In the previous chapter, we discussed delegating application responsibility to networked services accessible by API and intra-process communication and handled by a message queue.
This approach mimics an emerging trend of breaking large monolithic applications into smaller chunks; thus, allowing developers to leverage dissonant languages, frameworks, and designs.
We listed a few upsides and downsides of this approach; while most of the upsides dealt with keeping the development agile and lean while preventing catastrophic and cascading errors that might bring down an entire application, a large downside is the fragility of each individual component. For example, if our e-mail microservice had bad code as a part of a large application, the error would make itself known quickly because it would almost assuredly have a direct and detectable impact on another component. But by isolating processes as part of microservices, we also isolate their state and status.
This is where the contents of this chapter come into play—the ability to test and log within a Go application is the strength of the language's design. By utilizing these in our application, it grows to include more microservices; due to which we can be in a better position to keep track of any issues with a cog in the system without imposing too much additional complexity to the overall application.
In this chapter we will cover the following topics:
- Introducing logging in Go
- Logging to IO
- Formatting your output
- Using panics and fatal errors
- Introducing testing in Go
Go comes with innumerable ways to display output to stdout
, most commonly the fmt
package's Print
and Println
. In fact, you can eschew the fmt
package entirely and just use print()
or println()
.
In mature applications, you're unlikely to see too many of these, because simply displaying an output without having the capability to store that somewhere for debugging or later analysis is rare and lacks much utility. Even if you're just outputting minor feedback to a user, it often makes sense to do so and keep the ability to save that to a file or elsewhere, this is where the log
package comes into play. Most of the examples in this book have used log.Println
in lieu of fmt.Println
for this very reason. It's trivial to make that change if, at some point, you choose to supplant stdout
with some other (or additional) io.Writer
.
So far we've been logging in to stdout
, but you can utilize any io.Writer
to ingest the log data. In fact, you can use multiple io.Writers
if you want the output to be routed to more than one place.
Most mature applications will write to more than one log file to delineate between the various types of messages that need to be retained.
The most common use case for this is found in web server. They typically keep an access.log
and an error.log
file to allow the analysis of all successful requests; however, they also maintain separate logging of different types of messages.
In the following example, we modify our logging concept to include errors as well as warnings:
package main import ( "log" "os" ) var ( Warn *log.Logger Error *log.Logger Notice *log.Logger ) func main() { warnFile, err := os.OpenFile("warnings.log", os.O_RDWR|os.O_APPEND, 0660) defer warnFile.Close() if err != nil { log.Fatal(err) } errorFile, err := os.OpenFile("error.log", os.O_RDWR|os.O_APPEND, 0660) defer errorFile.Close() if err != nil { log.Fatal(err) } Warn = log.New(warnFile, "WARNING: ", Log.LstdFlags ) Warn.Println("Messages written to a file called 'warnings.log' are likely to be ignored :(") Error = log.New(errorFile, "ERROR: ", log.Ldate|log.Ltime) Error.SetOutput(errorFile) Error.Println("Error messages, on the other hand, tend to catch attention!") }
We can take this approach to store all sorts of information. For example, if we wanted to store registration errors, we can create a specific registration error logger and allow a similar approach if we encounter an error in that process as shown:
res, err := database.Exec("INSERT INTO users SET user_name=?, user_guid=?, user_email=?, user_password=?", name, guid, email, passwordEnc) if err != nil { fmt.Fprintln(w, err.Error) RegError.Println("Could not complete registration:", err.Error) } else { http.Redirect(w, r, "/page/"+pageGUID, 301) }
Multiple loggers
Most mature applications will write to more than one log file to delineate between the various types of messages that need to be retained.
The most common use case for this is found in web server. They typically keep an access.log
and an error.log
file to allow the analysis of all successful requests; however, they also maintain separate logging of different types of messages.
