Opening Visual Studio
You’ll need to obtain the rest of the software from within Visual Studio, so let’s open that next. If the installation went as expected, the launch dialog will be on the screen:
- Click on Create a new project. (If you’ve been brought directly into Visual Studio, bypassing the launch dialog, just click File | New Project.)
- It is now time to pick the template we want. Templates make getting started easier. In the Search for Templates box, enter
MAUI
. A few choices will be presented; you want .NET MAUI App, as shown in the following figure:
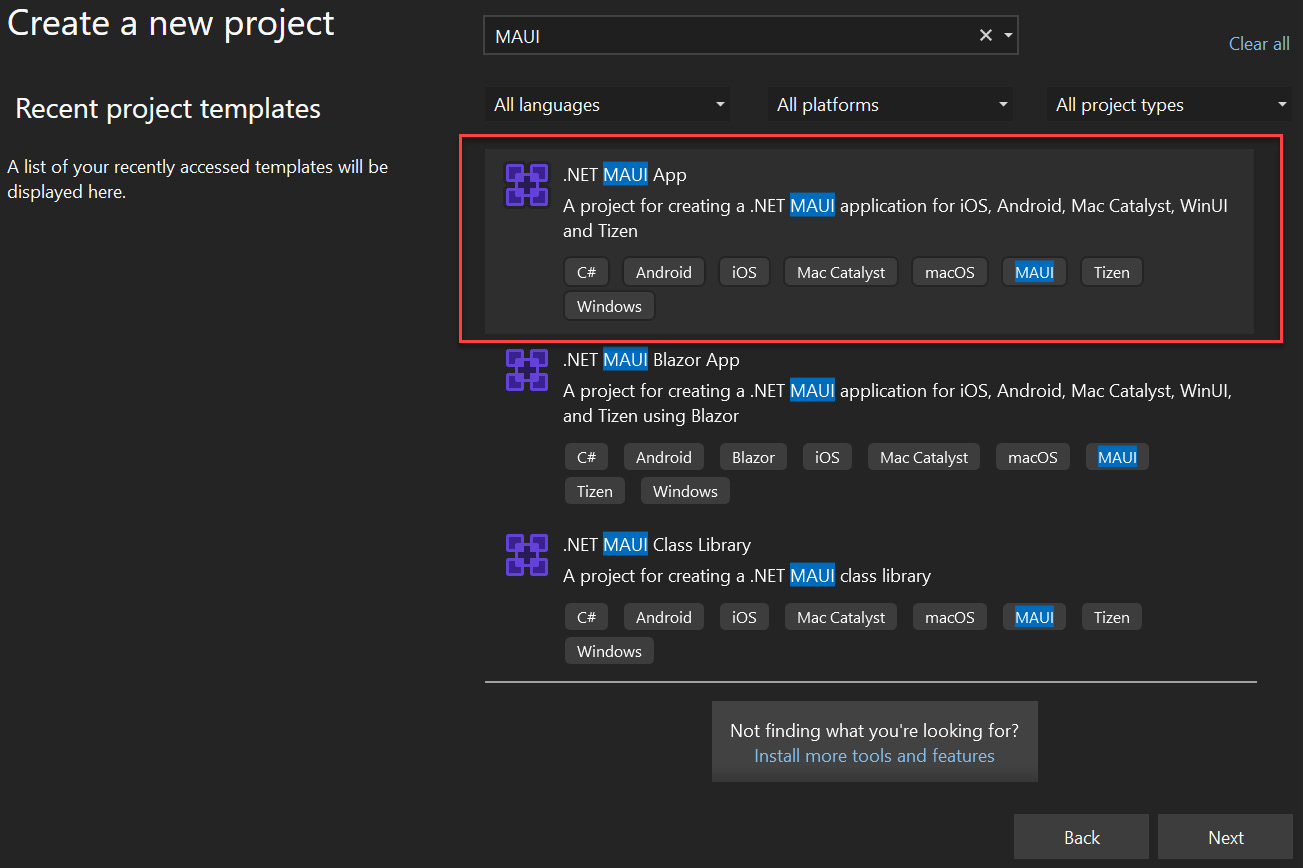
Figure 1.4 – Creating a new project
- Click Next. Here we give our project a name. The first project we’re going to create is not
ForgetMeNotDemo
(the project that you will be building as part of this book), but rather a sample project just to take a quick look around. Name it something creative such asSampleApp
and place it in a location on your disk where you will be able to easily find it later. Before clicking Next, make sure your dialog looks similar to Figure 1.5.
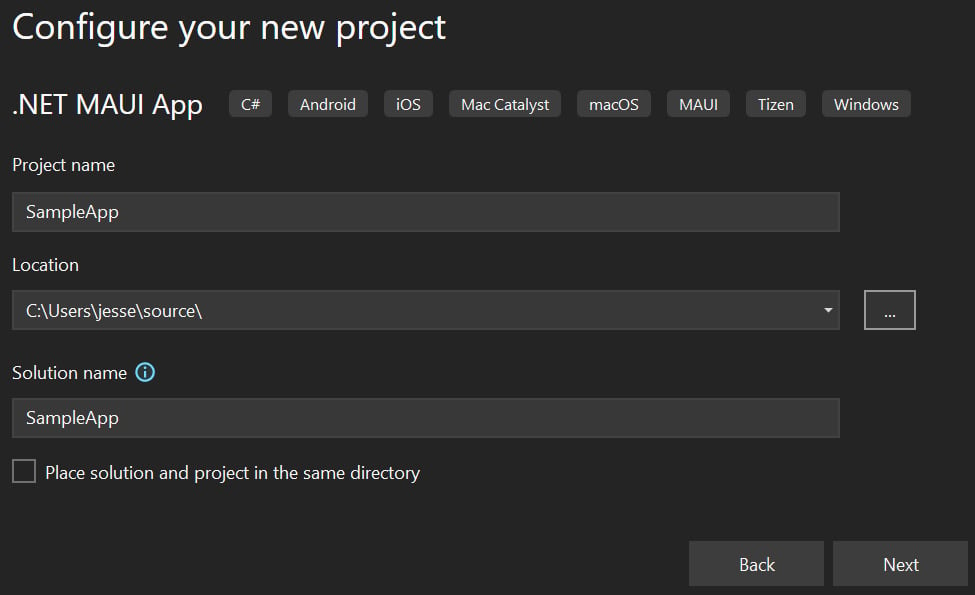
Figure 1.5 – Naming your project
- Click Next and use the dropdown to choose the latest version of .NET. At the time of writing, that is .NET 7. Finally, click on Create.
