Java EE 7 supports transactions, which are a group of important operations, commands, or behaviors that are executed as a unit of work. A true transaction must follow the ACID principles: Atomic, Consistent, Isolated, and Durable. In Java EE 7, behaviors involved in a transaction may be synchronous or asynchronous, and they involve persisting entities to a database with JPA, sending or receiving messages using JMS, sending content over the network, invoking EJBs, and can even include other external systems.
These are the ACID principles of transactions:
Java EE 7 meets the requirements for ACID transactions. Enterprise Java Beans are transactional by default. CDI managed beans can be uplifted into ACID transactions with the application of the annotation @javax.transaction.Transactional
.
Java Transaction API (JTA) is the cornerstone of all Java EE transactions. JTA looks after local and distributed transactions. Non-JTA transactions are a matter for standalone Java SE applications. The popular confusion abounds when developers are configuring persistence unit facilities for the first time (the persistence.xml
files). The XML element jta-data-source
is used in Java EE and non-jta-data-source
for Java SE.
Relational databases generally have an internal component called a Two-Phase Commit Transaction Manager (2PC) to ensure ACID principles. The two-phase commit is a protocol, which is based on resource managers, veto authorities, and acknowledgements, and takes place at the end of a transaction. The JTA specification refers to a XA (Extended Architecture) transaction manager and its participant resources.
The first phase in 2PC is called the prepare command. It consists of a transaction manager communicating with resources through the protocol with the notification that a commit is about to be issued. A resource has a chance to declare whether it can fulfill committing the transaction or not. If it can, the resource prepares the work to save the data and acknowledges with a prepared response, otherwise it vetoes the protocol, which effectively rolls the entire transaction back.
The second phase in 2PC only proceeds once all resources have given their unanimous consent. The transaction manager then sends a commit command to the resources. Each resource applies the changes to the database. This is usually a short error-free operation and afterwards the resource acknowledges with a final commit-done command to the transaction manager.
Sometimes issues will occur in 2PC transactions, and usually they occur in the second phase of commit. One or more resources will actually fail to save the data changes to the database leaving it in an inconsistent state. Heuristic failures are the result of network outages, power failures, hardware failures such as disk I/O error, or other extremes that sometimes go wrong in any data center anywhere in the world. The JTA manager will raise an unwelcome javax.transaction.HeuristicMixedException
. At the worst case scenario, you might even achieve a HeuristicRolledBackException
. Heuristics are not recovered automatically and sadly they necessitate a replay of a database server's transaction log if and when these vendor features are enabled on production! The following diagram gives an example of the sequence of actions taking place in the transaction activity:
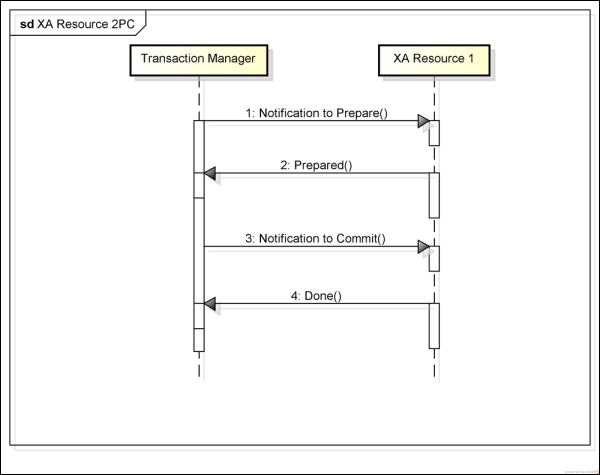
A transaction is deemed local when it is working with resources, which are co-located within a running JVM and the application server. A local transaction involves no distributed resources.
A local transaction can be either non-XA or XA transaction on the Java EE 7 platform. A non-XA local transaction takes place with standard JDBC or JPA environment without involvement of a 2PC XA transaction manager. A XA local transaction is one that takes place within the remit of a 2PC XA transaction manager.
Some application servers such as JBoss WildFly are able to optimize a single non-XA local transaction so that they participate in a set of XA resources in a 2PC transaction. The optimization is called last non-XA resource commit.
A transaction is deemed distributed when it is executed across different JVMs and operating system process boundaries, and across the network. Because of the JTA specification, the EJB and CDI containers can participate in distributed transaction with other containers and third-party XA resources like database servers. A distributed transaction can span a cluster of application servers running on JVMs that may or may not be on the same machine or co-located across hardware racks in a remote data center.