NAV includes a full set of software development tools. All NAV development tools are accessed through the C/SIDE Integrated Development Environment. This environment and its full complement of tools are generally just referred to as C/SIDE. C/SIDE includes the C/AL compiler. All NAV programming uses C/AL. No NAV development can be done without using C/SIDE.
The C/SIDE Integrated Development Environment is referred to as the Object Designer within NAV. It is accessed through the Tools | Object Designer menu option as shown in the following screenshot:
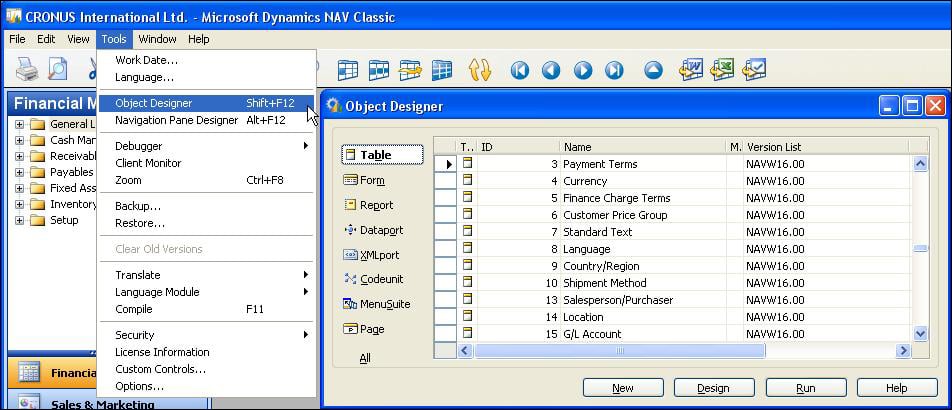
The language in which NAV is coded is C/AL. A sample of some C/AL code is shown as follows. C/AL syntax has considerable similarity to Pascal. As with any programming language, readability is enhanced by careful programmer attention to structure, logical variable naming, process flow consistent with that of the code in the base product and good documentation both inside and outside of the code.
IF "No." = '' THEN BEGIN SalesSetup.GET; SalesSetup.TESTFIELD("Customer Nos."); NoSeriesMgt.InitSeries(SalesSetup."Customer Nos.",xRec."No. Series",0D,"No.","No. Series"); END; IF "Invoice Disc. Code" = '' THEN "Invoice Disc. Code" := "No."; IF NOT InsertFromContact THEN UpdateContFromCust.OnInsert(Rec); DimMgt.UpdateDefaultDim( DATABASE::Customer,"No.", "Global Dimension 1 Code","Global Dimension 2 Code");
A large portion of the NAV system is defined by tabular entries, properties, and references. This doesn't reduce the requirement for the developer to understand the code, but it does allow some very significant applications development work to be done on a more of a point-and-choose or fill-in-the-blank approach than the traditional "grind it out" approach to coding. As we may mention more than once in this book, NAV gives developers the ability to focus on design rather than code. Much of the time, the effort required to create a new NAV application or modification will be heavily weighted on the side of design time, rather than technical development time. This long term goal for systems development tools has always been true for NAV. As the tools mature, NAV development continues to be more and more heavily weighted to design time rather than coding time.
The following screenshot shows a list of Object Designer tool icons. These Object Designer icons are shown isolated in the screenshot and then described briefly in the following table. Some of these icons apply to actions that are only for objects which run in the Classic Client, some are for objects for both clients. Where appropriate, the terminology in these descriptions will be explained later in this book. Additional information is available in the C/SIDE Help files and the Microsoft NAV documentation.
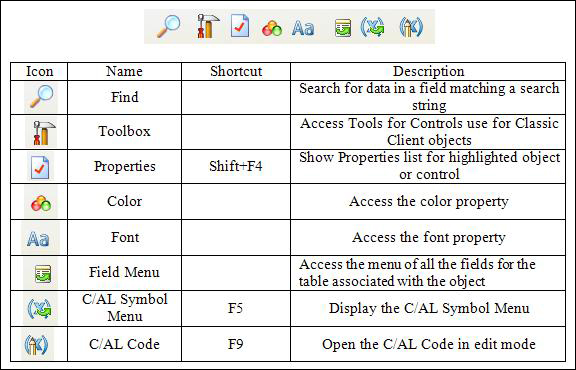
The following table lists the specific development tools used for each object type. Also, as shown in this table, some objects are limited to being used in the Classic Client, some are limited to being used in the RTC environment, some are used in both, and some are interpreted differently depending on the environment in which they are invoked.
Object Type |
Design Tool |
User Interface |
Comments |
---|---|---|---|
Table |
Table Designer |
Classic and RTC | |
Form |
Form Designer |
Classic | |
Page |
Page Designer |
RTC | |
Report |
Report Designer |
Classic (and RTC) |
If a report is run from the RTC but doesn't have an RTC compatible layout, NAV will run it under a temporary instance of the Classic Client |
Report Designer (data definition) + Visual Studio (user interface) |
RTC | ||
Dataport |
Dataport Designer |
Classic | |
XMLPort |
XMLport Designer |
Classic and RTC |
For the RTC, Dataport functionality is handled through appropriately defined XMLports |
Codeunit |
IDE code editor for the C/AL language |
Classic and RTC | |
MenuSuite |
Navigation Pane Designer |
Classic and RTC |
Much of the navigation in the RTC is done via Role Center pages rather than menus |
Let's take a look at the following:
Database: NAV has two physical database options. One is the C/SIDE Database Server; the other is the Microsoft SQL Server. The C/SIDE Database Server, formerly known as the "Native" database, only supports the two-tier client. The NAV 2009 three-tier functionality is only compatible with a SQL Server implementation. You won't be surprised to know that one or two product versions in the future, the only database option will be SQL Server.