Note
Because Microsoft is always updating Visual Studio, your screens or steps may vary slightly. Don’t let that worry you. The version I am using is Visual Studio 2022, version 17.4.3. As long as yours is the same or later, you’re all set. But just to be sure, let’s launch the sample app (F5). You should see something that looks like Figure 1.6.
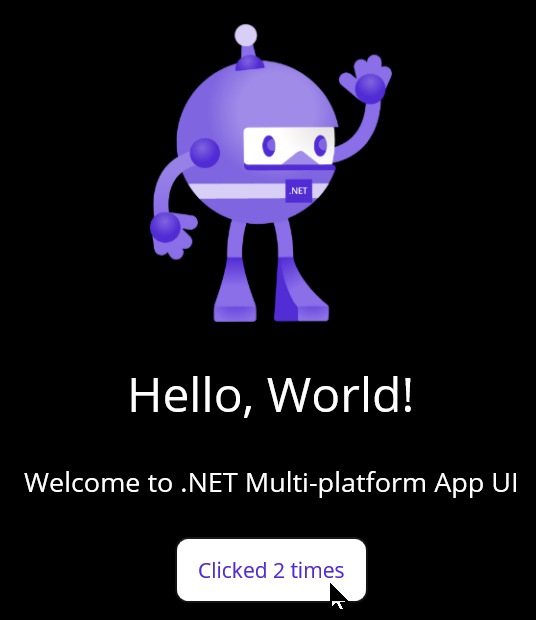
Figure 1.6 – Running your app
- On the screen that you see in the preceding figure, click the button a couple times to make sure it is working.
Generally speaking, I will not be walking through how to do simple things on Visual Studio. The assumption is that you are a C# programmer and so you are probably familiar with Visual Studio. On the other hand, on the off chance that you are not, I’ll describe how to do anything that is not immediately intuitive. Next, let’s explore the out-of-the-box app in a bit more detail.
Quick tour of the app
Let’s take a quick tour to see what comes with an out-of-the-box app. First, stop the app by pressing the red square button in the menu bar. Make sure Solution Explorer is open (if not, go to View | Solution Explorer). Notice that there are three folders and four files, as shown in Figure 1.7:
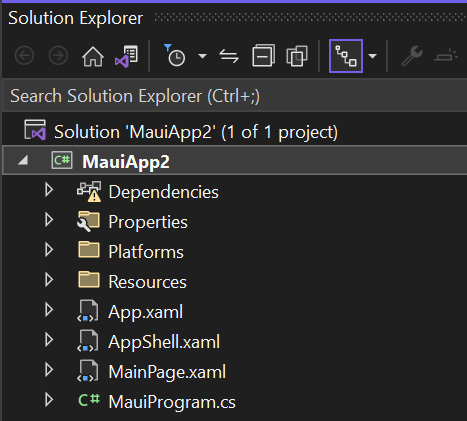
Figure 1.7 – Three folders and four files
The files with the .xaml
extension are XAML files – that is, they use the XAML markup language. I will not assume you know XAML, and in fact, throughout this book, I will provide layout and other code in both XAML and fluent C#, but that is for the next chapter.
Right now, let’s open this out of the
box project
.
This is the entry point for the program. As you can see, it is a static class with a static method that is responsible for creating the app itself. We’ll come back to this file in subsequent chapters.
When you open MainPage.xaml
, you will see a layout with controls for the page we just looked at (with the goofy MAUI guy waving and counting our button clicks). Again, we’re going to come back to layout and controls, but scan this page and see whether you can guess what is going on. You may find that it isn’t as alien as it seemed at first glance. You can, if you are so motivated, learn quite a bit about XAML just by reading this page carefully.
Click on the triangle next to MainPage.xaml
to reveal the code-behind file. Code-behind files are always named <PageName>.xaml.cs
– in this case, MainPage.xaml.cs
. These files are always in C#. Here, we see the constructor and then an event handler. When the user clicks on the button, this event handler (OnCounterClicked
) is called.
By flipping back and forth between the XAML and the code-behind file, you may be able to figure out how the button works and how the count of clicks is displayed. No need to do this, however, as we’ll be covering all these details in upcoming chapters.
At the moment, most of the other files are nearly empty and not worth the time to examine.
Just for fun, expand the Resources
folder. You’ll see that there are folders for the application icon, fonts, images, and so forth. All the resources for all of the platforms are kept here.
Then there is a Platforms
folder, which contains whatever is needed on a per-platform basis. For example, iOS applications require an info.plist
file, which you’ll find in Platforms | iOS.
There is much more to see in a .NET MAUI application, but we will tackle each part as we build Forget Me Not™.