The three most basic parts of the Java ecosystem are the Java Virtual Machine (JVM), the Java Runtime Environment (JRE), and the Java Development Kit (JDK), which are stock parts that are supplied by Java implementations.
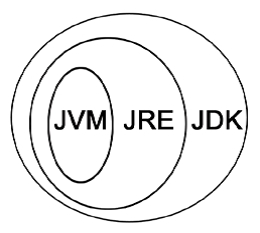
Figure 1.1: A representation of the Java ecosystem
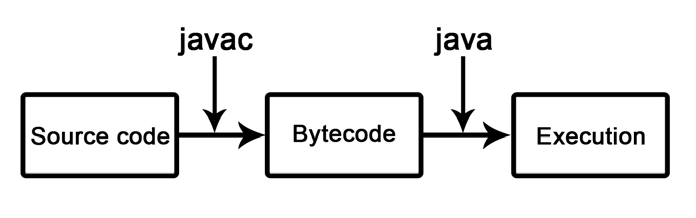
Every Java program runs under the control of a JVM. Every time you run a Java program, an instance of JVM is created. It provides security and isolation for the Java program that is running. It prevents the running of the code from clashing with other programs within the system. It works like a non-strict sandbox, making it safe to serve resources, even in hostile environments such as the internet, but allowing interoperability with the computer on which it runs. In simpler terms, JVM acts as a computer inside a computer, which is meant specifically for running Java programs.
Up in the hierarchy of stock Java technologies is the JRE. The JRE is a collection of programs that contains the JVM and also many libraries/class files that are needed for the execution of programs on the JVM (via the java command). It includes all the base Java classes (the runtime) as well as the libraries for interaction with the host system (such as font management, communication with the graphical system, the ability to play sounds, and plugins for the execution of Java applets in the browser) and utilities (such as the Nashorn JavaScript interpreter and the keytool cryptographic manipulation tool). As stated before, the JRE includes the JVM.
At the top layer of stock Java technologies is the JDK. The JDK contains all the programs that are needed to develop Java programs, and it's most important part is the Java Compiler (javac). The JDK also includes many auxiliary tools such as a Java disassembler (javap), a utility to create packages of Java applications (jar), system to generate documentation from source code (javadoc), among many other utilities. The JDK is a superset of the JRE, meaning that if you have the JDK, then you also have the JRE (and the JVM).
But those three parts are not the entirety of Java. The ecosystem of Java includes a very large participation of the community, which is one of the reasons for the popularity of the platform.
Note
Research into the most popular Java libraries that are used by the top Java projects on GitHub (according to research that has been repeated in 2016 and 2017) showed that JUnit, Mockito, Google's Guava, logging libraries (log4j, sl4j), and all of Apache Commons (Commons IO, Commons Lang, Commons Math, and so on), marked their presence, together with libraries to connect to databases, libraries for data analysis and machine learning, distributed computing, and almost anything else that you can imagine. In other words, for almost any use that you want to write programs to, there are high chances of an existing library of tools to help you with your task.
Besides the numerous libraries that extend the functionality of the stock distributions of Java, there is a myriad of tools to automate builds (for example, Apache Ant, Apache Maven, and Gradle), automate tests, distribution and continuous integration/delivery programs (for example, Jenkins and Apache Continuum), and much, much more.