This recipe explains how to handle the faults thrown from a synchronous BPEL process. A BPEL process uses the <throw>
activity in case of exceptional situations. It gives the client feedback on what went wrong with the BPEL process processing. In a scenario where the client is expecting the response message and does not capture the faults thrown from the BPEL process, we can define the inline fault in the BPEL process reply activity.
We modified the synchronous BPEL process to throw an exception when the input parameter says FAULT
. With the check_fault
condition, we check if the input parameter contains the word FAULT
. The modified BPEL process is shown in the following screenshot:
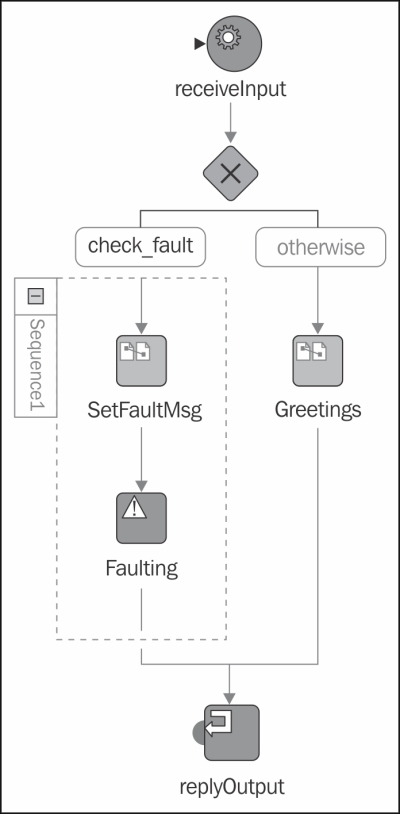
We also need to define the fault structure in the XSD schema (HelloWorldProcess.xsd
) as shown in the following code:
<element name = "fault"> <complexType> <sequence> <element name = "msg" type = "string"/> </sequence> </complexType> </element>
We define the element fault
, which contains the element msg
of the type string
. We then define the fault message in the WSDL document as follows:
<wsdl:message name = "ProcessFaultMessage"> <wsdl:part name = "message" element = "client:fault"/> </wsdl:message>
We also specify the fault in the <portType>
and <binding>
elements of the WSDL document (HelloWorldProcess.wsdl
). In the following code, we omit the <binding>
element definition:
<wsdl:portType name = "HelloWorldProcess"> <wsdl:operation name = "process"> <wsdl:input message = "client:HelloWorldProcessRequestMessage"/> <wsdl:output message = "client:HelloWorldProcessResponseMessage"/> <wsdl:fault name = "fault" message = "client:ProcessFaultMessage"/> </wsdl:operation> </wsdl:portType>
We create the client proxy the same way we created it in the Calling a synchronous BPEL process from Java recipe. We now see the additional class ProcessFaultMessage.java
. We use this class when the fault occurs in the BPEL process. The class is annotated with the @WebFault
annotation, which indicates the service specific exception class as follows:
@WebFault(faultBean = "org.packt.bpel.sync.gen.Fault", targetNamespace = "http://xmlns.oracle.com/bpel_101_HelloWorld_jws/bpel_101_HelloWorld/HelloWorldProcess", name = "fault") public class ProcessFaultMessage extends Exception
The faultBean
attribute defines the Java class that will transform the fault from XML to Java. Additionally, the namespace of the BPEL process is also defined.
We also need to modify the client proxy main class HelloWorldProcess_ptClient.java
. Since it is possible that the BPEL process throws a fault, we must prepare our client code in advance for such a situation.
First, we create the input and output variables as follows:
String input = "FAULT"; String output = "";
Tip
We changed the code for calling the BPEL process because it requests to be in the
try
/catch
block.try { output = helloWorldProcess.process(input); } catch (ProcessFaultMessage e) { System.out.println( e.getFaultInfo().getMsg()); } System.out.println("Business process returned:" + output);
We run the client and observer BPEL process execution in the Oracle Enterprise Manager Console as shown in the following screenshot:
The output of the console in JDeveloper contains the following messages:
Error while processing input parameter Business process returned: Process exited with exit code 0
The business faults, as opposed to the runtime faults, are thrown by the applications when a problem with processing information occurs. Various situations can cause the BPEL process to throw a fault. The BPEL process might interact with web services and web service itself may throw a fault. Consequently, the BPEL process must react on the fault thrown by web service. When an exceptional situation occurs in the BPEL process, the fault is propagated to the client. As we can see, the newly created class ProcessFaultMessage.java
extends the Exception
class. We see that the BPEL faults correspond to the Exception
class in Java with extensions. The BPEL process also provides the ability to define the compensation handlers. With the compensation handlers, it is possible to undo the actions that were executed during the BPEL process execution. We can consider the compensation handler as a block of code containing the activities performing the compensation tasks.
The BPEL specification defines two types of faults. In this, we meet the BPEL process fault; however, there also exists a set of standard faults.
Note
A list of standard faults can be accessed at the following URL: http://docs.oasis-open.org/wsbpel/2.0/wsbpel-v2.0.pdf (see Appendix A, Standard Faults).
The BPEL standard faults are thrown if the BPEL server encounters some conditions in the runtime environment that do not correspond to the specifications. This category also includes situations where the variables might not be initialized if transformation does not find the XSLT file or if some problems occur on the network.
Tip
When we want to add additional information to the already existing fault in the BPEL process, we define new fault message with additional information in the WSDL document of the BPEL process. We then model the fault handling within the fault handler (the catch
or catchall
activity). We specify the fault condition that will be caught by the fault handler. Inside the fault handler, we use the rethrow
activity that throws the fault, which will give the client a better insight into the problem that occurred in the BPEL process.