F# is very well integrated into Visual Studio 2010 and higher versions. This is a first-class language along with C# and VB.NET. There is also support for F# in cross-platform integrated development environments (IDEs) such as Xamarin Studio, the Ionide extension for Visual Studio Code, or Atom. See http://fsharp.org/ for more information.
Visual Studio includes the default project templates for F#. We can look for Visual F# and see a list of templates available.
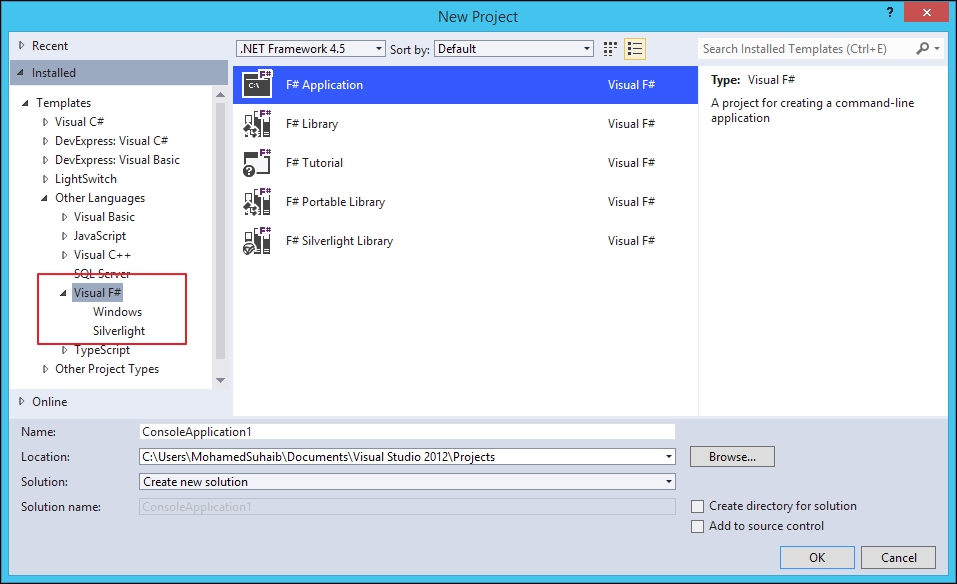
Note
The screenshots in this book have been taken with Visual Studio 2012, but they should be very similar to other Visual Studio versions.
For example, let's create an F# console application called FSharpHelloWorld
and understand the different aspects of an F# project. The Solution Explorer lists the following files:
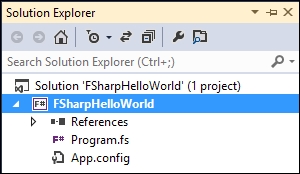
Open Program.fs
and view the contents; it has very low-ceremony code to run the console application, which is as follows:
[<EntryPoint>] let main argv = printfn "%A" argv 0 // return an integer exit code
If you are a C# developer, some elements of this little program may surprise you. For example, the EntryPoint
attribute indicates which function must be run when a console application starts. Also, as mentioned in the preceding code snippet, every expression in F# returns a value, so there is no need for an explicit return
keyword.
Modify the printfn
statement to include "Hello World"
, as shown in the following code snippet:
[<EntryPoint>] let main argv = printfn "Hello from F# :)" 0 // return an integer exit code
F# projects follow a top-to-bottom structuring of program files. Unlike C# or VB.NET, this is the very first thing to understand to organize files and folders with F#.
Note
Folder management is not available in F# within Visual Studio by default; you will need to install the Visual F# Power Tools extension for that.
You will learn by adding some files to the hello world project, as follows:
- Add a new F#
Employee.fs
source file to the hello world project - The
Employee.fs
file gets added to the end of the project - Press ALT + the up arrow, or right-click and move up or down, to arrange the file as shown in the following screenshot:
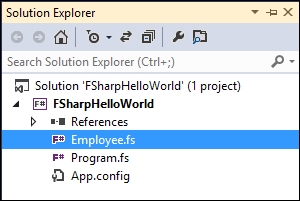
With F#, you can not write projects (.fsproj
) containing module files (.fs
), but also scripts (.fsx
). These are single-file projects that are useful to write short programs, automation tasks, and so on.
