Each development team is unique. Teams have their own way of doing business. In many organizations, there are one-off tasks that need to be done periodically, for example at the end of each year.
This recipe details a script that checks for the last successful run of any Job, and if the year is different to the current year, then a warning is set at the beginning of the Jobs description. Thus, hinting to you it is time to perform some action, such as archiving and then deleting. You can, of course, programmatically do the archiving. However, for high value actions, it is worth forcing interceding, letting the Groovy scripts focus your attention.
Within the Manage Jenkins page, click on the Script console link, and run the following script:
import hudson.model.Run; import java.text.DateFormat; def warning='<font color=\'red\'>[ARCHIVE]</font> ' def now=new Date() for (job in hudson.model.Hudson.instance.items) { println "\nName: ${job.name}" Run lastSuccessfulBuild = job.getLastSuccessfulBuild() if (lastSuccessfulBuild != null) { def time = lastSuccessfulBuild.getTimestamp().getTime() if (now.year.equals(time.year)){ println("Project has same year as build"); }else { if (job.description.startsWith(warning)){ println("Description has already been changed"); }else{ job.setDescription("${warning}${job.description}") } } } }
Any project that had its last successful build in another year than this will have the word [ARCHIVE]
in red, added at the start of its description.
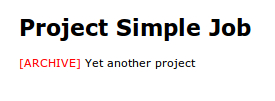
Reviewing the code listing:
A warning string is defined, and the current date is stored in now. Each Job in Jenkins is programmatically iterated through the for
statement.
Jenkins has a class to store information about the running of builds. The runtime information is retrieved through job.getLastSuccessfulBuild()
, and is stored in the lastSuccessfulBuild
instance. If no successful build has occurred, then lastSuccessfulBuild
is set to null
, otherwise it has the runtime information.
The time of the last successful build is retrieved, and then stored in the time
instance through lastSuccessfulBuild.getTimestamp().getTime()
.
The current year is compared with the year of the last successful build, and if they are different and the warning string has not already been added to the front of the Job description, then the description is updated.
Tip
Javadoc
You will find the Job API mentioned at http://javadoc.jenkins-ci.org/hudson/model/Job.html and the Run information at http://javadoc.jenkins-ci.org/hudson/model/Run.html.
Before writing your own code, you should review what already exists. With 300 plugins, Jenkins has a large, freely-available, and openly licensed example code base. Although in this case the standard API was used, it is well worth reviewing the plugin code base. In this example, you will find part of the code re-used from the lastsuccessversioncolumn
plugin (https://github.com/jenkinsci/lastsuccessversioncolumn-plugin/blob/master/src/main/java/hudson/plugins/lastsuccessversioncolumn/LastSuccessVersionColumn.java).