Running quick commands through the interactive shell is fun. It comes in handy when you have a quick hypothesis that you want to test out or when you want to check whether a specific method exists for some data type. However, you can't really write a full-fledged program through the interactive shell.
Python allows you to run your instructions from a saved file. A file containing Python instructions is called a module. A script is a module that can be run. Anything you can run on the interactive shell can be written and ran as a Python script.
By convention, Python scripts should have the file extension .py. The filename should be a valid filename, as defined by your operating system.
In this exercise, we will create a script that displays Hello five times in a single line:
Open your text editor.
Create a file called test1.py and insert the following code:
print("---------------------------------") print("Hello " * 5) print("---------------------------------")
Save it to your working directory.
Open your terminal, change into the directory where the file is saved, and run the following command: python test1.py. You should see the following output:
Figure 1.2: Creating and running a script
Python opens the file and executes each instruction, line by line. First, it runs the call to print on the first line and prints out a series of dashes. It then calls the second print, which prints our message five times, hence the * 5 bit. This can be any value you want and is basically a shorthand way of saying, "repeat that string of characters an n number of times", n being 5 in our case. For example, if you change that 5 to 100, it'll print Hello 100 times, as shown in the following code:
print("---------------------------------") print("Hello " * 100) print("---------------------------------")
This will be the output:
Figure 1.3: Creating and running the script with modified values
Finally, the last line is executed, just like our first, and prints out dashes. This execution is done in a blocking manner, so each line is executed after the previous line has completed running.
As with the interactive shell, putting in invalid instructions also causes an error. Make the following changes to your file and run it:
print("---------------------------------") print(invalid instruction) print("---------------------------------")
You should see an error. This output is called a stack trace. It tells us useful things such as where the error happened, what kind of error it was, and what other calls were triggered along the way when we ran our command. Stack traces should be read from bottom to top. Another name for a stack trace is a traceback:
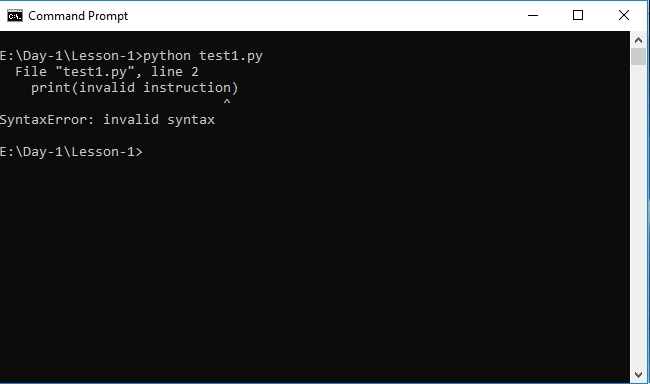
Figure 1.4: Running the file with invalid syntax
The last line tells us what kind of error was raised, that is, a SyntaxError, meaning that our instructions were invalid. The line above it logs out the source line that caused the error, and the first line references our test1.py module where the line is. You'll be seeing different types of errors as you go through this book, and we'll have an in-depth look at errors and exception handling in one of the later chapters. For now, it is important that we understand how to read a stack trace and identify what is causing the error, and then act accordingly to fix it.
To make a script more dynamic, you can have the user provide arguments to it when calling it:
Create a new file called test2.py in the same directory that we created test1.py in. Then, add the following code:
import sys print("This argument was passed to the script:", sys.argv[1])
Save and run the script as usual, passing it an argument, as illustrated here:
python test2.py foobar
The output should be as follows:
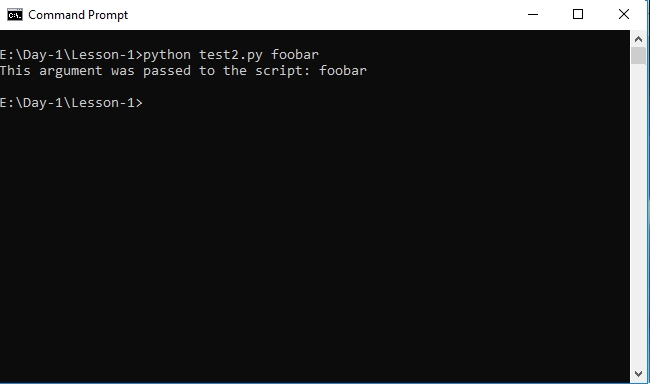
Figure 1.5: Passing arguments to the script
We have some new syntax in this script. We won't go over all of it in detail in this chapter, but for the purposes of this explanation, import sys imports the sys module that's built into Python into our module. This module provides access to Python interpreter functions. For our purposes, we're using it to read command-line arguments that have been passed to the interpreter when invoking our script.
When we call sys.argv[1], we're asking for the first argument that's been passed when running the script. Generally, you can pass as many arguments as you like by separating each argument with a blank space.
In this activity, we will create a name card generator script that, when called with a first name and last name, will generate a name card with the names.
The steps are as follows:
Open your editor and create a script named activity.py.
Use two print statements to print the First name and Last name. Also, use these print statements to print 20 underscores as borders above and below the names.
Two parameters should be passed with the script: one for the first name and the second for the last name.
Run the script by passing two string arguments.
Your output should be similar to the following:
Figure 1.6: Running simple scripts
Note
Python comes with its own IDE (Integrated Development Environment) known as IDLE. IDLE comes with an editor and interactive shell that supports syntax highlighting, a debugger, and a handful of other practical features. Since it comes with your Python installation, it's ready to use immediately and can help improve your productivity as it offers a larger set of capabilities compared to a bare editor and shell. More information about it can be found on the Python website at https://docs.python.org/3/library/idle.html.