Agile is a software development methodology. As the name suggests, it is quick and supports ease of change. Agile is an idea supported by a set of values and beliefs.
Waterfall or sequential project development process is unpredictable; prediction doesn't have any base, it is mostly done using someone's experience or guess work. For example, in waterfall, project management commits to a customer that software will be delivered within 12 months; but in reality, PM doesn't have any base to predict this and the predictions fail.
Agile is more predictable—it is iterative and incremental. In Scrum, an iteration is called a sprint.
Sprint time varies from a couple of weeks to a couple of months. To learn more about the Agile manifesto, visit the following website:
Scrum is a very popular implementation of the Agile methodology. Scrum manifests for a self-organized team, continuous feedback, incremental build, and testing.
To achieve this, software requirements are broken into small testable pieces. Each testable requirement piece is called a user story. A group of stories is called an epic, a group of epics called a feature.
A story description is self-explanatory.
Here is an example of a story: "As an admin user I can log in to the admin console". Each story must have an acceptance test associated with it. Acceptance tests are the criterion of acceptance. Following is an example of AT:
Log in with admin user credentials, admin menu should be visible
Log in with normal user credentials, admin menu should not be visible
Before accepting a story, testers verify the software and check if ATs are met. If development or testing is blocked for an issue, the team focuses on resolving the issue. At the end of each sprint, stories are demonstrated to customers and feedback is taken.
Scrum is a type of nonbureaucratic management. Instead of someone outside, the team decides what will be delivered.
Scrum comes up with three roles:
Product manager: The manager shadows customers and provides what they require
Scrum master: The Scrum master facilitates the team
Scrum team: The team consists of analysts, architects, developers, testers, tech writers, and so on
Scrum doesn't advocate for long meetings. Although it defines five meetings:
Backlog grooming: Customers (including the product manager) come up with user requirements. Before each sprint, the product backlog is created or modified based on customer requirements.
During backlog grooming meeting, requirements are understood and big requirements are broken into epics and testable user stories.
The team also carries out complexity estimation (aka T-shirt size of the story). Pointing range is a Fibonacci series—1, 2, 3, 5, 8, 13,...
Here, 1 represents a very trivial number or a least complex task. 2 means complexity of work is twice as of 1.
Team decides what 1 is, it could be adding a widget to an UI for display or a SQL to fetch data.
Sprint planning: Sprint planning is scheduled at the beginning of the sprint. The product owner explains what are the real business import features for the customers, and then the team decides what the epics/stories do, which will be included in the coming sprint. The team considers the T-shirt size.
Finally the team pulls in stories from product backlog to sprint backlog.
Daily stand-up meeting: Every day the Scrum team members spend a total of 15 minutes reporting to each other. The agenda is for summarizing the work of the previous day, current day, and to determine whether any help is required (due to any impediment).
Each team member speaks about his/her status. As the name suggests, standing up at the meeting helps to reduce the time. The meeting should not exceed more than 15 minutes. If anyone is blocked or anything critical needs to be resolved, then only the required members meet again after the stand-up meeting.
Sprint review: After a Sprint ends, the team holds a sprint review meeting to demonstrate a working product to the product owner and the stakeholders.
After the demonstration, the product owner reviews the sprint backlog (created during the planning) and declares which items are considered as complete.
If anything was committed but not done, team provides the explanation to all stakeholders for the slippage.
Retrospective: Reflection in the mirror tells you who you are. In the retrospective meeting, the team reflects on its own process, inspects behavior/process, and takes action for future sprints.
Each member, not mandatorily, speaks about things that went wrong, went fine, and those that made him/her mad.
The Scrum master helps to identify the owner of each item and owner takes action. One example could be that white board/projector/meeting rooms weren't available for critical technical walkthrough. Someone can take this item and work with office administrators to allocate a dedicated meeting room for the team.
Another example could be that analysts spoke about many things but did not document them.
Scrum teams keep a board with working stories. This board reflects the status of the team. The board contains many columns, such as On Deck/TO-DO, Analysis Active, Analysis Done, Development, Development Done, Testing, and Done. You can visit scrumy.com to get a clearer picture.
TODO represents the stories accepted by the team, Analysis active shows that stories are being worked on by the business analysts, Analysis Done represents stories that will be picked up by the developers, developers work on Development stories, Testing column represents stories being tested by the testers, and the Done column represents stories accepted by the team.
