Before we start talking about what Node is and why it's useful, you need to first install Node on your machine, because in the next couple of sections, we'll want to run a little bit of Node code.
Now, to get started, we just need two programs—a browser, I'll be using Chrome throughout the book, but any browser will do, and Terminal. I'll use Spotlight to open up Terminal, which is what it's known as on my operating system.
If you're on Windows, look for the Command Prompt, you can search using the Windows key and then by typing command prompt, and on Linux, you're looking for the command line, although depending on your distribution it might be called Terminal or Command Prompt.
Now, once you have that program open, you'll see a screen, as shown in the following screenshot:
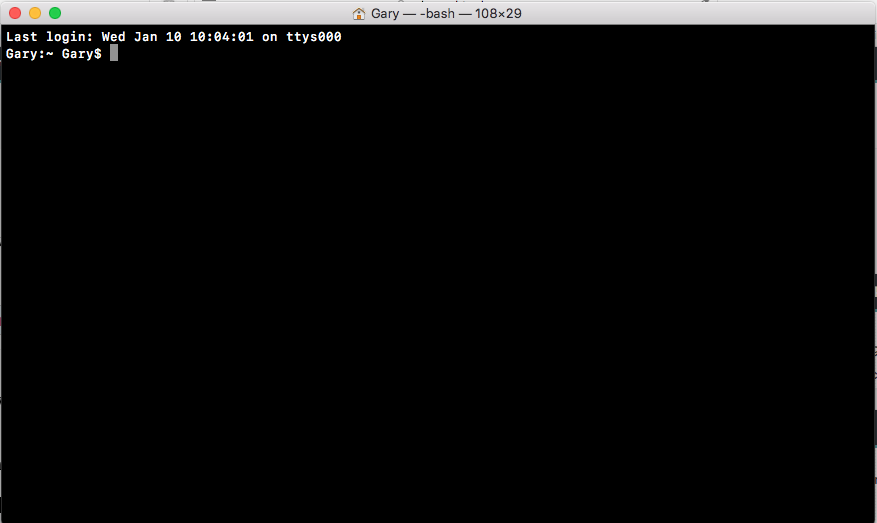
Essentially, it's waiting for you to run a command. We'll run quite a few commands from Terminal throughout the book. I'll discuss it in a few sections later, so if you've never used this before, you can start navigating comfortably.