Not so long ago, every time you started working for a new company, you would spend an important part of your first few days setting up your new environment—that is, installing all the necessary tools on your new computer in order to be able to code. This was incredibly frustrating because even though the software to install was the same, there was always something that failed or was missing, and you would spend less time being productive.
Luckily for us, people tried to fix this big problem. First, we have virtual machines, which are emulations of computers inside your own computer. With this, we can have Linux inside our MacBook, which allows developers to share environments. It was a good step, but it still had some problems; for example, VMs were quite big to move between different environments, and if developers wanted to make a change, they had to apply the same change to all the existing virtual machines in the organization.
After some deliberation, a group of engineers came up with a solution to these issues and we got Vagrant. This amazing software allows you to manage virtual machines with simple configuration files. The idea is simple: a configuration file specifies which base virtual machine we need to use from a set of available ones online and how you would like to customize it—that is, which commands you will want to run the first time you start the machine—this is called "provisioning". You will probably get the Vagrant configuration from a public repository, and if this configuration ever changes, you can get the changes and reprovision your machine. It's easy, right?
If you still do not have Vagrant, installing it is quite easy. You will need to visit the Vagrant download page at https://www.vagrantup.com/downloads.html and select the operating system that you are working with. Execute the installer, which does not require any extra configuration, and you are good to go.
Using Vagrant is quite easy. The most important piece is the Vagrantfile
file. This file contains the name of the base image we want to use and the rest of the configuration that we want to apply. The following content is the configuration needed in order to get an Ubuntu VM with PHP 7, MySQL, Nginx, and Composer. Save it as Vagrantfile
at the root of the directory for the examples of this book.
VAGRANTFILE_API_VERSION = "2" Vagrant.configure(VAGRANTFILE_API_VERSION) do |config| config.vm.box = "ubuntu/trusty32" config.vm.network "forwarded_port", guest: 80, host: 8080 config.vm.provision "shell", path: "provisioner.sh" end
As you can see, the file is quite small. The base image's name is ubuntu/trusty32
, messages to our port 8080
will be redirected to the port 80
of the virtual machine, and the provision will be based on the provisioner.sh
script. You will need to create this file, which will be the one that contains all the setup of the different components that we need. This is what you need to add to this file:
#!/bin/bash sudo apt-get install python-software-properties -y sudo LC_ALL=en_US.UTF-8 add-apt-repository ppa:ondrej/php -y sudo apt-get update sudo apt-get install php7.0 php7.0-fpm php7.0-mysql -y sudo apt-get --purge autoremove -y sudo service php7.0-fpm restart sudo debconf-set-selections <<< 'mysql-server mysql-server/root_password password root' sudo debconf-set-selections <<< 'mysql-server mysql-server/root_password_again password root' sudo apt-get -y install mysql-server mysql-client sudo service mysql start sudo apt-get install nginx -y sudo cat > /etc/nginx/sites-available/default <<- EOM server { listen 80 default_server; listen [::]:80 default_server ipv6only=on; root /vagrant; index index.php index.html index.htm; server_name server_domain_or_IP; location / { try_files \$uri \$uri/ /index.php?\$query_string; } location ~ \.php\$ { try_files \$uri /index.php =404; fastcgi_split_path_info ^(.+\.php)(/.+)\$; fastcgi_pass unix:/var/run/php/php7.0-fpm.sock; fastcgi_index index.php; fastcgi_param SCRIPT_FILENAME \$document_root\$fastcgi_script_name; include fastcgi_params; } } EOM sudo service nginx restart
The file looks quite long, but we will do quite a lot of stuff with it. With the first part of the file, we will add the necessary repositories to be able to fetch PHP 7, as it does not come with the official ones, and then install it. Then, we will try to install MySQL, server and client. We will set the root password on this provisioning because we cannot introduce it manually with Vagrant. As this is a development machine, it is not really a problem, but you can always change the password once you are done. Finally, we will install and configure Nginx to listen to the port 8080
.
To start the virtual machine, you need to execute the following command in the same directory where Vagrantfile
is:
$ vagrant up
The first time you execute it, it will take some time as it will have to download the image from the repository, and then it will execute the provisioner.sh
file. The output should be something similar to this one followed by some more output messages:
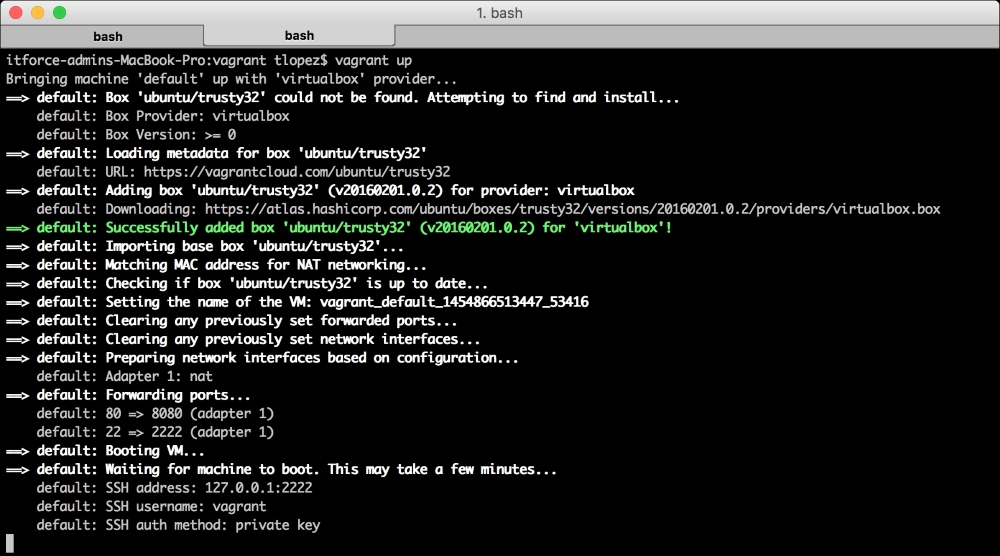
In order to access your new VM, run the following command on the same directory where you have your Vagrantfile
file:
$ vagrant ssh
Vagrant will start an SSH session to the VM, which means that you are inside the VM. You can do anything you would do with the command line of an Ubuntu system. To exit, just press Ctrl + D.
Sharing files from your laptop to the VM is easy; just move or copy them to the same directory where your Vagrantfile
file is, and they will "magically" appear on the /vagrant
directory of your VM. They will be synchronized, so any changes that you make while in your VM will be reflected on the files of your laptop.
Once you have a web application and you want to test it through a web browser, remember that we will forward the ports. This means that in order to access the port 80
of your VM, the common one for web applications, you will have to point to the port 8080
on your browsers; here's an example: http://localhost:8080
.