Traditionally, throw has been a statement in C#. As we know, because it is a statement and not an expression, we could not use it in certain places. Thanks to expression-bodied members, C# 7.0 introduced throw expressions. There isn't any difference in how an exception is thrown, only in where you can throw them from.
throw expressions
Getting ready
Throwing exceptions is nothing new. You have been doing it ever since you have been writing code. I will admit that throw expressions are a very welcome addition to C# and it's all thanks to expression-bodied members.
How to do it...
- To illustrate the use of a throw expression, create a method called GetNameLength() in the Chapter1 class. All it does is check to see if the length of a name is not zero. If it is, then the method will throw an exception right there in the expression.
public int GetNameLength(string firstName, string lastName)
{
return (firstName.Length + lastName.Length) > 0 ?
firstName.Length + lastName.Length : throw new
Exception("First name and last name is empty");
}
- To see the throw expression in action, create an instance of the Chapter1 class and call the GetNameLength() method. Pass it two blank strings as parameters.
try
{
Chapter1 ch1 = new Chapter1();
int nameLength = ch1.GetNameLength("", "");
}
catch (Exception ex)
{
WriteLine(ex.Message);
}
- Running your console application will then return the exception message as the output.
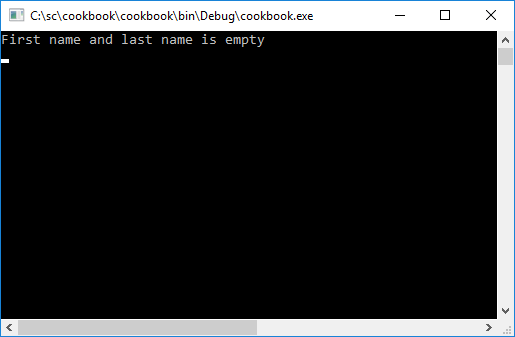
How it works...
Being able to use throw expressions makes your code easier to write and easier to read. The new features in C# 7.0 build on top of the fantastic foundation laid down by C# 6.0.