In this recipe, we will go in the opposite direction to the previous recipe and try to call our tkinter GUI code from within a wxPython GUI.
We will reuse some of the wxPython GUI code we created in a previous recipe in this chapter.
We will start from a simple wxPython GUI, which looks like this:
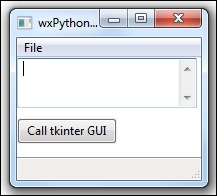
Next, we will try to invoke a simple tkinter GUI.
This is the entire code to do this in a simple, non-OOP way:
#============================================================= def tkinterApp(): import tkinter as tk from tkinter import ttk win = tk.Tk() win.title("Python GUI") aLabel = ttk.Label(win, text="A Label") aLabel.grid(column=0, row=0) ttk.Label(win, text="Enter a name:").grid(column=0, row=0) name = tk.StringVar() nameEntered = ttk.Entry(win, width=12, textvariable=name) nameEntered.grid(column=0, row=1) nameEntered.focus() def buttonCallback(): action...