When starting with Go, one of the most common things you'll hear being said is that it's a systems language.
Indeed, one of the earlier descriptions of Go, by the Go team itself, was that the language was built to be a modern systems language. It was constructed to combine the speed and power of languages, such as C with the syntactical elegance and thrift of modern interpreted languages, such as Python. You can see that goal realized when you look at just a few snippets of Go code.
From the Go FAQ on why Go was created:
"Go was born out of frustration with existing languages and environments for systems programming."
Perhaps the largest part of present-day Systems programming is designing backend servers. Obviously, the Web comprises a huge, but not exclusive, percentage of that world.
Go hasn't been considered a web language until recently. Unsurprisingly, it took a few years of developers dabbling, experimenting, and finally embracing the language to start taking it to new avenues.
While Go is web-ready out of the box, it lacks a lot of the critical frameworks and tools people so often take for granted with web development now. As the community around Go grew, the scaffolding began to manifest in a lot of new and exciting ways. Combined with existing ancillary tools, Go is now a wholly viable option for end-to-end web development. But back to that primary question: Why Go? To be fair, it's not right for every web project, but any application that can benefit from high-performance, secure web-serving out of the box with the added benefits of a beautiful concurrency model would make for a good candidate.
In this book, we're going to explore those aspects and others to outline what can make Go the right language for your web architecture and applications.
We're not going to deal with a lot of the low-level aspects of the Go language. For example, we assume you're familiar with variable and constant declaration. We assume you understand control structures.
In this chapter we will cover the following topics:
- Installing Go
- Structuring a project
- Importing packages
- Introducing the net package
- Hello, Web
The most critical first step is, of course, making sure that Go is available and ready to start our first web server.
Note
While one of Go's biggest selling points is its cross-platform support (both building and using locally while targeting other operating systems), your life will be much easier on a Nix compatible platform.
If you're on Windows, don't fear. Natively, you may run into incompatible packages, firewall issues when running using go run
command and some other quirks, but 95% of the Go ecosystem will be available to you. You can also, very easily, run a virtual machine, and in fact that is a great way to simulate a potential production environment.
In-depth installation instructions are available at https://golang.org/doc/install, but we'll talk about a few quirky points here before moving on.
For OS X and Windows, Go is provided as a part of a binary installation package. For any Linux platform with a package manager, things can be pretty easy.
On both OS X and Linux, you'll need to add a couple of lines to your path—the GOPATH
and PATH
. First, you'll want to find the location of your Go binary's installation. This varies from distribution to distribution. Once you've found that, you can configure the PATH
and GOPATH
, as follows:
export PATH=$PATH:/usr/local/go/bin export GOPATH="/usr/share/go"
While the path to be used is not defined rigidly, some convention has coalesced around starting at a subdirectory directly under your user's home directory, such as $HOME/go
or ~Home/go
. As long as this location is set perpetually and doesn't change, you won't run into issues with conflicts or missing packages.
You can test the impact of these changes by running the go env
command. If you see any issues with this, it means that your directories are not correct.
Note that this may not prevent Go from running—depending on whether the GOBIN directory is properly set—but will prevent you from installing packages globally across your system.
To test the installation, you can grab any Go package by a go get
command and create a Go file somewhere. As a quick example, first get a package at random, we'll use a package from the Gorilla framework, as we'll use this quite a bit throughout this book.
go get github.com/gorilla/mux
If this runs without any issue, Go is finding your GOPATH
correctly. To make sure that Go is able to access your downloaded packages, draw up a very quick package that will attempt to utilize Gorilla's mux package and run it to verify whether the packages are found.
package main import ( "fmt" "github.com/gorilla/mux" "net/http" ) func TestHandler(w http.ResponseWriter, r *http.Request) { } func main() { router := mux.NewRouter() router.HandleFunc("/test", TestHandler) http.Handle("/", router) fmt.Println("Everything is set up!") }
Run go run test.go
in the command line. It won't do much, but it will deliver the good news as shown in the following screenshot:

When you're first getting started and mostly playing around, there's no real problem with setting your application lazily.
For example, to get started as quickly as possible, you can create a simple hello.go
file anywhere you like and compile without issue.
But when you get into environments that require multiple or distinct packages (more on that shortly) or have more explicit cross-platform requirements, it makes sense to design your project in a way that will facilitate the use of the go build tool.
The value of setting up your code in this manner lies in the way that the go build tool works. If you have local (to your project) packages, the build tool will look in the src
directory first and then your GOPATH
. When you're building for other platforms, go build will utilize the local bin folder to organize the binaries.
