The order of appearance and importance that we propose here only reflects our personal experience since every project and group of programmers usually have their own agenda when it comes to priorities.
The ultimate reason why you should adopt Scala is readability: code that is similar to plain English will make it easier for anyone (including yourself) to understand, maintain, and refactor it. Scala is unique in that it unifies the object-oriented side that Java has in order to make code modular with the power of functional languages to express transformations concisely. To illustrate how to achieve conciseness by the introduction of anonymous functions (also called lambdas) into the language, take a look at the following line of code:
List(1,2,3,4,5) filter (element => element < 4)
As a Java programmer, the line might look awkward at first since it does not follow the usual pattern of invoking method signatures on classes. A possible Java translation of the previous code could be as follows:
import java.util.*; public class ListFilteringSample { public static void main(String[] args) { List<Integer> elements = Arrays.asList(1, 2, 3, 4, 5); List<Integer> filteredElements = new ArrayList<Integer>(); for (Integer element : elements) if (element < 4) filteredElements.add(element); System.out.println("filteredElements:" + filteredElements); } }
Tip
Downloading the example code
You can download the example code files for all books by Packt Publishing that you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
We first create a List
with five integers, then create an empty List
that will hold the result of the filtering and then loop over the elements of the List
to retain only the ones that match the if
predicate (element < 4
) and finally print out the result. Even if this is straightforward to write, it requires a few lines of code, whereas the Scala line could just be read like the following:
"From the given List
, filter each element such that this element is lower than 4
".
The fact that the code becomes really concise but expressive makes it easier for the programmer to comprehend at once a difficult or lengthy algorithm.
Having a compiler that performs a lot of type checking and works as a personal assistant, is in our opinion, a significant advantage over languages that check types dynamically at runtime, and the fact that Java is a statically-typed language is probably one of the main reasons that made it so popular in the first place. The Scala compiler belongs to this category as well and goes even further by finding out many of the types automatically, often relieving the programmer from specifying these types explicitly in the code. Moreover, the compiler in your IDE gives instant feedback, and therefore, increases your productivity.
Scala integrates seamlessly with Java, which is a very attractive feature, to avoid reinventing the wheel. You can start running Scala today in a production environment. Large corporations such as Twitter, LinkedIn, or Foursquare (to name a few) have done that on large-scale deployments for many years now, followed recently by other big players such as Intel or Amazon. Scala compiles to Java bytecode, which means that performance will be comparable. Most of the code that you are running while executing Scala programs is probably Java code, the major difference being what programmers see and the advanced type checking while compiling code.
To achieve better performance and handle more load, modern Java frameworks and libraries for web development are now tackling difficult problems that are tied to multi-core architectures and the integration with unpredictable external systems. Scala's incentive to use immutable data structures and functional programming constructs as well as its support for parallel collections has a better chance to succeed in writing concurrent code that will behave correctly. Moreover, Scala's superior type system and macro support enable DSLs for trivially safe asynchronous constructs, for example, composable futures and asynchronous language extensions.
In summary, Scala is the only language that has it all. It is statically typed, runs on the JVM and is totally Java compatible, is both object-oriented and functional, and is not verbose, thereby leading to better productivity, less maintenance, and therefore more fun.
If you are now getting impatient to start experimenting with the promising features of Scala that were briefly described previously, this is a good time to open a browser, access the Typesafe page URL at http://www.typesafe.com/platform/getstarted, and download the Typesafe Activator.
The intent of the rest of the chapter is to incrementally introduce some of the basic concepts of Scala by typing commands in an interactive shell and get direct feedback from the compiler. This method of learning by experimentation should feel like a breath of fresh air and has already proven to be a very effective way of learning the syntax and useful constructs of the language. While Scala continues to evolve at École Polytechnique Fédérale de Lausanne (EPFL), many large and small corporations are now taking advantage of the features of the Typesafe platform.
As stated on their website, the Typesafe Activator is "a local web and command-line tool that helps developers get started with the Typesafe platform". We will cover the Activator in more detail in a later chapter dedicated to programming tools, but for now, we will only take the shortest path in getting up and running and get familiar with some of the syntax of the language.
You should now be able to extract the downloaded zip archive to your system in a directory of your choice.
Locate the activator script within the extracted archive and either right-click on it and select Open if you are running Windows or enter the following command in a terminal window if you are on Linux/Mac:
> ./activator ui
In both cases, this will start the Activator UI in a browser window.
In the New application section of the HTML page of the Activator, click on the [Basics] Hello-Scala!
template.
Notice the Location field of the HTML form in the following screenshot. It indicates where your project will be created:
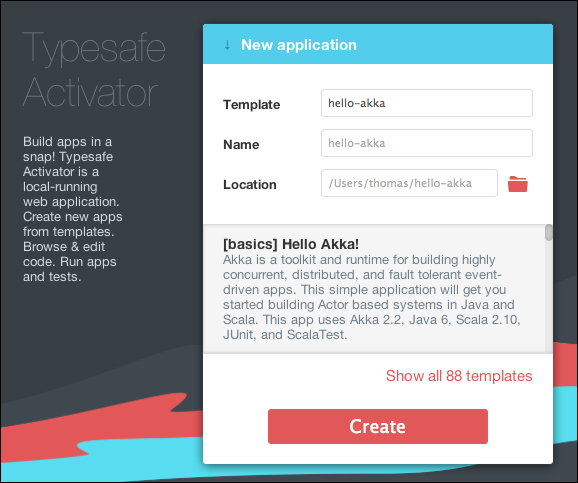
At present, you don't need to pay too much attention to all of the things that happen in the background nor to the generated structure of the project. Out of curiosity, you may click on the Code view & Open in IDE tab and then on the Run tab to execute this Hello World Scala project, which should print, well, "Hello, world !".
Start a terminal window and navigate to the root directory of the hello-scala project that we just created, by entering the following command on the command line (assuming our project is under C:\Users\Thomas\hello-scala
):
> cd C:\Users\Thomas\hello-scala C:\Users\Thomas\hello-scala> activator console
This command will start the Scala interpreter, also known as Scala REPL (Read-Eval-Print-Loop), a simple command-line tool to program interactively.