Tuples can be consumed using a deconstruction declaration. This simply splits a Tuple into its individual parts and assigns these parts to new variables. This is called deconstruction, and it is not only reserved for Tuples.
Deconstruction
Getting ready
Remember when we used Tuples at the beginning of this chapter? Well we were using code similar to the following to get the values returned by the Tuple literal.
var (average, studentCount) = ch1.GetAverageAndCount(scores);
This was deconstructing the parts of the Tuple into the new variables average and studentCount. I do not, however, want to take a look at Tuples again. What I want to do is show how you can implement a deconstruction declaration on any type. To do this, all that we need to do is ensure that the type has a deconstructor method. We will modify our existing Student class to add a deconstructor.
How to do it...
- If you created the Student class earlier, you should have something similar to this in your code:
public class Student
{
public string Name { get; set; }
public string LastName { get; set; }
public List<int> CourseCodes { get; set; }
}
- To create a deconstructor, add a Deconstruct method to your Student class. You will notice that this is a void method that takes two out parameters (in this instance). We then just assign the values of Name and LastName to the out parameters.
public void Deconstruct(out string name, out string lastName)
{
name = Name;
lastName = LastName;
}
- Your modified Student class should now look as follows:
public class Student
{
public string Name { get; set; }
public string LastName { get; set; }
public List<int> CourseCodes { get; set; }
public void Deconstruct(out string name, out string lastName)
{
name = Name;
lastName = LastName;
}
}
- Consuming our Student class (just like we did with Tuples) can now be accomplished as follows:
Student student = new Student();
student.Name = "Dirk";
student.LastName = "Strauss";
var (FirstName, Surname) = student;
WriteLine($"The student name is {FirstName} {Surname}");
- Running the Console Application will display the deconstructed values returned from the Student class.
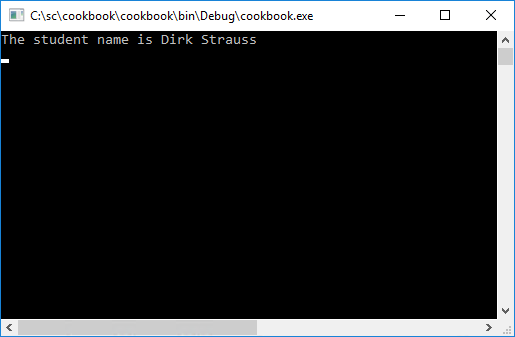
- Deconstructors can just as easily be used in extension methods. This is quite a nice way to extend the existing type to include a deconstruction declaration. To implement this, we need to remove the deconstructor from our Student class. You can just comment it out for now, but essentially this is what we are after:
public class Student
{
public string Name { get; set; }
public string LastName { get; set; }
public List<int> CourseCodes { get; set; }
}
- The Student class now does not contain a deconstructor. Head on over to the extension methods class and add the following extension method:
public static void Deconstruct(this Student student,
out string firstItem, out string secondItem)
{
firstItem = student.Name;
secondItem = student.LastName;
}
- The extension method acts on a Student type only. It follows the same basic implementation of the deconstructor created earlier in the Student class itself. Running the console application again, you will see the same result as before. The only difference is that the code is now using the extension method to deconstruct values in the Student class.
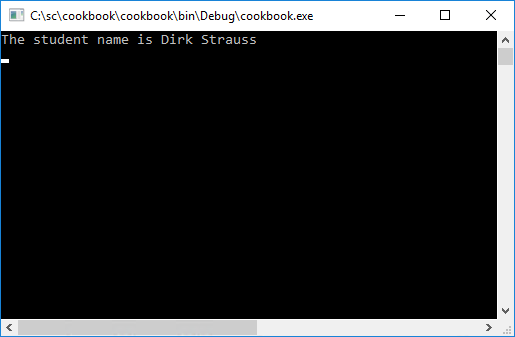
How it works...
In the code example, we set the student name and last name to specific values. This was just to illustrate the use of deconstruction. A more likely scenario would be to pass a student number to the Student class (in the constructor perhaps), as follows:
Student student = new Student(studentNumber);
The implementation within the Student class would then perform a database lookup using the student number passed through in the constructor. This will then return the student details. A more likely implementation of the Student class would probably look as follows:
public class Student
{
public Student(string studentNumber)
{
(Name, LastName) = GetStudentDetails(studentNumber);
}
public string Name { get; private set; }
public string LastName { get; private set; }
public List<int> CourseCodes { get; private set; }
public void Deconstruct(out string name, out string lastName)
{
name = Name;
lastName = LastName;
}
private (string name, string surname) GetStudentDetails(string studentNumber)
{
var detail = (n: "Dirk", s: "Strauss");
// Do something with student number to return the student details
return detail;
}
}
You will notice that the GetStudentDetails() method is just a dummy implementation. This is where the database lookup will start and the values will be returned from here. The code that calls the Student class now makes more sense. We call the Student class, pass it a student number, and deconstruct it to find the student's first name and surname.
Student student = new Student("S20323742");
var (FirstName, Surname) = student;
WriteLine($"The student name is {FirstName} {Surname}");