@PixieApp
: Class annotation that must be added to any class that is a PixieApp.Arguments: None
Example:
from pixiedust.display.app import * @PixieApp class MyApp(): pass
@route
: Method annotation required to denote that a method—which can have any name—is associated with a route.Arguments:
**kwargs
. Keyword arguments (key-value pairs) representing the route definition. The PixieApp dispatcher will match the current kernel request with a route according to the following rules:The route with the highest number of arguments get evaluated first.
All arguments must match for a route to be selected. Argument values can use
*
to denote that any value will match.If a route is not found, then the default route (the one with no argument) is selected.
Each key of the route argument can be either a transient state (defined by the
pd_options
attribute) or persisted (field of the PixieApp class that remains present until explicitly changed).The method can have any number of arguments. When invoking the method, the PixieApp dispatcher will try to match the method argument with the route arguments with the same name.
Return: The method must return an HTML fragment (except if the
@captureOutput
annotation is used) that will be injected in the frontend. The method can leverage the Jinja2 template syntax to generate the HTML. The HTML template has access to a certain number of variables:this: Reference to the PixieApp class (Note that we use
this
instead ofself
becauseself
is already used by the Jinja2 framework itself)prefix: String ID that is unique to the PixieApp instance
entity: The current data entity for the request
Method arguments: All arguments of the method can be accessed as a variable in the Jinja2 template
from pixiedust.display.app import * @PixieApp class MyApp(): @route(key1=”value1”, key2=”*”) def myroute_screen(self, key1, key2): return “<div>fragment: Key1 = {{key1}} - Key2 = {{key2}}”
Example:
Note
You can find the code file here:
https://github.com/DTAIEB/Thoughtful-Data-Science/blob/master/chapter%205/sampleCode25.py
@templateArgs
: Annotation that enables any local variable to be used within the Jinja2 template. Note that@templateArgs
cannot be used in combination with@captureOutput
:Arguments: None
Example:
from pixiedust.display.app import * @PixieApp class MyApp(): @route(key1=”value1”, key2=”*”) @templateArgs def myroute_screen(self, key1, key2): local_var = “some value” return “<div>fragment: local_var = {{local_var}}”
Note
You can find the code file here:
https://github.com/DTAIEB/Thoughtful-Data-Science/blob/master/chapter%205/sampleCode26.py
@captureOutput
: Annotation that changes the contract with the route method, so that it doesn’t have to return an HTML fragment anymore. Instead, the method body can simply output the results as it would in a Notebook cell. The framework will capture the output and return it as HTML. Note that you cannot use Jinja2 template in this case.Arguments: None
Example:
from pixiedust.display.app import * import matplotlib.pyplot as plt @PixieApp class MyApp(): @route() @captureOutput def main_screen(self): plt.plot([1,2,3,4]) plt.show()
Note
You can find the code file here:
https://github.com/DTAIEB/Thoughtful-Data-Science/blob/master/chapter%205/sampleCode27.py
@Logger
: Add logging capabilities by adding logging methods to the class:debug
,warn
,info
,error
,critical
,exception
.Arguments: None
Example:
from pixiedust.display.app import * from pixiedust.utils import Logger @PixieApp @Logger() class MyApp(): @route() def main_screen(self): self.debug(“In main_screen”) return “<div>Hello World</div>”
Note
You can find the code file here:
https://github.com/DTAIEB/Thoughtful-Data-Science/blob/master/chapter%205/sampleCode28.py
Note
You can find the code file here:
https://github.com/DTAIEB/Thoughtful-Data-Science/blob/master/chapter%205/sampleCode28.py
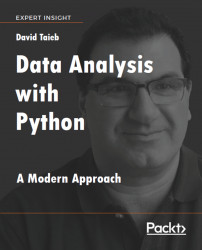
Data Analysis with Python
By :
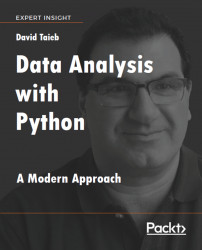
Data Analysis with Python
By:
Overview of this book
Data Analysis with Python offers a modern approach to data analysis so that you can work with the latest and most powerful Python tools, AI techniques, and open source libraries. Industry expert David Taieb shows you how to bridge data science with the power of programming and algorithms in Python. You'll be working with complex algorithms, and cutting-edge AI in your data analysis. Learn how to analyze data with hands-on examples using Python-based tools and Jupyter Notebook. You'll find the right balance of theory and practice, with extensive code files that you can integrate right into your own data projects.
Explore the power of this approach to data analysis by then working with it across key industry case studies. Four fascinating and full projects connect you to the most critical data analysis challenges you’re likely to meet in today. The first of these is an image recognition application with TensorFlow – embracing the importance today of AI in your data analysis. The second industry project analyses social media trends, exploring big data issues and AI approaches to natural language processing. The third case study is a financial portfolio analysis application that engages you with time series analysis - pivotal to many data science applications today. The fourth industry use case dives you into graph algorithms and the power of programming in modern data science. You'll wrap up with a thoughtful look at the future of data science and how it will harness the power of algorithms and artificial intelligence.
Table of Contents (16 chapters)
Data Analysis with Python
Contributors
Preface
Other Books You May Enjoy
Programming and Data Science – A New Toolset
Python and Jupyter Notebooks to Power your Data Analysis
Accelerate your Data Analysis with Python Libraries
Publish your Data Analysis to the Web - the PixieApp Tool
Python and PixieDust Best Practices and Advanced Concepts
Analytics Study: AI and Image Recognition with TensorFlow
Analytics Study: NLP and Big Data with Twitter Sentiment Analysis
Analytics Study: Prediction - Financial Time Series Analysis and Forecasting
Analytics Study: Graph Algorithms - US Domestic Flight Data Analysis
The Future of Data Analysis and Where to Develop your Skills
PixieApp Quick-Reference
Index
Customer Reviews