Before sketching the Java EE architecture, let's take a quick look at the process through which the standard is created.
Java EE
Java Community Process
Java EE is a standard designed for building enterprise applications with the Java programming language. It contains a number of specifications, which define functionalities required by implementations of the standard.
Specifications that constitute Java EE are developed in an open, community-based process. Both organizations and individual users can join it and take part in the development.
As a standard, Java EE may possess multiple implementations. A vendor who is willing to create a product that is Java EE-certified has to pass a technology compliance test, which guarantees that the product is in alignment with the standard.
The standard provides the contract between enterprise application developers and the vendors of standard implementations. An application developer can be sure that their application will be supported and portable, as there are a number of standard implementations; they are not dependent on one vendor. Application developers are free to migrate their applications between different standard implementations.
It is also important to note that the standard does not determine the details of server implementation. As a result, vendors have to compete to provide the most efficient, robust, and easy-to-use implementation.
To sum up, the Java EE standard provides enterprise application developers with an ability to write supported and portable applications. Furthermore, the community-based specification development process and competition between vendors help the standard to evolve and allows users to choose the best implementation for their needs.
On the flip side, the fact that Java EE is a standard implementation result in a slower evolution and decision-making process than alternative frameworks. In a world in which technology is being developed rapidly, this becomes a bigger problem. As a result, recently, an effort has been made to refactor the way in which standards and specifications are created. Java EE is currently transforming into EE4J, a standard developed under Eclipse Foundation's governance. We will return to this topic in the final Chapter 12: Future Directions.
The basic architecture of Java EE applications
Java EE applications are written in the Java language and run on Java virtual machine (JVM). On top of the standard Java SE functionality, the Java EE implementation provider implements a number of services, which can be used by those applications. Examples of such services may be security, transactions, or dependency injection.
Applications don't interact with enterprise services directly. Instead, the specifications define the component and containers concepts. Components are software units written in the Java language and configured and built in a similar way to standard Java classes. The difference is that metatada provided with the component allows it to be run using a runtime provided by the Java EE implementation. Such a runtime, which may differ for the different types of component, is called a container. The container is responsible for providing access to all enterprise services required by the component.
As an example, let's take a look at the following component:
package org.tadamski.examples.javaee;
import org.tadamski.examples.java.ee.model.Foo;
import javax.ejb.Stateless;
import javax.enterprise.event.Event;
import javax.inject.Inject;
import javax.persistence.EntityManager;
//1
@Stateless
public class FooDaoBean implements FooDao {
