For the most part, ideas are only applicable in one place. Adding peanut butter is really only a great idea in cooking and not in sewing. However, from time to time, it is possible to find applicability for a great idea outside of its original purpose. This is the story behind design patterns.
In 1977, Christopher Alexander, Sara Ishikawa, and Murray Silverstein authored a seminal book on what they called design patterns in urban planning called A Pattern Language: Towns, Buildings, Construction, Oxford.
The book described a language for talking about the commonalities of design. In the book, a pattern is described by Christopher Alexander as follows:
The elements of this language are entities called patterns. Each pattern describes a problem that occurs over and over again in our environment, and then describes the core of the solution to that problem, in such a way that you can use this solution a million times over, without ever doing it the same way twice.
These design patterns included such things as how to lay out cities to provide a mixture of city and country living or how to build roads in loops as a traffic calming measure in residential areas. This is shown in the following image taken from the book:
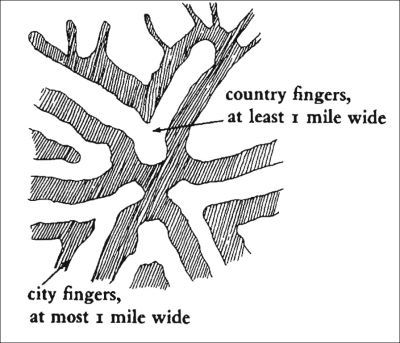
Even for those without a strong interest in urban planning, the book presents some fascinating ideas about how to structure our world to promote healthy societies.
Using the work of Christopher Alexander and the other authors as a source of inspiration, Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides wrote a book called Design Patterns: Elements of Reusable Object-Oriented Software, Addison-Wesley. When a book is very influential in computing science curriculum, it is often given a pet name. For instance, most computing science graduates will know of which book you speak if you talk about "the dragon book" (Principles of Compiler Design, Addison-Wesley, 1986). In enterprise software, "the blue book" is well known to be Eric Evan's book on domain-driven design. The design patterns book has been so important that it is commonly referred do as the Gang of Four (GoF) book for its four authors.
This book outlined 23 patterns for use in object-oriented design. It divided the patterns into three major groups:
The purpose of design patterns is not to instruct you how to build software but rather to give guidance on ways in which to solve common problems. For instance, many applications have a need to provide some sort of an undo function. The problem is common to text editors, drawing programs, and even e-mail clients. Solving this problem has been done many times before, so it would be great to have a common solution. The command pattern provides just such a common solution. It suggests keeping track of all the actions performed in an application as instances of a command. This command will have forward and reverse actions. Every time a command is processed, it is placed onto a queue. When the time comes to undo a command, it is as simple as popping the top command off of the command queue and executing the undo action on it.
Design patterns provide some hints about how to solve common problems such as the undo problem. They have been distilled from performing hundreds of iterations of solving the same problem. The design pattern may not be exactly the correct solution for the problem you have, but it should, at the very least, provide some guidance to implement a solution more easily.
Note
A consultant friend of mine once told me a story about starting an assignment at a new company. The manager told them that he didn't think there would be a lot of work to do with the team because they had bought the GoF design pattern book for the developers early on and they'd implemented every last design pattern. My friend was delighted about hearing this because he charges by the hour. The misapplication of design patterns paid for much of his first-born's college education.
Since the GoF book, there has been a great proliferation of literature dealing with enumerating and describing design patterns. There are books on design patterns that are specific to a certain domain and books that deal with patterns for large enterprise systems. The Wikipedia category for software design patterns contains 130 entries for different design patterns. I would, however, argue that many of the entries are not true design patterns but rather programming paradigms.
For the most part, design patterns are simple constructs that don't need complicated support from libraries. While there do exist pattern libraries for most languages, you need not go out and spend a lot of money to purchase the libraries. Implement the patterns as you find the need. Having an expensive library burning a hole in your pocket encourages blindly applying patterns just to justify having spent the money. Even if you did have the money, I'm not aware of any libraries for JavaScript whose sole purpose is to provide support for patterns. Of course, GitHub has a wealth of interesting JavaScript projects, so there may well be a library on there of which I'm unaware.
There are some who suggest that design patterns should be emergent. That is to say that by simply writing software in an intelligent way one can see the patterns emerge from the implementation. I think that may be an accurate statement; however, it ignores the actual cost of getting to those implementations by trial and error. Those with an awareness of design patterns are much more likely to spot emergent patterns early on. Teaching junior programmers about patterns is a very useful exercise. Knowing early on which pattern or patterns can be applied acts as a shortcut. The full solution can be arrived at earlier and with fewer missteps.