In the following example, we modify our logging concept to include errors as well as warnings:
package main import ( "log" "os" ) var ( Warn *log.Logger Error *log.Logger Notice *log.Logger ) func main() { warnFile, err := os.OpenFile("warnings.log", os.O_RDWR|os.O_APPEND, 0660) defer warnFile.Close() if err != nil { log.Fatal(err) } errorFile, err := os.OpenFile("error.log", os.O_RDWR|os.O_APPEND, 0660) defer errorFile.Close() if err != nil { log.Fatal(err) } Warn = log.New(warnFile, "WARNING: ", Log.LstdFlags ) Warn.Println("Messages written to a file called 'warnings.log' are likely to be ignored :(") Error = log.New(errorFile, "ERROR: ", log.Ldate|log.Ltime) Error.SetOutput(errorFile) Error.Println("Error messages, on the other hand, tend to catch attention!") }
We can take this approach to store all sorts of information. For example, if we wanted to store registration errors, we can create a specific registration error logger and allow a similar approach if we encounter an error in that process as shown:
res, err := database.Exec("INSERT INTO users SET user_name=?, user_guid=?, user_email=?, user_password=?", name, guid, email, passwordEnc) if err != nil { fmt.Fprintln(w, err.Error) RegError.Println("Could not complete registration:", err.Error) } else { http.Redirect(w, r, "/page/"+pageGUID, 301) }
When instantiating a new Logger
, you can pass a few useful parameters and/or helper strings to help define and clarify the output. Each log entry can be prepended with a string, which can be helpful while reviewing multiple types of log entries. You can also define the type of date and time formatting that you would like on each entry.
To create a custom formatted log, just invoke the New()
function with an io.Writer
as shown:
package main import ( "log" "os" ) var ( Warn *log.Logger Error *log.Logger Notice *log.Logger ) func main() { warnFile, err := os.OpenFile("warnings.log", os.O_RDWR|os.O_APPEND, 0660) defer warnFile.Close() if err != nil { log.Fatal(err) } Warn = log.New(warnFile, "WARNING: ", log.Ldate|log.Ltime) Warn.Println("Messages written to a file called 'warnings.log' are likely to be ignored :(") log.Println("Done!") }
This not only allows us to utilize stdout
with our log.Println
function but also store more significant messages in a log file called warnings.log
. Using the os.O_RDWR|os.O_APPEND
constants allow us to write to the file and use an append file mode, which is useful for logging.
In addition to simply storing messages from your applications, you can create application panics and fatal errors that will prevent the application from continuing. This is critical for any use case where errors that do not halt execution lead to potential security issues, data loss, or any other unintended consequence. These types of mechanisms are generally relegated to the most critical of errors.
When to use a panic()
method is not always clear, but in practice this should be relegated to errors that are unrecoverable. An unrecoverable error typically means the one where state becomes ambiguous or cannot otherwise be guaranteed.
For example, operations on acquired database records that fail to return expected results from the database may be considered unrecoverable because future operations might occur on outdated or missing data.
In the following example, we can implement a panic where we can't create a new user; this is important so that we don't attempt to redirect or move forward with any further creation steps:
if err != nil { fmt.Fprintln(w, err.Error) RegError.Println("Could not complete registration:", err.Error) panic("Error with registration,") } else { http.Redirect(w, r, "/page/"+pageGUID, 301) }
Note that if you want to force this error, you can just make an intentional MySQL error in your query:
res, err := database.Exec("INSERT INTENTIONAL_ERROR INTO users SET user_name=?, user_guid=?, user_email=?, user_password=?", name, guid, email, passwordEnc)
When this error is triggered you will find this in your respective log file or stdout
:

In the preceding example, we utilize the panic as a hard stop, one that will prevent further execution that could lead to further errors and/or data inconsistency. If it need not be a hard stop, utilizing the recover()
function allows you to re-enter application flow once the problem has been addressed or mitigated.
Go comes packaged with a great deal of wonderful tools for making sure your code is clean, well-formatted, free of race conditions, and so on. From go vet
to go fmt
, many of the helper applications that you need to install separately in other languages come as a package with Go.
Testing is a critical step for software-development. Unit testing and test-driven development helps find bugs that aren't immediately apparent, especially to the developer. Often we're too close and too familiar with the application to make the types of usability mistakes that can invoke the otherwise undiscovered errors.
Go's testing package allows unit testing of actual functionality as well as making certain that all of the dependencies (network, file system locations) are available; testing in disparate environments allows you to discover these errors before users do.
