-
Book Overview & Buying
-
Table Of Contents
-
Feedback & Rating
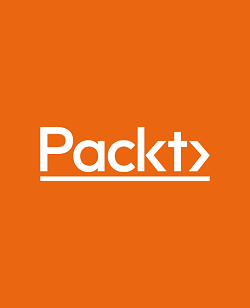
Numpy Beginner's Guide (Update)
By :
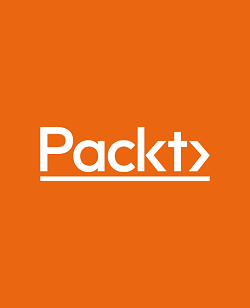
Numpy Beginner's Guide (Update)
By:
Overview of this book
This book is for the scientists, engineers, programmers, or analysts looking for a high-quality, open source mathematical library. Knowledge of Python is assumed. Also, some affinity, or at least interest, in mathematics and statistics is required. However, I have provided brief explanations and pointers to learning resources.
Table of Contents (16 chapters)
Preface
In Progress
| 0 / 13 sections completed |
0%
1. NumPy Quick Start
In Progress
| 0 / 32 sections completed |
0%
2. Beginning with NumPy Fundamentals
In Progress
| 0 / 11 sections completed |
0%
3. Getting Familiar with Commonly Used Functions
In Progress
| 0 / 37 sections completed |
0%
4. Convenience Functions for Your Convenience
In Progress
| 0 / 14 sections completed |
0%
5. Working with Matrices and ufuncs
In Progress
| 0 / 26 sections completed |
0%
6. Moving Further with NumPy Modules
In Progress
| 0 / 28 sections completed |
0%
7. Peeking into Special Routines
In Progress
| 0 / 37 sections completed |
0%
8. Assuring Quality with Testing
In Progress
| 0 / 26 sections completed |
0%
9. Plotting with matplotlib
In Progress
| 0 / 26 sections completed |
0%
10. When NumPy Is Not Enough – SciPy and Beyond
In Progress
| 0 / 22 sections completed |
0%
11. Playing with Pygame
In Progress
| 0 / 18 sections completed |
0%
A. Pop Quiz Answers
In Progress
| 0 / 10 sections completed |
0%
B. Additional Online Resources
In Progress
| 0 / 3 sections completed |
0%
C. NumPy Functions' References
In Progress
| 0 / 1 sections completed |
0%
Index
In Progress
| 0 / 1 sections completed |
0%
Customer Reviews