//2
@Inject
private EntityManager em;
public void save(Foo foo) throws Exception {
//3
em.persist(foo);
}
}
The preceding script presents an ejb component (1), that is, FooDaoBean, which is responsible for saving objects of the Foo type into the database.
The ejb container in which this component will run will be responsible for pooling instances of this component and managing the lifecycle for all of them. Furthermore, this concrete component takes advantage of the number of enterprise services: dependency injection (2), ORM persistence (3), and transactions (the default for this kind of component).
In general, the goal of the Java EE runtime is to take care of all technical aspects of enterprise applications so that the application developer can concentrate on writing business code. The preceding example demonstrates how it is realized in Java EE: the application developer writes their code using POJOs with minimal configuration (provided mostly by annotations). The code written by an application developer implements business functionalities declaratively, informing middleware about its technical requirements.
The scope of the Java EE standard
Traditionally, business applications written in the Java EE technology were based on a three-tier architectures, web, business, and enterprise information system tier:
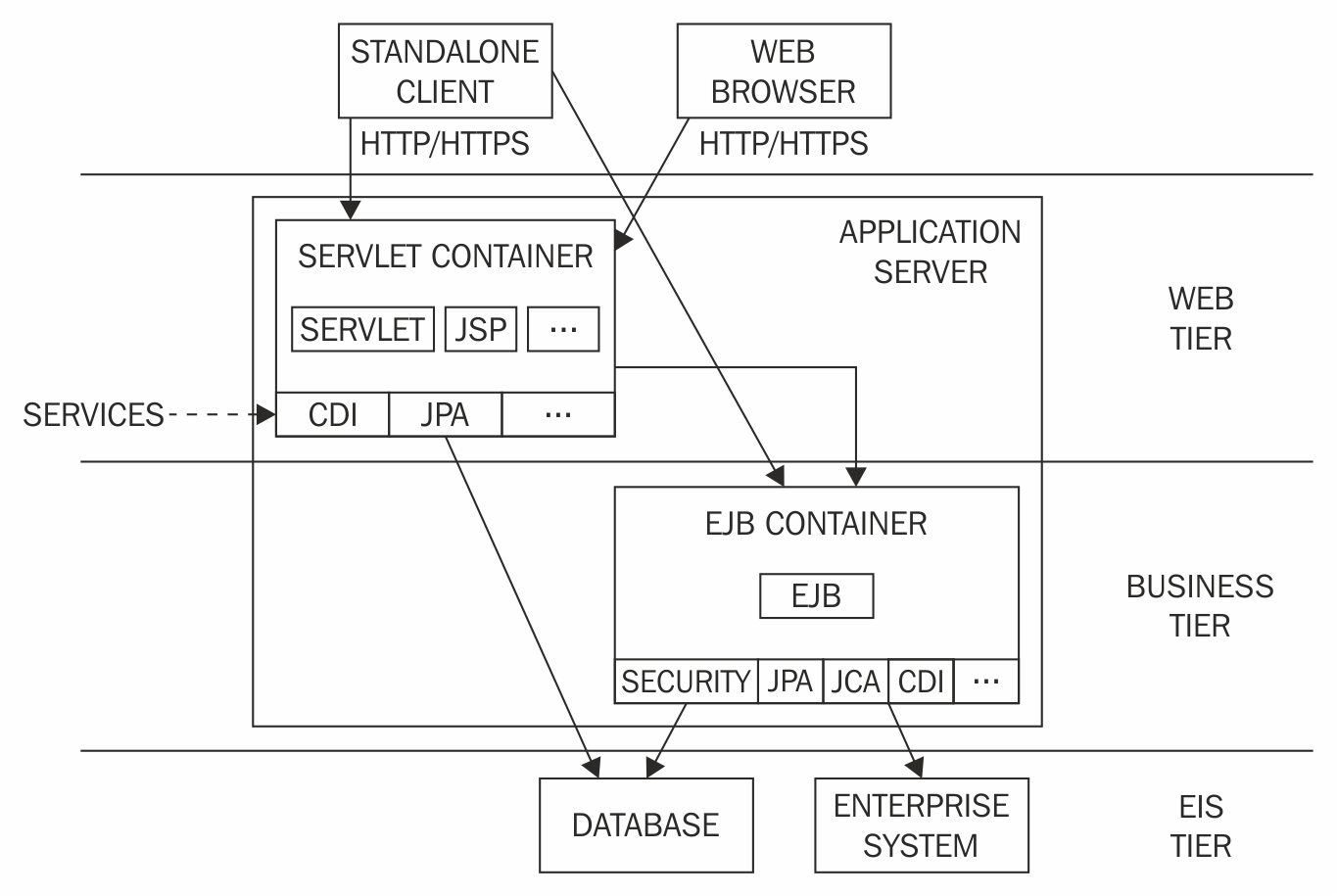
Application server implements web and business tiers. It can be accessed by various types of clients
Web components, such as Servlets, JSPs, or JAX-RS, allow for the implementation of the web layer. They are able to respond to the HTTP requests from different kinds of clients. For example, JSF may be used to create web user interfaces in a convenient way, whereas the JAX-RS API allows for the implementation of RESTful services.
The business layer is implemented by EJBs, pooled POJO-based components that allow for the easy implementation of transactional operations and that can provide a wide array of capabilities such as security, database, external system integration, remote access, or dependency injection.
Although the bird's-eye view architecture is quite straightforward, it is very elastic and allows for the implementation of a wide array of enterprise applications. Moreover, the standard has evolved throughout the years, providing tools for a wide array enterprise usage.
If you take a look at Java EE specification (Further Reading, link 1) you will be able to see all the specifications that are part of the standard. The shared amount of them may be intimidating at first slight. It should be noted that, in most cases, you will have to deal with only a subset of those. On the other hand, when your applications require any kind of enterprise functionality, it is highly probable that the needed tool is already there for you—integrated with the whole platform and easy to use.
Implementation of Java EE standard
Java EE standard implementations are runtimes that allow us to run the components and provide them with the services specified in the Java EE standard. Such runtimes are called application servers.
Application developers create components based on the specification. Those components are assembled into archives, which can be deployed on application servers.
Application servers allow for the deployment of a number of applications. Furthermore, as hinted at the beginning of this chapter, an application can change the server implementation and deploy archives using the application server from the other vendor:
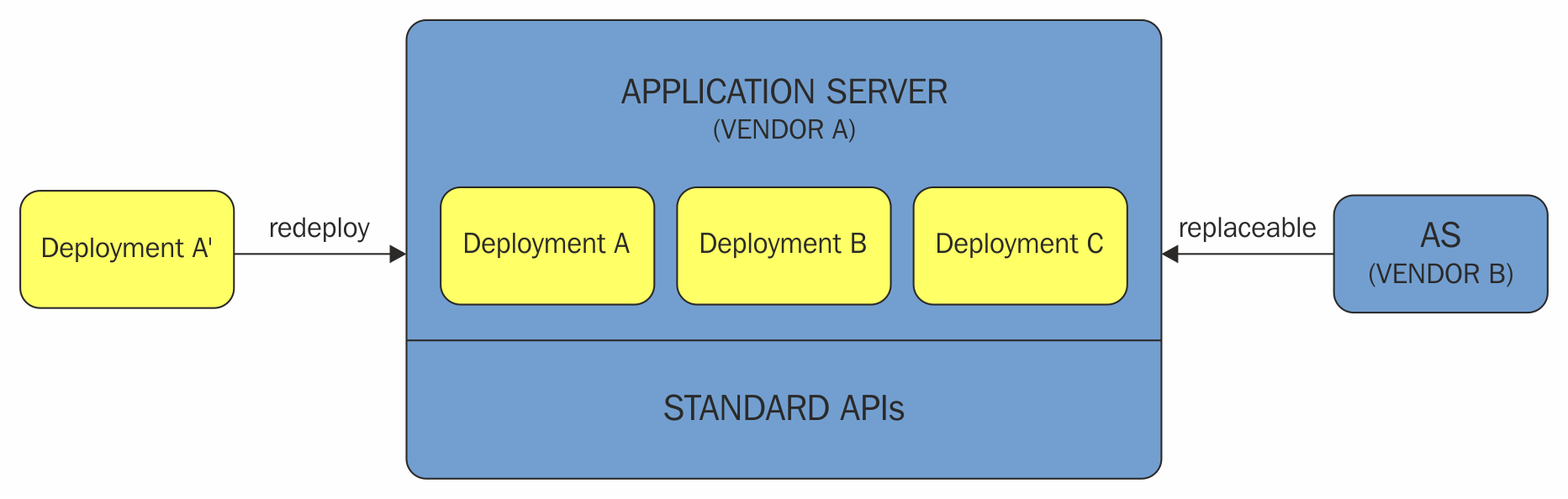