If you're already utilizing unit tests, Go's implementation will be both familiar and pleasant to get started in:
package example func Square(x int) int { y := x * x return y }
This is saved as example.go
. Next, create another Go file that tests this square root functionality, with the following code:
package example import ( "testing" ) func TestSquare(t *testing.T) { if v := Square(4); v != 16 { t.Error("expected", 16, "got", v) } }
You can run this by entering the directory and simply typing go test -v
. As expected, this passes given our test input:

This example is obviously trivial, but to demonstrate what you will see if your tests fail, let's change our Square()
function as shown:
func Square(x int) int { y := x return y }
And again after running the test, we get:
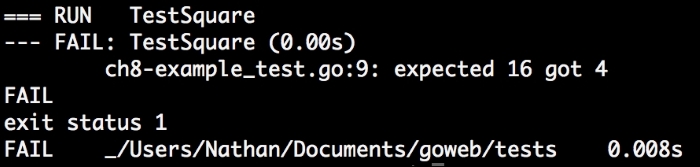
Running command-line tests against command-line applications is different than interacting with the Web. Our application being the one that includes standard HTML endpoints as well as API endpoints; testing it requires more nuance than the approach we used earlier.
Luckily, Go also includes a package for specifically testing the results of an HTTP application, net/http/httptest
.
Unlike the preceding example, httptest
lets us evaluate a number of pieces of metadata returned from our individual functions, which act as handlers in the HTTP version of unit tests.
So let's look at a simple way of evaluating what our HTTP server might be producing, by generating a quick endpoint that simply returns the day of the year.
To begin, we'll add another endpoint to our API. Lets separate this handler example into its own application to isolate its impact:
package main import ( "fmt" "net/http" "time" ) func testHandler(w http.ResponseWriter, r *http.Request) { t := time.Now() fmt.Fprintln(w, t.YearDay()) } func main() { http.HandleFunc("/test", testHandler) http.ListenAndServe(":8080", nil) }
This will simply return the day (1-366) of the year through the HTTP endpoint /test
. So how do we test this?
First, we need a new file specifically for testing. When it comes to how much test coverage you'll need to hit, which is often helpful to the developer or organization—ideally we'd want to hit every endpoint and method to get a fairly comprehensive coverage. For this example, we'll make sure that one of our API endpoints returns a proper status code and that a GET
request returns what we expect to see in the development:
package main import ( "io/ioutil" "net/http" "net/http/httptest" "testing" ) func TestHandler(t *testing.T) { res := httptest.NewRecorder() path := "http://localhost:4000/test" o, err := http.NewRequest("GET", path, nil) http.DefaultServeMux.ServeHTTP(res, req) response, err := ioutil.ReadAll(res.Body) if string(response) != "115" || err != nil { t.Errorf("Expected [], got %s", string(response)) } }
Now, we can implement this in our actual application by making certain that our endpoints pass (200) or fail (404) and return the text we expect them to return. We could also automate adding new content and validating it, and you should be equipped to take that on after these examples.
Given the fact that we have a hello-world endpoint, let's write a quick test that validates our response from the endpoint and have a look at how we can get a proper response in a test.go
file:
package main import ( "net/http" "net/http/httptest" "testing" ) func TestHelloWorld(t *testing.T) { req, err := http.NewRequest("GET", "/page/hello-world", nil) if err != nil { t.Fatal("Creating 'GET /page/hello-world' request failed!") } rec := httptest.NewRecorder() Router().ServeHTTP(rec, req) }
Here we can test that we're getting the status code we expect, which is not necessarily a trivial test despite its simplicity. In practice, we'd probably also create one that should fail and another test that checks to make sure that we get the HTTP response we expect. But this sets the stage for more complex test suites, such as sanity tests or deployment tests. For example, we might generate development-only pages that generate HTML content from templates and check the output to ensure our page access and our template parsing work as we expect.
Note
Read more about the testing with http and the httptest package at https://golang.org/pkg/net/http/httptest/
Simply building an application is not even half the battle and user-testing as a developer introduces a huge gap in testing strategy. Test coverage is a critical weapon when it comes to finding bugs, before they ever manifest to an end user.
Luckily, Go provides all the tools necessary to implement automated unit tests and the logging architecture necessary to support it.
In this chapter, we looked at both loggers and testing options. By producing multiple loggers for different messages, we were able separate warnings from errors brought about by internal application failures.
We then examined unit testing using the test and the httptest
packages to automatically check our application and keep it current by testing for potential breaking changes.
In Chapter 9, Security, we'll look at implementing security more thoroughly; from better TLS/SSL, to preventing injection and man-in-the-middle and cross-site request forgery attacks in our application.