This recipe extends the previous one. Therefore, it is necessary to have typed Recipe 1 yourself into a project of your own or downloaded the code from https://www.packtpub.com/support.
We are preventing the GUI from being resized.
import tkinter as tk # 1 imports win = tk.Tk() # 2 Create instance win.title("Python GUI") # 3 Add a title win.resizable(0, 0) # 4 Disable resizing the GUI win.mainloop() # 5 Start GUI
Running the code creates this GUI:
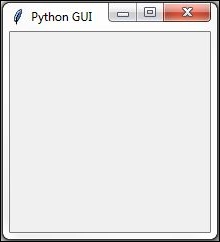
Line 4 prevents the Python GUI from being resized.
Running this code will result in a GUI similar to the one we created in Recipe 1. However, the user can no longer resize it. Also, notice how the maximize button in the toolbar of the window is grayed out.
Why is this important? Because, once we add widgets to our form, resizing can make our GUI look not as good as we want it to be. We will add widgets to our GUI in the next recipes.
Resizable()
is a method of the Tk()
class and, by passing in (0, 0),
we prevent the GUI from being resized. If we pass in other values, we hard-code the x and y start up size of the GUI, but that won't make it nonresizable.
We also added comments to our code in preparation for the recipes contained in this book.