This section introduces you about how to install AWS SDK for Node.js and how to create S3 buckets, put objects, get objects using the sample code, and explains how the sample code runs as well.
AWS SDK for JavaScript is available for browsers and mobile services, on the other hand Node.js supports as backend. Each API call is exposed as a function on the service.
To get started with AWS SDK for Node.js, it is necessary to install the following on your development machine:
Node.js (http://nodejs.org/)
Proceed with the following steps to install the packages and run the sample application. The preferred way to install SDK is to use npm, the package manager for Node.js.
Download the sample SDK application:
$ git clone https://github.com/awslabs/aws-nodejs-sample.git $ cd aws-nodejs-sample/
Run the sample application:
$ node sample.js
The sample code works as shown in the following diagram; initiating the credentials to allow access to Amazon S3, creating a bucket in a region, putting objects into the bucket, and then, finally, deleting the objects and the bucket. Make sure that you delete the objects and the bucket yourself after running this sample application because the application does not delete the bucket:
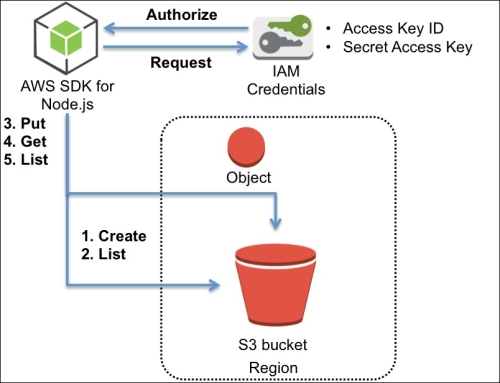
Now, let's run the sample application and see the output of the command, as shown in the following screenshot, and then follow the source code step by step:

Now, let's examine the sample code; the path is aws-nodejs-sample/sample.js
. The AWS.S3
method creates an AWS client:
var s3 = new AWS.S3();
The createBucket
method creates an S3 bucket with the specified name defined as the bucketName
variable. The bucket is created in the standard US region, by default. The putObject
method uploads an object defined as the keyName
variable into the bucket:
var bucketName = 'node-sdk-sample-' + uuid.v4(); var keyName = 'hello_world.txt'; s3.createBucket({Bucket: bucketName}, function() { var params = {Bucket: bucketName, Key: keyName, Body: 'Hello World!'}; s3.putObject(params, function(err, data) { if (err) console.log(err) else console.log("Successfully uploaded data to " + bucketName + "/" + keyName); }); });
The whole sample code is as follows:
var AWS = require('aws-sdk'); var uuid = require('node-uuid'); var s3 = new AWS.S3(); var bucketName = 'node-sdk-sample-' + uuid.v4(); var keyName = 'hello_world.txt'; s3.createBucket({Bucket: bucketName}, function() { var params = {Bucket: bucketName, Key: keyName, Body: 'Hello World!'}; s3.putObject(params, function(err, data) { if (err) console.log(err) else console.log("Successfully uploaded data to " + bucketName + "/" + keyName); }); });
AWS SDK for the Node.js sample application, available at https://github.com/aws/aws-sdk-js
Developer Guide available at http://docs.aws.amazon.com/AWSJavaScriptSDK/guide/index.html
The API documentation available at http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/index.html