When building packages that are intended for mass use, you may also find that either starting your application under your GOPATH
directory and then symbolically linking it to another directory, or doing the opposite, will allow you to develop without the need to subsequently go get your own code.
As with any language, being a part of the Go community means perpetual consideration of the way others create their code. Particularly if you're going to work in open source repositories, you'll want to generate your code the way that others do, in order to reduce the amount of friction when people get or include your code.
One incredibly helpful piece of tooling that the Go team has included is go fmt
. fmt
here, of course, means format and that's exactly what this tool does, it automatically formats your code according to the designed conventions.
By enforcing style conventions, the Go team has helped to mitigate one of the most common and pervasive debates that exist among a lot of other languages.
While the language communities tend to drive coding conventions, there are always little idiosyncrasies in the way individuals write programs. Let's use one of the most common examples around—where to put the opening bracket.
Some programmers like it on the same line as the statement:
for (int i = 0; i < 100; i++) { // do something }
While others prefer it on the subsequent line:
for (int i = 0; i < 100; i++) { // do something }
These types of minor differences spark major, near-religious debates. The Gofmt tool helps alleviate this by allowing you to yield to Go's directive.
Now, Go bypasses this obvious source of contention at the compiler, by formatting your code similar to the latter example discussed earlier. The compiler will complain and all you'll get is a fatal error. But the other style choices have some flexibility, which are enforced when you use the tool to format.
Here, for example, is a piece of code in Go before go fmt
:
func Double(n int) int { if (n == 0) { return 0 } else { return n * 2 } }
Arbitrary whitespace can be the bane of a team's existence when it comes to sharing and reading code, particularly when every team member is not on the same IDE.
By running go fmt
, we clean this up, thereby translating our whitespace according to Go's conventions:
func Double(n int) int { if n == 0 { return 0 } else { return n * 2 } }
Long story short: always run go fmt
before shipping or pushing your code.
Code conventions
As with any language, being a part of the Go community means perpetual consideration of the way others create their code. Particularly if you're going to work in open source repositories, you'll want to generate your code the way that others do, in order to reduce the amount of friction when people get or include your code.
One incredibly helpful piece of tooling that the Go team has included is go fmt
. fmt
here, of course, means format and that's exactly what this tool does, it automatically formats your code according to the designed conventions.
By enforcing style conventions, the Go team has helped to mitigate one of the most common and pervasive debates that exist among a lot of other languages.
While the language communities tend to drive coding conventions, there are always little idiosyncrasies in the way individuals write programs. Let's use one of the most common examples around—where to put the opening bracket.
Some programmers like it on the same line as the statement:
for (int i = 0; i < 100; i++) { // do something }
While others prefer it on the subsequent line:
for (int i = 0; i < 100; i++) { // do something }
These types of minor differences spark major, near-religious debates. The Gofmt tool helps alleviate this by allowing you to yield to Go's directive.
Now, Go bypasses this obvious source of contention at the compiler, by formatting your code similar to the latter example discussed earlier. The compiler will complain and all you'll get is a fatal error. But the other style choices have some flexibility, which are enforced when you use the tool to format.
Here, for example, is a piece of code in Go before go fmt
:
func Double(n int) int { if (n == 0) { return 0 } else { return n * 2 } }
Arbitrary whitespace can be the bane of a team's existence when it comes to sharing and reading code, particularly when every team member is not on the same IDE.
By running go fmt
, we clean this up, thereby translating our whitespace according to Go's conventions:
func Double(n int) int { if n == 0 { return 0 } else { return n * 2 } }
Long story short: always run go fmt
before shipping or pushing your code.
Beyond the absolute and the most trivial application—one that cannot even produce a Hello World output—you must have some imported package in a Go application.
To say Hello World, for example, we'd need some sort of a way to generate an output. Unlike in many other languages, even the core language library is accessible by a namespaced package. In Go, namespaces are handled by a repository endpoint URL, which is github.com/nkozyra/gotest, which can be opened directly on GitHub (or any other public location) for the review.
The go get tool easily handles packages hosted at the repositories, such as GitHub, Bitbucket, and Google Code (as well as a few others). You can also host your own projects, ideally a git project, elsewhere, although it might introduce some dependencies and sources for errors, which you'd probably like to avoid.
But what about the private repos? While go get is a wonderful tool, you'll find yourself looking at an error without some additional configuration, SSH agent forwarding, and so on.
