Every programming language has a different approach to handling strings. Erlang does not even have strings. In the C programming language, strings are null-terminated. Strings in C are basically character arrays where \0
states the end of the string. String manipulation errors are common and the result of many security vulnerabilities.
According to Miller and others (1995), 65 percent of Unix failures are due to string manipulation errors such as null-terminated byte and buffer overflow; therefore, handling strings in C should be done carefully.
When you send a message with ZeroMQ, it is your responsibility to format it safely, so that other applications can read it. ZeroMQ only knows the size of the message. That's about it.
It is a common way to use different programming languages in an application. An application written in a programming language that does not add a null-byte at the end of strings and C application code needs to communicate properly otherwise you will get strange results.
You could send a message such as world
as in our example with the null byte, as follows:
zmq_msg_init_data_(&request, "world", 6, NULL, NULL);
However, you would send the same message in Erlang as follows:
erlzmq:send(Request, <<"world">>)
Let's say our C client connects to a ZeroMQ service written in Erlang and we send the message world
to this service. In this case Erlang will see it as world
. If we send the message with the null byte, Erlang will see it as [119,111,114,108,100,0]
. Instead of a string, we would get a list that contains some numbers! Well, those numbers are the ASCII-encoded characters. However, it is not interpreted as a string anymore.
You cannot rely on the fact that a message coming from a ZeroMQ service is safely terminated when you work in C.
Strings in ZeroMQ are fixed in length and are sent without the null byte. So, ZeroMQ strings are transmitted as some bytes (the string itself in this example) along with the length.
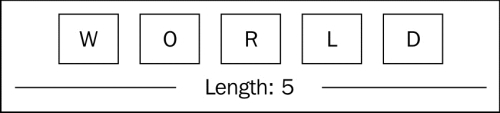
A ZeroMQ string