At the basic application or code design levels, you don't care which database is being used. For sophisticated or high data volume applications you care a great deal about the underlying database strengths, weaknesses and features.
Properties: These are the attributes of the element (for example object, data field, or control) that define some aspect of its behavior or use. For example, attributes such as display length, font type or size, and if the elements are either editable or viewable.
Fields: These are the individual data items, defined either in a table or in the working storage of an object.
Records: These are groups of fields (data items) that are handled as a unit in most Input/Output operations. The table data consists of rows of records with columns consisting of fields.
Controls: These are containers for constants and data. A control corresponds to a User Interface element on a form/page or a report. The visible displays in reports and forms consist primarily of controls.
Triggers: The generic definition is a mechanism that initiates (fires) an action when an event occurs and is communicated to the application object. A trigger is either empty or contains code that is executed when the associated event fires the trigger. Each object type has its own set of predefined triggers. The event trigger name begins with the word "On" such as
OnInsert, OnOpenPage
, andOnNextRecord
. NAV triggers have some similarities to those in SQL, but they are not the same. NAV triggers are locations within the various objects where a developer can place comments or C/AL code.When you look at the C/AL code of an object in its Designer, the following have a physical resemblance to the NAV event based triggers:
Documentation which can contain comments only, no executable code. Every object type except MenuSuite has a single Documentation section at the beginning.
Functions which can be defined by the developer. They represent callable routines that can be accessed from other C/AL code either inside or outside the object where the called function resides. Many functions are provided as part of the standard product. As a developer, you may add your own custom functions as needed.
Object numbers and field numbers: The object numbers from 1 (one) to 50,000 and in the 99,000,000 (99 million) range are reserved for use by NAV as part of the base product. Objects in this number range can be modified or deleted, but not created with a developer's license. Field numbers are often assigned in ranges matching the related object numbers (that is starting with 1 for fields relating to objects numbered 1 to 50,000, starting with 99,000,000 for fields in objects in the 99,000,000 and up number range). Object and field numbers from 50,001 to 99,999 are generally available to the rest of us for assignment as part of customizations developed in the field using a normal development license. But object numbers from 90,000 to 99,999 should not be used for permanent objects as those numbers are often used in training materials. Microsoft allocates other ranges of object and field numbers to Independent Software Vendor (ISV) developers for their add-on enhancements. Some of these (in the 14,000,000 range in North America, other ranges for other geographic regions) can be accessed, modified, or deleted, but not created using a normal development license. Others (such as in the 37,000,000 range) can be executed, but not viewed or modified with a typical development license. The following table summarizes the content as:
Object Number range
Usage
1 9,999
Base-application objects
10,000 49,999
Country-specific objects
50,000 99,999
Customer-specific objects
100,000 98,999,999
Partner-created objects
Above 98,999,999
Microsoft territory
Work Date: This is a date controlled by the operator that is used as the default date for many transaction entries. The System Date is the date recognized by Windows. The Work Date that can be adjusted at any time by the user, is specific to the workstation, and can be set to any point in the future or the past. This is very convenient for procedures such as ending Sales Order entry for one calendar day at the end of the first shift, and then entering Sales Orders by the second shift dated to the next calendar day. You set the Work Date in the Classic Client by selecting Tools | Work Date (see following screenshot).
In the Role Tailored Client, you can set the Work Date by selecting Microsoft Dynamics NAV | Set Work Date, and then entering a date (see following screenshot).
License: A data file supplied by Microsoft that allows a specific level of access to specific object number ranges. NAV licenses are very clever constructs which allow distribution of a complete system, all objects, modules, and features (including development) while constraining exactly what is accessible and how it can be accessed. Each license feature has its price. Microsoft Partners have access to "full development" licenses to provide support and customization services for their clients. End-user firms can also purchase licenses allowing them developer access to NAV.
For various application functions, NAV uses terminology that is more akin to accounting terms than to traditional data processing terminology. Some examples are:
Journal: A table of transaction entries, each of which represents an event, an entity, or an action to be processed. There are General Journals for general accounting entries, Item Journals for changes in inventory, and so on.
Ledger: A detailed history of transaction entries that have been processed. For example, General Ledger, a Customer Ledger, a Vendor Ledger, an Item Ledger, and so on. Some Ledgers have subordinate detail ledgers, typically providing a greater level of date plus quantity and/or value detail.
Posting: The process by which entries in a Journal are validated, and then entered into one or more Ledgers.
Batch: A group of one or more Journal entries that were posted in one group.
Register: An audit trail showing a history, by Entry No. ranges, of the Journal Batches that have been posted.
Document: A formatted report such as an Invoice, a Purchase Order, or a Check, typically one page for each primary transaction.