The following figure represents a story board:
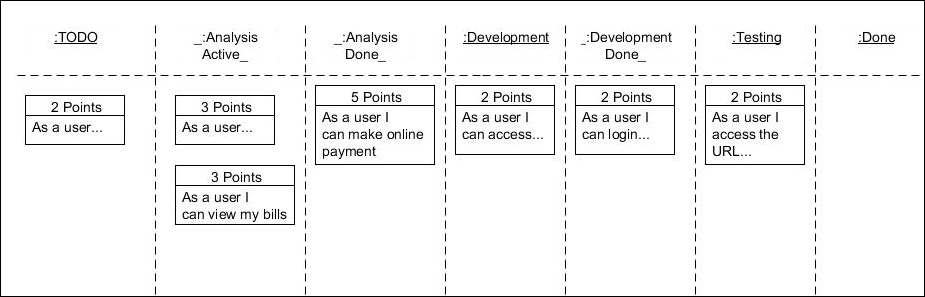
Kanban is a highly efficient way of managing software development processes.
The software development process mainly consists of three key things: analysis, development, and testing. The progress of the process depends on the progress of these three areas. If analysts deliver five features in a week, developers code 10 features in a week; but testers can test only two features per week, then the output of the software process is two features per week.
In the preceding example, if analysts and developers keep delivering five and 10 features respectively, then after the second week testing will block 16 (20 - 4 = 16) features.
Here, testing is the bottleneck. So the progress of the software development process is the progress of the bottleneck.
The Kanban process helps to resolve the bottleneck, it introduces a work-in-progress (WIP) limit. Kanban has a story board and each swim lane in the board has a work-in-progress limit.
These work-in-progress limits are the critical difference between a Kanban board and Scrum story board. Limiting the amount of work-in-progress at each step prevents bottlenecks dynamically.
Development can have a maximum work-in-progress limit. Once maximum features are code, the developers cannot take any more features. Instead, they will help in testing. Hence, the software development flow is not stuck. Similarly, analysts will help with testing when done with analysis.
In the preceding example, testing was a bottleneck. But in a real project, a bottleneck could be development, analysis, or testing.
The main theme is to control the flow and resolve bottlenecks. Visit the following URL to get a feel of a Kanban story board:
The following figure represents a Kanban story board with a WIP limit:
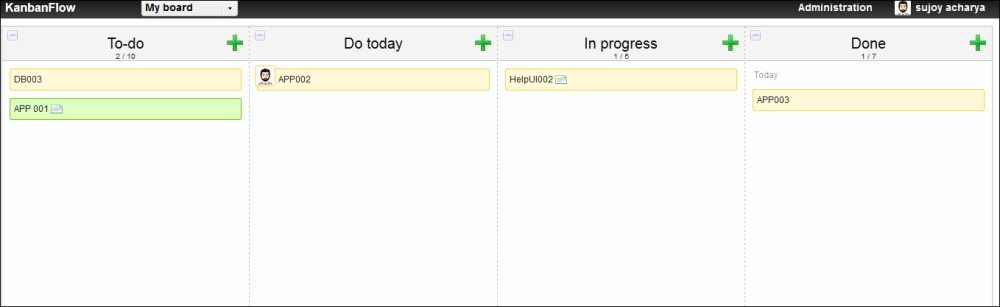
The following figure represents a Kanban board with a WIP limit exceeded:
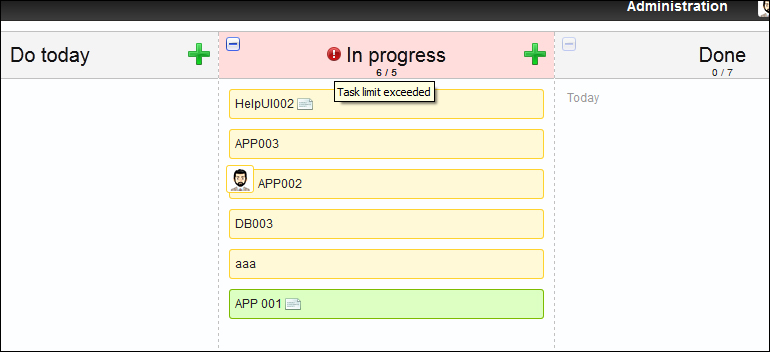