Create a new function app called VerifyUserEmail in a new folder and install Durable Functions, Cosmos DB, and SendGrid:
func extensions install -p Microsoft.Azure.WebJobs.Extensions.DurableTask -v 1.6.2 dotnet add package Microsoft.Azure.WebJobs.Extensions.SendGrid --version 3.0.0 dotnet add package Microsoft.Azure.WebJobs.Extensions.CosmosDB --version 3.0.2
Figure 3.58: Durable Functions project
Add a function called UserAdded using the CosmosDBTrigger. It will all be templated out by VS Code, but the code is here, too:
using System.Collections.Generic; using Microsoft.Azure.Documents; using Microsoft.Azure.WebJobs; using Microsoft.Azure.WebJobs.Host; using Microsoft.Extensions.Logging; namespace VerifyUserEmail.OrchestrationTriggers { public static class UserAdded { [FunctionName("UserAdded")] public static void Run([CosmosDBTrigger( databaseName: "serverless", collectionName: "users", ConnectionStringSetting = "AzureWebJobsStorage", LeaseCollectionName = "leases")]IReadOnlyList<Document> input, ILogger log) { if (input != null && input.Count > 0) { log.LogInformation("Documents modified " + input.Count); log.LogInformation("First document Id " + input[0].Id); } } } }
Your function should look as follows:
Figure 3.59: Durable Functions project
Turn this into an orchestrator trigger that triggers an orchestrator called OrchestrateVerifyUserEmailWorkflow by adding another argument of type DurableOrchestrationClientBase:
[OrchestrationClient] DurableOrchestrationClientBase orchestrationClientBase,
Figure 3.60: Durable Functions project
Add the orchestrator called OrchestrateVerifyUserEmailWorkflow that triggers an activity called SendUserEmailVerificationRequest to send an email to the user's email address with a link for them to click on (use exactly the same pattern that we used in Exercise 11, Error Notifications with Durable Functions, again):
Figure 3.61: Durable Functions project
Create a SendUserEmailVerificationRequest activity that sends the user an email with a link to a function called VerifyEmailAddress. The quickest way to test this would be by using localhost, but you can use either localhost or the deployed version of your app. You function should look as follows:
Figure 3.62: Durable Functions project
Create an HTTP triggered function called VerifyEmailAddress that emits an EmailVerified event upon a GET request to the address that was sent out in the email:
Figure 3.63: Durable Functions project
Modify the OrchestrateVerifyUserEmailWorkflow orchestrator to wait for either a timer or the EmailVerified event and call either an activity called SendUserSuccessMessage or SendUserFailureMessage, depending on the result. Your orchestrator should now look as follows:
Figure 3.64: Durable Functions project
Create an Activity called SendUserSuccessMessage and send the user an email with a successful message. Copy and paste the activity and rename it SendUserFailureMessage before changing the message to a failure message:
Figure 3.65: Durable Functions project
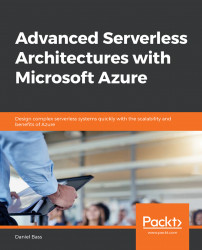
Advanced Serverless Architectures with Microsoft Azure
By :
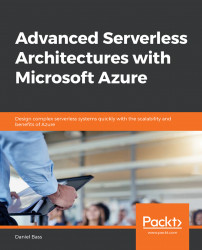
Advanced Serverless Architectures with Microsoft Azure
By:
Overview of this book
Advanced Serverless Architectures with Microsoft Azure redefines your experience of designing serverless systems. It shows you how to tackle challenges of varying levels, not just the straightforward ones. You'll be learning how to deliver features quickly by building systems, which retain the scalability and benefits of serverless.
You'll begin your journey by learning how to build a simple, completely serverless application. Then, you'll build a highly scalable solution using a queue, load messages onto the queue, and read them asynchronously. To boost your knowledge further, the book also features durable functions and ways to use them to solve errors in a complex system. You'll then learn about security by building a security solution from serverless components. Next, you’ll gain an understanding of observability and ways to leverage application insights to bring you performance benefits. As you approach the concluding chapters, you’ll explore chaos engineering and the benefits of resilience, by actively switching off a few of the functions within a complex system, submitting a request, and observing the resulting behavior.
By the end of this book, you will have developed the skills you need to build and maintain increasingly complex systems that match evolving platform requirements.
Table of Contents (8 chapters)
Preface
Complete Serverless Architectures
Microservices and Serverless Scaling Patterns
Azure Durable Functions
Security
Observability
Chaos Engineering
Customer Reviews