Techniques that repeat can be classified as patterns; you probably already have a bunch of patterns in your own code that you might classify even as your own patterns. Some of these become popular outside the realms of one developer's head and are promoted beyond just this one guy. A pattern is a well-understood solution to a particular problem. They are identified rather than created. That is, they emerge and are abstracted from solutions to real-world problems rather than being imposed on a problem from the outside. It's also a common vocabulary that allows developers to communicate more efficiently.
Note
A popular book that aims to gather some of these patterns is Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, Addison-Wesley Professional.
You can find a copy at http://www.amazon.com/Design-Patterns-Elements-Reusable-Object-Oriented/dp/0201633612.
We will be using different patterns throughout this book, so it's important to understand what they are, the reasons behind them, and how they are applied successfully. The following sections will give you a short summary of the patterns being referred to and used.
Interestingly enough, most of the patterns we have started applying have been around for quite a while. The Model View Controller (MVC) pattern is a great example of this.
Note
MVC was first introduced by a fellow Norwegian national called Dr. Trygve Reenskaug in 1973 in a paper called Administrative Control in the Shipyard (http://martinfowler.com/eaaDev/uiArchs.html). Since then, it has been applied successfully in a variety of frameworks and platforms. With the introduction of Ruby on Rails in 2005, I would argue the focus on MVC really started to get traction in the modern web development sphere. When Microsoft published ASP.NET MVC at the end of 2007, they helped gain focus in the .NET community as well.
The purpose of MVC is to decouple the elements in the frontend and create a better isolated focus on these different concerns. Basically, what one has is a controller that governs the actions that are allowed to be performed for a particular feature of your application. The actions can return a result in the form of either data or concrete new views to navigate to. The controller is responsible for holding and providing any state to the views through the actions it exposes. By state, we often think of the model and often the data comes from a database, either directly exposed or adapted into a view-specific model that suits the view better than the raw data from the database. The relationship between model, controller, view, and the user is well summarized in the following diagram:
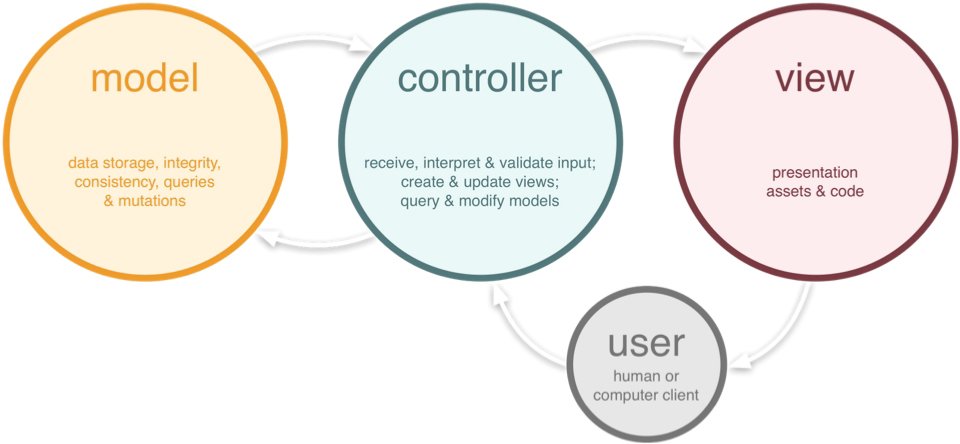
A representation of how the artifacts make up MVC (don't forget there is a user that will interact with all of these artifacts)
With this approach, you separate out the presentation aspect of the business logic into the controller. The controller then has a relationship with other subsystems that knows the other aspects of the business logic in a better manner, allowing the controller to only focus on the logic that is specific to the presentation and not on any concrete business logic but more on the presentation aspect of any business logic. This decouples it from the underlying subsystem and thus more specialized. The view now has to concern itself with only view-related things, which are typically HTML and CSS for web applications. The model, either a concrete model from the database or adapted for the view, is fetched from whatever data source you have.
Extending on the promise of decoupling in the frontend, we get something called Model View ViewModel (short for MVVM).
Note
For more information, visit http://www.codeproject.com/Articles/100175/Model-View-ViewModel-MVVM-Explained.
This is a design pattern for the frontend based largely on MVC, but it takes it a bit further in terms of decoupling. From this, Microsoft created a specialization that happened to be MVVM.
Note
MVVM was presented by Martin Fowler in 2004 to what he referred to as the Presentation Model (which you can access at http://martinfowler.com/eaaDev/PresentationModel.html).
The ViewModel is a key player in this that holds the state and behavior needed for your feature to be able to do its job, without it knowing about the view. The view will then be observing the ViewModel for any changes it might get and utilize any behaviors it exposes. In the ViewModel, we keep the state, and as with MVC, the state is in the form of a model that could be a direct model coming from your data source or an adapted model more fine-tuned for the purpose of the frontend.
The additional decoupling, which this model represents, lies within the fact that the ViewModel has no clue to any view, and in fact should be blissfully unaware that it is being used in a view. This makes the code even more focused and it opens an opportunity of being able to swap out the view at any given time or even reuse the same ViewModel with its logic and state for second view.
The relationship between the model, view, viewmodel, and the user is summarized in the following diagram:
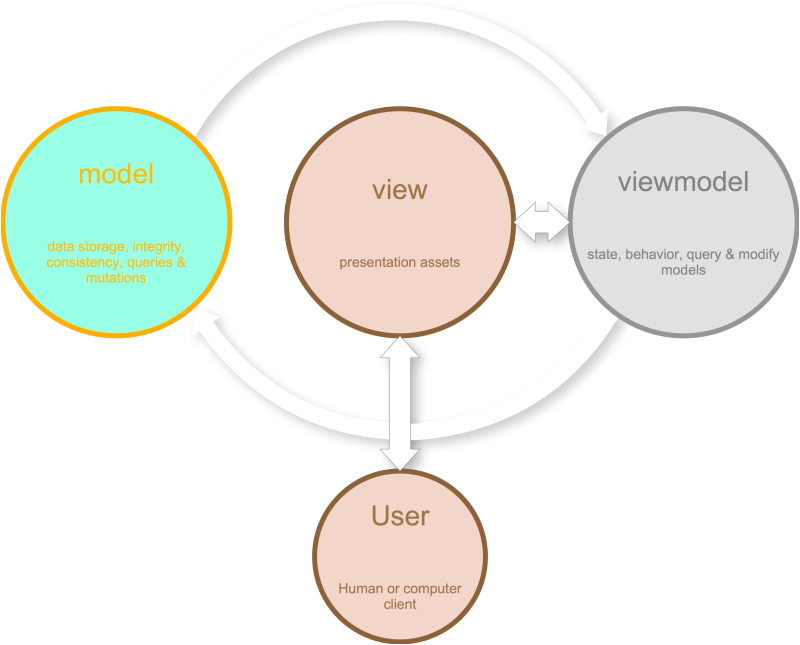
The artefacts that make up MVVM. As a reminder, don't forget that the user interacts with these artifacts through the view.