—it will be covered in Chapter 12, Microservices with Kotlin.
From the Terminal, run the following command:
$ mkdir -p src/main/kotlin/com/programming/kotlin/chapter01 $ echo "" >> src/main/kotlin/com/programming/kotlin/chapter01/Program.kt $ cat <<EOF >> src/main/kotlin/com/programming/kotlin/chapter01/Program.kt package com.programming.kotlin.chapter01 fun main(args: Array<String>) { println("Hello World!") }
We are now in a position to build and run the application, as follows:
$ gradle build $ gradle run
Now, we want to be able to run our program using java -jar [artefact]. Before we can do that, we need to adapt the build.gradle file. First, we need to create a manifest file and set the main class, as follows; the JVM will look for the main function to start executing it:
jar { manifest { attributes( 'Main-Class': 'com.programming.kotlin.chapter01.ProgramKt' ) } from { configurations.compile.collect { it.isDirectory() ? it : zipTree(it) } } }
Furthermore, we also embed the dependency for kotlin-stdlib, as well as kotlin-runtime, into the JAR. If we leave out these dependencies, we will need to add them to the classpath when we run the application. Now, you are ready to build and run the code.