When you write a Terraform configuration file where all the properties are hardcoded in the code, you often find yourself faced with the problem of having to duplicate it in order to reuse it.
In this recipe, we'll learn how to make the Terraform configuration more dynamic by using variables.
Getting ready
To begin, we are going to work on the main.tf file, which contains a basic Terraform configuration:
resource "azurerm_resource_group" "rg" {
name = "My-RG"
location = "West Europe"
}
As we can see, the name and location properties have values written in the code in a static way.
Let's learn how to make them dynamic using variables.
How to do it…
Perform the following steps:
- In the same main.tf file, add the following variable declarations:
variable "resource_group_name" {
description ="The name of the resource group"
}
variable "location" {
description ="The name of the Azure location"
default ="West Europe"
}
- Then, modify the Terraform configuration we had at the beginning of this recipe so that it refers to our new variables, as follows:
resource "azurerm_resource_group" "rg" {
name = var.resource_group_name
location = var.location
}
- Finally, in the same folder that contains the main.tf file, create a new file called terraform.tfvars and add the following content:
resource_group_name = "My-RG"
location = "westeurope"
How it works…
In step 1, we wrote the declaration of the two variables, which consists of the following elements:
- A variable name: This must be unique to this Terraform configuration and must be explicit enough to be understood by all the contributors of the code.
- A description of what this variable represents: This description is optional, but is recommended because it can be displayed by the CLI and can also be integrated into the documentation, which is automatically generated.
- A default value: This is optional. Not setting a default value makes it mandatory to enter a default value.
Then, in step 2, we modified the Terraform configuration to use these two variables. We did this using the var.<name of the variable> syntax.
Finally, in step 3, we gave values to these variables in the terraform.tfvars file, which is used natively by Terraform.
The result of executing this Terraform configuration is shown in the following screenshot:
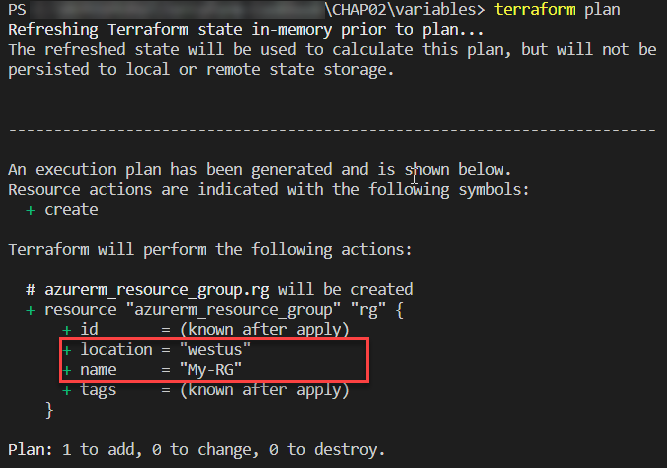
There's more…
Setting a value in the variable is optional in the terraform.tfvars file since we have set a default value for the variable.
Apart from this terraform.tfvars file, it is possible to give a variable a value using the -var option of the terraform plan and terraform apply commands, as shown in the following command:
terraform plan -var "location=westus"
So, with this command, the location variable declared in our code will have a value of westus instead of westeurope.
In addition, with the 0.13 version of Terraform released in August 2020, we can now create custom validation rules for variables which makes it possible for us to verify a value during the terraform plan execution.
In our recipe, we can complete the location variable with a validation rule in the validation block as shown in the following code:
variable "location" {
description ="The name of the Azure location"
default ="West Europe"
validation { # TF 0.13
condition = can(index(["westeurope","westus"], var.location) >= 0)
error_message = "The location must be westeurope or westus."
}
}
In the preceding configuration, the rule checks that if the value of the location variable is westeurope or westus.
The following screenshot shows the terraform plan command in execution if we put another value in the location variable, such as westus2:
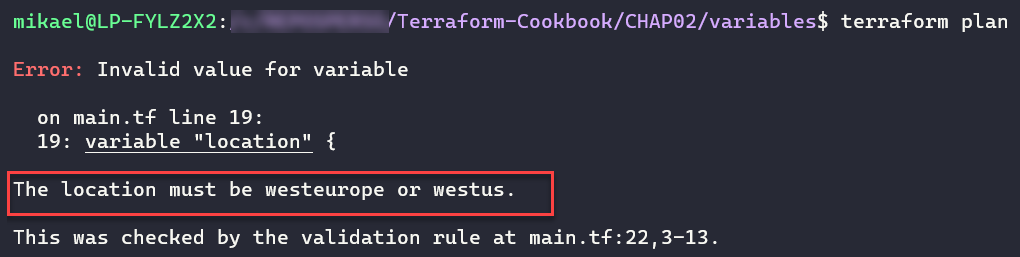
For more information about variable custom rules validation read the documentation at https://www.terraform.io/docs/configuration/variables.html#custom-validation-rules.
Finally, there is another alternative to setting a value to a variable, which consists of setting an environment variable called TF_VAR_<variable name>. As in our case, we can create an environment variable called TF_VAR_location with a value of westus and then execute the terraform plan command in a classical way.
See also
In this recipe, we looked at the basic use of variables. We will look at more advanced uses of these when we learn how to manage environments in the Managing infrastructure in multiple environments recipe, later in this chapter.
For more information on variables, refer to the documentation here: https://www.terraform.io/docs/configuration/variables.html