You can work around this in a couple of ways, but one very simple method is to clone the repository locally, using your version control software directly.
You may have paused when you read about the way namespaces are defined and imported in a Go application. What happens if you're using version 1 of the application but would like to bring in version 2? In most cases, this has to be explicitly defined in the path of the import
. For example:
import ( "github.com/foo/foo-v1" )
versus:
import ( "github.com/foo/foo-v2" )
As you might imagine, this can be a particularly sticky aspect of the way Go handles the remote packages.
Unlike a lot of other package managers, go get is decentralized—that is, nobody maintains a canonical reference library of packages and versions. This can sometimes be a sore spot for new developers.
For the most part, packages are always imported via the go get
command, which reads the master branch of the remote repository. This means that maintaining multiple versions of a package at the same endpoint is, for the most part, impossible.
It's the utilization of the URL endpoints as namespaces that allows the decentralization, but it's also what provides a lack of internal support for versioning.
Your best bet as a developer is to treat every package as the most up-to-date version when you perform a go get
command. If you need a newer version, you can always follow whatever pattern the author has decided on, such as the preceding example.
As a creator of your own packages, make sure that you also adhere to this philosophy. Keeping your master branch HEAD as the most up-to-date will make sure your that the code fits with the conventions of other Go authors.
Handling private repositories
The go get tool easily handles packages hosted at the repositories, such as GitHub, Bitbucket, and Google Code (as well as a few others). You can also host your own projects, ideally a git project, elsewhere, although it might introduce some dependencies and sources for errors, which you'd probably like to avoid.
But what about the private repos? While go get is a wonderful tool, you'll find yourself looking at an error without some additional configuration, SSH agent forwarding, and so on.
You can work around this in a couple of ways, but one very simple method is to clone the repository locally, using your version control software directly.
You may have paused when you read about the way namespaces are defined and imported in a Go application. What happens if you're using version 1 of the application but would like to bring in version 2? In most cases, this has to be explicitly defined in the path of the import
. For example:
import ( "github.com/foo/foo-v1" )
versus:
import ( "github.com/foo/foo-v2" )
As you might imagine, this can be a particularly sticky aspect of the way Go handles the remote packages.
Unlike a lot of other package managers, go get is decentralized—that is, nobody maintains a canonical reference library of packages and versions. This can sometimes be a sore spot for new developers.
For the most part, packages are always imported via the go get
command, which reads the master branch of the remote repository. This means that maintaining multiple versions of a package at the same endpoint is, for the most part, impossible.
It's the utilization of the URL endpoints as namespaces that allows the decentralization, but it's also what provides a lack of internal support for versioning.
Your best bet as a developer is to treat every package as the most up-to-date version when you perform a go get
command. If you need a newer version, you can always follow whatever pattern the author has decided on, such as the preceding example.
As a creator of your own packages, make sure that you also adhere to this philosophy. Keeping your master branch HEAD as the most up-to-date will make sure your that the code fits with the conventions of other Go authors.
Dealing with versioning
You may have paused when you read about the way namespaces are defined and imported in a Go application. What happens if you're using version 1 of the application but would like to bring in version 2? In most cases, this has to be explicitly defined in the path of the import
. For example:
import ( "github.com/foo/foo-v1" )
versus:
import ( "github.com/foo/foo-v2" )
As you might imagine, this can be a particularly sticky aspect of the way Go handles the remote packages.
Unlike a lot of other package managers, go get is decentralized—that is, nobody maintains a canonical reference library of packages and versions. This can sometimes be a sore spot for new developers.
For the most part, packages are always imported via the go get
command, which reads the master branch of the remote repository. This means that maintaining multiple versions of a package at the same endpoint is, for the most part, impossible.
It's the utilization of the URL endpoints as namespaces that allows the decentralization, but it's also what provides a lack of internal support for versioning.
Your best bet as a developer is to treat every package as the most up-to-date version when you perform a go get
command. If you need a newer version, you can always follow whatever pattern the author has decided on, such as the preceding example.
As a creator of your own packages, make sure that you also adhere to this philosophy. Keeping your master branch HEAD as the most up-to-date will make sure your that the code fits with the conventions of other Go authors.
At the heart of all network communications in Go is the aptly-named net package, which contains subpackages not only for the very relevant HTTP operations, but also for other TCP/UDP servers, DNS, and IP tools.
In short, everything you need to create a robust server environment.
