openFrameworks has a built-in implementation of simplex noise, implemented in the ofNoise( t )
function. For example, the following code draws the Perlin noise function, ofNoise( t )
, for t
ranging from 0
to 10
on the screen:
ofSetColor( 0, 0, 0 );
for (int x=0; x<1000; x++) {
float t = x * 0.01;
float y = ofNoise( t );
ofLine( x, 300, x, 300 - y * 300 );
}
Run the code and you will see the following graph:
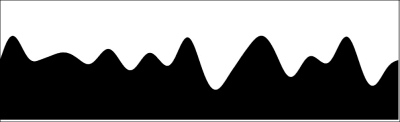
Now replace the line float y = ofNoise( t );
with the following line:
float y = ofNoise( t + 493.0 );
This code renders the noise function in the range [443, 453].
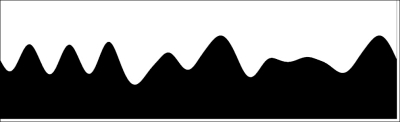
Considering the preceding graphs, you can note the following properties:
The function values are from 0 to 1, and the mean value is clearly about 0.5. Hence you can think about the noise function as describing the fluctuation of some random parameter near its center value, equal to 0.5.
These two graphs depict different ranges of
t
– [0, 10] and [443, 493]. They look different but the scales of fluctuations int
are roughly the same for both these (and actually, any other) ranges of the same width. This property is called statistical homogeneity and means that the statistical properties of the function in the range [t, t+Q] for any fixed and big constantQ
does not depend on any particular value oft
. So you can get as many random functions as you want by just considering the noise function shifted by some constant int
. For example,ofNoise(
t + 293.4
)
andofNoise(
t + 3996.4
)
will generate two distinct and uncorrelated random values for a givent
.
Note
An interesting feature of this noise is that its value, ofNoise( t )
, is equal to 0.5
for all the integer values of t
. So do not wonder when you obtain a constant output from ofNoise( t )
—just check, maybe you are accidentally using integer values for t
.
You can use this function for randomly changing some parameters of the objects in your project; for example, position, size, or angle. Normally, we don't use the pure ofNoise(
t
)
function, but a more complex formula.
float value = amplitude * ofNoise( timePosition + position0 );
Here, amplitude
defines the output range of the value, namely [0, amplitude
]; position0
is a constant (for example, random); and timePosition
is a value that increases with time.
See a simple example of the implementation of this idea for controlling sound volumes in The singing voices example section in Chapter 6, Working with Sounds. Using Perlin noise for creating a knot curve and changing its color is shown in The twisting knot example section in Chapter 7, Drawing in 3D.
Another interesting example is drawing a cloud of a hundred randomly flying points. It can be done with the following code:
ofSetColor( 0, 0, 0 ); float time = ofGetElapsedTimef(); for (int i=0; i<100; i++) { float ampX = ofGetWidth(); float ampY = ofGetHeight(); float speed = 0.1; float posX0 = i * 104.3 + 14.6; float posY0 = i * 53.3 + 35.2; float x = ampX * ofNoise( time * speed + posX0 ); float y = ampY * ofNoise( time * speed + posY0 ); ofCircle( x, y, 10 ); }
Here, constants 104.3
, 14.6
, 53.3
, and 35.2
were chosen quite arbitrarily—just to obtain distinct values for posX0
and posY0
. On running this, you will see a cloud of slowly flying points:
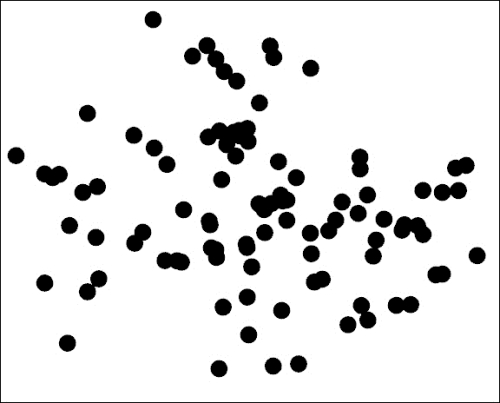
See the further evolution of this example in the Dancing cloud example section in Chapter 6, Working with Sounds.
By summing up several Perlin noises with different scales, it is possible to obtain more interesting noises. See the openFrameworks example in the openFrameworks' folder examples/math/noise1dOctaveExample
. It sums up several noises and you can see the resultant function.
Note
There is a signed version of the ofNoise( t )
function. It's a function called ofSignedNoise( t )
that returns a noise value in the range [-1, 1]. The mean value of the function is zero. Actually, ofSignedNoise( t )
is just equal to 2.0*ofNoise( t ) – 1.0
.
Now we will see how to use multidimensional Perlin noise for generating textures and other fields.