We will start by creating a new Swift playground. As the name suggests, a playground is a place where you can play around with code. With Xcode open, navigate to File | New | Playground from the menu bar, as shown here:
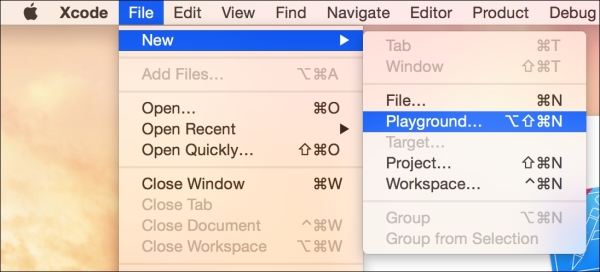
Name it MyFirstPlayground
, leave the platform as iOS, and save it wherever you like.
Once created, a playground window will appear with some code already populated inside it for you:
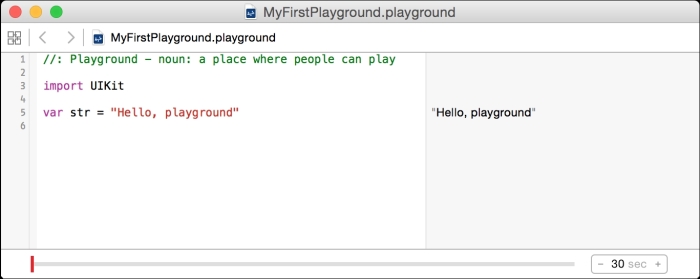
You have already run your first Swift code! A playground in Xcode runs your code every time you make a change and shows you the code results along the right-hand side of the sidebar.
Let's break down what this code is doing. The first line is a comment that is ignored while being run. It can be really useful to add extra information about your code there inline with it. In Swift there are two types of comments: single line and multiline. Single line comments such as the one in the previous code always start with //
. You can also write comments that span multiple lines by surrounding them with /*
and */
. For example:
/* This is a multi-line comment that takes up more than one line of code */
The second line, import UIKit
, imports a framework called UIKit. UIKit is the name of Apple's framework for iOS development. For this example, we are not actually making use of the UIKit framework, so it is safe to completely remove that line of code.
Finally, on the last line, the code defines a variable called str
that is being assigned to the text "Hello, playground"
. In the results sidebar, next to the last line, you can see that "Hello, playground"
was indeed stored in the variable. As your code becomes more complex, this will become incredibly useful to help you track and watch the state of your code as it is run. Every time you make a change to the code, the results will be updated, showing you the consequences of the change.
If you are familiar with other programming languages, many of them require some sort of line terminator. In Swift, you do not need anything like that.
Another great thing about Xcode playgrounds is that they will show you errors as you type them in. Let's add a third line to the playground:
var str = "Something Else"
On its own, this Swift code is completely valid. It stores the text "Something Else"
into a new variable called str
. However, when we add this to the playground, we are shown an error in the form of a red exclamation mark next to the line number. If you click on the exclamation mark, you are shown the full error:
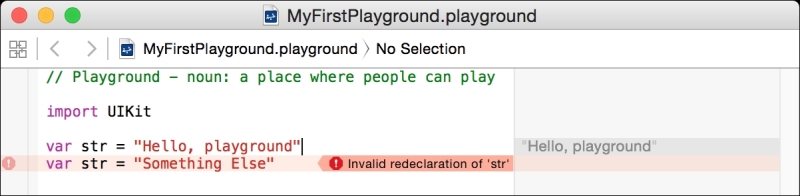
This line is highlighted in red and we are shown the error Invalid redeclaration of 'str'
. This is because you cannot declare two different variables with the exact same name. Also, notice that the results along the right-hand side turned gray instead of black. This indicates that the result being shown is not from the latest code, but from the last successful run of the code. The code cannot be successfully run to create a new result because of the error. Instead if we change the second variable to strTwo
, the error goes away:
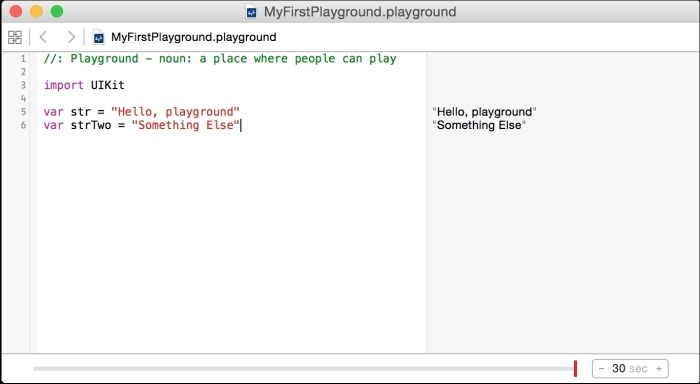
Now the results are shown in black again and we can see that they have been updated for the latest code. If you have experience with other programming environments, the reactiveness of the playground may surprise you. Let's take a peek under the hood to better understand what is happening and how Swift works.