Before version 4 of Ext JS, Sencha didn't try to impose any kind of structure on our applications. Components were split across multiple files, but other than this, we didn't have any guidance on how they should communicate or how they are pieced together to form an application. Ext JS began with version 1.0 on April 15, 2007 as a library of user interface widgets—buttons, trees, grids, and features (such as layouts) to help them all hang together, but not much more than this.
At the time, this didn't seem to matter much. The idea of a single page application written in JavaScript still felt like the technology of the future, and while it was starting to happen, it certainly wasn't as ordinary as it was in 2014. Augmenting existing web pages with slick Ajax-powered widgets was more than enough.
As time went on, with lots of widgets and dynamic parts of the website all needing to interact, the requirement for a formal mechanism for intercommunication became obvious. People were using Ext JS to build enterprise-level applications that specified high levels of availability and therefore rigorous testing regimes. Spaghetti code just wasn't going to cut it anymore.
Let's take a look at a classic piece of example code from back in the days of Ext 3.4:
// View a working version at https://fiddle.sencha.com/#fiddle/90s // Basic JSON sample data var sampleData = { data: [ { "firstName": "Jack", "surname": "Slocum" }, { "firstName": "Shea", "surname": "Frederick" }, { "firstName": "Colin", "surname": "Ramsay" }, { "firstName": "Steve", "middle": "Cutter", "surname": "Blades" }, { "firstName": "Nigel", "surname": "White" }, ] }; // Create a store to hold our JSON data var userStore = new Ext.data.JsonStore({ data: sampleData, root: 'data', fields: ['firstName', 'middle', 'surname'] }); // Grid panel using the store, setting the columns to match the incoming data var grid = new Ext.grid.GridPanel({ store: userStore, colModel: new Ext.grid.ColumnModel({ defaults: { width: 120, sortable: true }, columns: [ { header: 'First Name', dataIndex: 'firstName' }, { header: 'Middle', dataIndex: 'middle' }, { header: 'Surname', dataIndex: 'surname' } ] }), viewConfig: { forceFit: true }, width: 600, height: 300, frame: true }); // Event handler to do something when the user clicks a row grid.on('rowclick', function(g, idx) { Ext.Msg.alert('Alert', 'You clicked on the row at index ' + idx); }); // Render the grid to the viewport grid.render(document.body);
This block of code should be familiar to the reader as something that generates a populated grid:
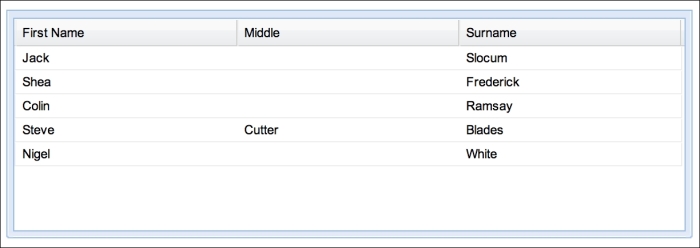
When a row on the grid is clicked on, an alert box appears showing the index of the target row. This is all well and good. It represents a fairly typical example of what you'd have seen in the Ext JS documentation at the time of Ext JS 3.4.
So, what's the problem? The problem is that this code does everything. It sets up our data store, drawing JSON from either a remote or local source. It creates the grid itself along with its many configuration options. It sets up event handling code to pop up the alert box. And after all that's been done, it finally renders the grid to the screen.
Within a simple piece of code like this, the problem is less pronounced. Although, when more functionality is added, such as paging or a detail window popping up with further information about a record and tying all of this into a larger application with server-side interaction, multiple screens, and so on, then things start to get tricky.This is the type of issue that Sencha tried to resolve by introducing defined architectural practices in Ext JS 4. Rather than putting everything together and mixing all these different concerns, the idea was that they could be pulled apart into more logical, concise, and distinct objects.
The model-view-controller (MVC) pattern was the choice of the new wave of web developers. Ruby on Rails had already popularized MVC and many other major frameworks had brought their own ideas to the table.
Tip
While MVC has often been used in web development over recent years, it's used elsewhere in software development and, indeed, was originally developed for desktop GUI coding. Learning about MVC as a general concept is outside our scope, but there's plenty of information and examples on the Web.
Ext JS 4.0 was released on April 26, 2011, over 4 years after v1.0, and many developers were already comfortable with using the MVC pattern in their server-side apps. Frameworks (such as Backbone.js) had slowly begun to give developers the tools to use such architectural patterns in their client-side code. Ext JS 4 needed to do the same and it delivered.
Prior to version 4, Ext JS had stores and records (the "model" in MVC). It had components or widgets (the "view" in MVC). The architectural issue was twofold; how did models and views talk to each other? How did we avoid making our views bloated with logic code?
One answer was to introduce the controller concept. This allowed us to create a layer of glue to create stores, hook them up to components, and use events to manage communication between all of these parts of the application.
In version 5 of Ext JS, a couple of extra features fully answered our architectural questions with ViewControllers and ViewModels. ViewControllers allow us to separate out logic code in our views into a distinct class, ensuring that both the view and ViewController can focus on their own set of responsibilities.
As well as building classes into the Ext JS framework to help us build complicated applications, Sencha has also provided a number of supplementary tools that assist with the development process. In particular, Sencha Cmd is a powerful command-line tool that introduces some indispensable facilities.
From the beginning, Sencha Cmd can help by generating the directory structure of your application with a best practice layout, specifically created to help with the new Ext JS 5 cross-device support. You can add new models, controllers, and so on, all from the command line.
As you're developing, Sencha Cmd can help you by compiling theme files, slicing and dicing images, and running a development web server to run your code locally. Also, during testing and deployment, it can package your code into an optimized bundle to give your users the minimum amount of code to download in their browser.
Sencha Cmd is a great example of a product that is growing up. It represents a key piece of your development infrastructure and a fantastic complement to the Ext JS framework itself.