React is an open source JavaScript library that provides a view-layer for rendering data as HTML to create interactive UI components. Components have been used typically to render React views that contain additional components specified as custom HTML tags. React views efficiently update and re-render the components without reloading the page when your data changes. It gives you a trivial virtual DOM, powerful views without templates, unidirectional data flow, and explicit mutation. It is a very systematic way of updating the HTML document when the data changes and provides a clean separation of components in a modern, single-page application.
The React Component is built entirely with Javascript, so it's easy to pass rich data through your app. Creating components in React lets you split the UI into reusable and independent pieces, which makes your application component reusable, testable, and makes the separation of concerns easy.
React is only focused on View in MVC, but it also has stateful components that remember everything within this.state
. It handles mapping from input to state changes and it renders components. Let's look at React's component life cycle and its different levels.
In React, each component has its own lifecycle methods. Every method can be overridden as per your requirements.
When the data changes, React automatically detects the change and re-renders the component. Also, we can catch the errors in the Error Handling
phase.
The following image shows the phases of a React Component:
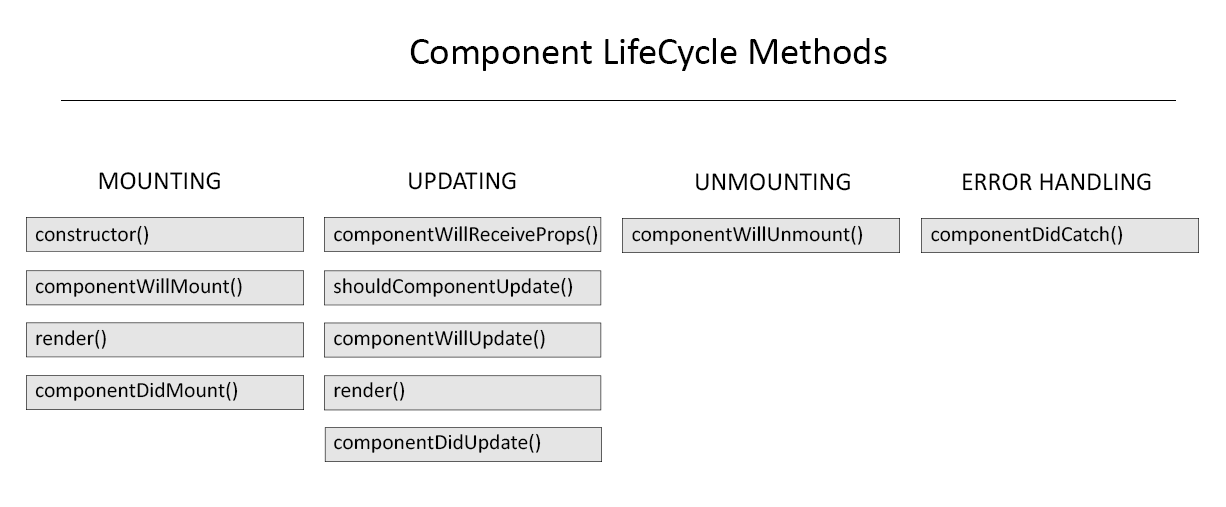
Let's take a quick look at the preceding methods.
The constructor method of React Component gets invoked first when the component is mounted. Here, we can set the state of the component.
Here's an example of constructor in React.Component
:
constructor(props) { super(props); this.state = { value: props.initialValue }; }
Note
Using this.props
inside the constructor, we need to call super(props)
to access and call functions of parents; otherwise, you will get this.props
undefined in the constructor because React sets the .props
on the instance from outside immediately after calling constructor, but it will not affect when you are usingthis.props
inside the render method.
The render()
method is required to render the UI component and examine this.props
and this.state
and return one of the following types:
- React elements
- String and numbers
- Portals
- null
- Booleans
This method is invoked immediately after a component gets the mount. We can use this method to load the data from a remote endpoint to instantiate a network request.
This method will be invoked when the mounted component receives new props. This method also allows comparing the current and next values to ensure the changes in props.
The shouldComponentUpdate()
method is invoked when the component has received the new props and state. The default value is true
; if it returns false
, React skips the update of the component.
The componentWillUpdate()
method is invoked immediately before rendering when a new prop or state is being received. We can use this method to perform an action before the component gets updated.
The componentDidUpdate()
method is invoked immediately when component gets updated. This method is not called for the initial render.
This method is invoked immediately before a React Component is unmounted and destroyed. Here, we can perform any necessary cleanup, such as canceling network requests or cleaning up any subscription that was created in componentDidMount
.
This method allows us to catch the JavaScript errors in React Components. We can log those errors and display another fallback UI instead of the component tree that crashed.
Now we have a clear idea about component methods that are available in React Components.
Observe the following JavaScript code snippet:
<section> <h2>My First Example</h2> </section> <script> var root = document.querySelector('section').createShadowRoot(); root.innerHTML = '<style>h2{ color: red; }</style>' +'<h2>Hello World!</h2>'; </script>
Now, observe the following ReactJS code snippet:
var sectionStyle = { color: 'red' }; var MyFirstExample = React.createClass({ render: function() { return (<section><h2 style={sectionStyle}> Hello World!</h2></section> )} }) ReactDOM.render(<MyFirstExample />, renderedNode);
Now, after observing the preceding examples of React and JavaScript, we will have a clear idea of normal HTML encapsulation and ReactJS custom HTML tags.
Note
React isn't an MVC framework; it's a library for building a composable user interface and reusable components. React is used at Facebook in its production stages and instagram.com is entirely built on React.