In this recipe, we will improve our GUI by adding drop-down combo boxes that can have initial default values. While we can restrict the user to only certain choices, at the same time, we can allow the user to type in whatever they wish.
We are inserting another column between the Entry
widget and the Button
using the grid layout manager. Here is the Python code.
ttk.Label(win, text="Choose a number:").grid(column=1, row=0) # 1 number = tk.StringVar() # 2 numberChosen = ttk.Combobox(win, width=12, textvariable=number) #3 numberChosen['values'] = (1, 2, 4, 42, 100) # 4 numberChosen.grid(column=1, row=1) # 5 numberChosen.current(0) # 6
This code, when added to previous recipes, creates the following GUI. Note how, in line 4 in the preceding code, we assign a tuple with default values to the combo box. These values then appear in the drop-down box. We can also change them if we like (by typing in different values when the application is running).
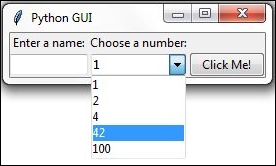
Line 1 adds a second label to match the newly created combo box (created in line 3). Line 2 assigns the value of the box to a variable of a special tkinter
type (StringVar
), as we did in a previous recipe.
Line 5 aligns the two new controls (label and combo box) within our previous GUI layout, and line 6 assigns a default value to be displayed when the GUI first becomes visible. This is the first value of the numberChosen['values']
tuple, the string "1"
. We did not place quotes around our tuple of integers in line 4, but they got casted into strings because, in line 2, we declared the values to be of type tk.StringVar
.
The screenshot shows the selection made by the user (42). This value gets assigned to the number
variable.
If we want to restrict the user to only be able to select the values we have programmed into the Combobox
, we can do that by passing the state property into the constructor. Modify line 3 in the previous code to:
numberChosen = ttk.Combobox(win, width=12, textvariable=number, state='readonly')
Now users can no longer type values into the Combobox
. We can display the value chosen by the user by adding the following line of code to our Button Click Event Callback function:
# Modified Button Click Callback Function def clickMe(): action.configure(text='Hello ' + name.get()+ ' ' + numberChosen.get())
After choosing a number, entering a name, and then clicking the button, we get the following GUI result, which now also displays the number selected:
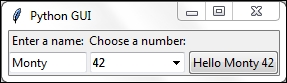