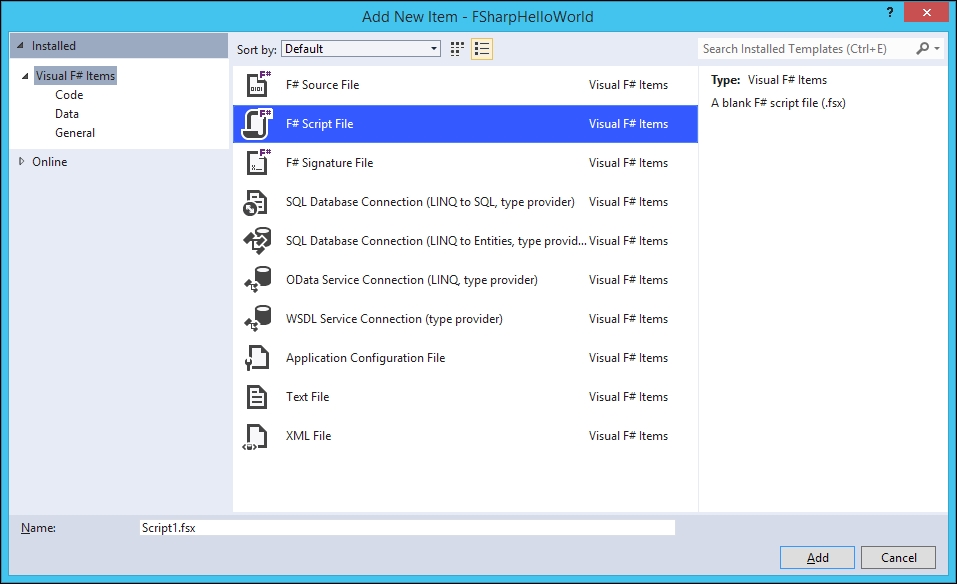
With F# Script File, we will get the following features:
- Full IntelliSense support
- Loading external files or .NET libraries
- Prototyping code that can easily be moved to a real codebase with minimal or no code changes
- An F# interactive window in Visual Studio that can be used to quickly test parts of the code from your F# script or normal F# files
The F# Interactive window provides a read-evaluate-print-loop (REPL) to evaluate your expressions without having to create, build, and run an F# project. This is very useful for developers to work in exploratory mode.
The following are the two ways to use the F# Interactive window:
- Visual Studio
- Command line
To access the F# Interactive window from the menu, navigate to View | Other Windows | F# Interactive.
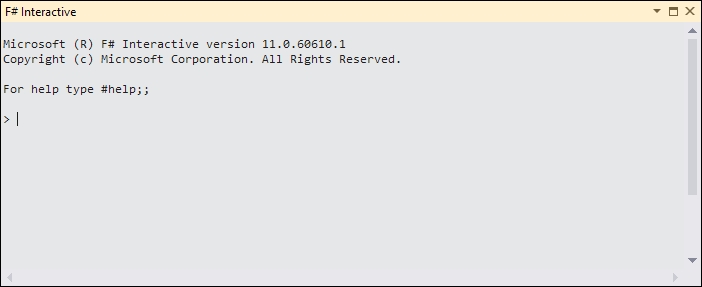
Note
To evaluate an expression directly in the interactive window, terminate the code with double semicolons (;;
). This is not necessary in the F# source code.
Use the F# script files to quickly check a piece of code. We will now check this feature by writing a square root and a Fibonacci function. Add the following functions to a script file:
let sqr x = x * x let rec fib n = if n < 2 then 1 else fib (n - 2) + fib (n - 1)
After adding the preceding code snippet in the script file, perform the following steps:
Right-click on the selected code and select Execute In Interactive or press Alt + Enter.
- This will send the functions to the F# Interactive window. The window then displays the signature of the functions. Note that the syntax for signatures is a bit different from what we saw before. In this case, it means both functions accept and return an
int
value. We can call the functions directly in the interactive window by applying an
int
argument to the name of the function, as shown in the following screenshot:
There are some idioms that you will need to be familiar with when using F# Script File or the F# Interactive mode.
Idioms |
Description |
|
This loads a script/F# file. For example, refer to the following piece of code: #load "sample.fs" #load "sample.fsx"
|
|
This refers to a folder to load the assemblies in. For example, refer to the following piece of code: #I @"C:\Program Files (x86)\Reference Assemblies\Microsoft\Framework\.NETFramework\v4.0"
|
|
This refers to the assembly to load from the referenced folder. Usually, this is used in conjunction with #I.
For example, refer to the following piece of code:
#I @"C:\Program Files (x86)\Reference Assemblies\Microsoft\Framework\.NETFramework\v4.0" #r "presentationcore.dll"
|
|
This displays information about available directives. |
|
This terminates the interactive session. |
|
The |
FSI interactive shell is a simple console app that is bundled with the F# installation. To open FSI, perform the following steps:
- Open a terminal window.
- Type
fsi
to log in to the FSI session. Try some simple code to evaluate.