Of course, what we care about for the purpose of this book lies primarily in the net/http
package, but we'll look at a few other functions that utilize the rest of the package, such as a TCP connection, as well as WebSockets.
Let's quickly take a look at just performing that Hello World (or Web, in this case) example we have been talking about.
The following application serves as a static file at the location /static
, and a dynamic response
at the location /dynamic
:
package main import ( "fmt" "net/http" "time" ) const ( Port = ":8080" ) func serveDynamic(w http.ResponseWriter, r *http.Request) { response := "The time is now " + time.Now().String() fmt.Fprintln(w,response) }
Just as fmt.Println
will produce desired content at the console level, Fprintln
allows you to direct output to any writer. We'll talk a bit more about the writers in Chapter 2, Serving and Routing, but they represent a fundamental, flexible interface that is utilized in many Go applications, not just for the Web:
func serveStatic(w http.ResponseWriter, r *http.Request) { http.ServeFile(w, r, "static.html") }
Our serveStatic
method just serves one file, but it's trivial to allow it to serve any file directly and use Go as an old-school web server that serves only static content:
func main() { http.HandleFunc("/static",serveStatic) http.HandleFunc("/",serveDynamic) http.ListenAndServe(Port,nil) }
Feel free to choose the available port of your choice—higher ports will make it easier to bypass the built-in security functionality, particularly in Nix systems.
If we take the preceding example and visit the respective URLs—in this case the root at /
and a static page at /static
, we should see the intended output as shown:
At the root, /
, the output is as follows:
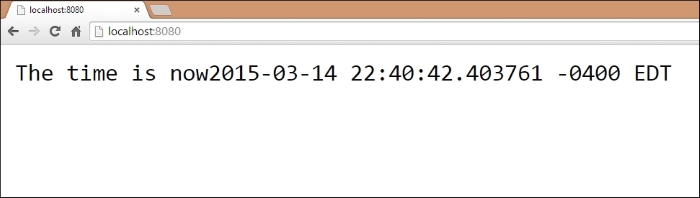
At /static
, the output is as follows:
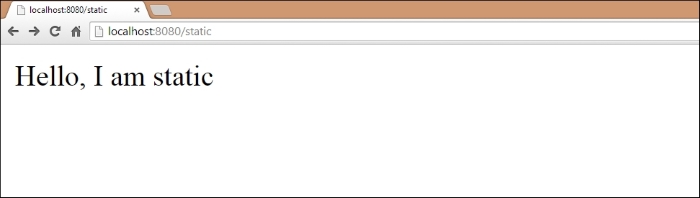
As you can see, producing a very simple output for the Web is, well, very simple in Go. The built-in package allows us to create a basic, yet inordinately fast site in Go with just a few lines of code using native packages.
This may not be very exciting, but before we can run, we must walk. Producing the preceding output introduces a few key concepts.
First, we've seen how net/http
directs requests using a URI or URL endpoint to helper functions, which must implement the http.ResponseWriter
and http.Request
methods. If they do not implement it, we get a very clear error on that end.
The following is an example that attempts to implement it in this manner:
func serveError() { fmt.Println("There's no way I'll work!") } func main() { http.HandleFunc("/static", serveStatic) http.HandleFunc("/", serveDynamic) http.HandleFunc("/error",serveError) http.ListenAndServe(Port, nil) }
The following screenshot shows the resulting error you'll get from Go:
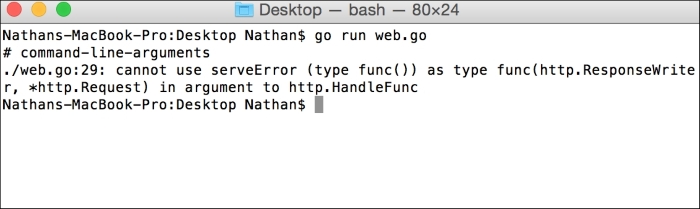
You can see that serveError
does not include the required parameters and thus results in a compilation error.
This chapter serves as an introduction to the most basic concepts of Go and producing for the Web in Go, but these points are critical foundational elements for being productive in the language and in the community.
We've looked at coding conventions and package design and organization, and we've produced our first program—the all-too-familiar Hello, World application—and accessed it via our localhost.
Obviously, we're a long way from a real, mature application for the Web, but the building blocks are essential to getting there.
In Chapter 2, Serving and Routing, we'll look at how to direct different requests to different application logic using the built-in routing functionality in Go's net/http
package, as well as a couple of third party router packages.