Finish this sentence, by replacing verb and noun with verb and noun 'objects':
I verb up to the noun. It's about 7 or 8 o'clock. I looked at my noun. I was there, to verb on my throne as prince of noun(place).
You just did a Madlibs, demonstrating some of the concepts of OOP. As you can see, it doesn't make much sense to read the sentence as "I verb up to the noun". Since verb and noun are classes, we don't use the actual term verb or noun in the sentence.
So, the idea would be to generate separate objects, one of the class noun and one of the class verb. For example, the previous sentence could be completed like: "I pulled up to the house". Pulled and house are objects that are instances of the verb and noun classes, respectively.
We use words that belong to those classes, which are objects. Another term used when referring to objects is instance, which is used to designate the class the object is derived from, for example, Frodo (a person / hobbit) is an instance of a noun.
The same concept applies to programming. The only thing we can really do with a class is to create an object from it. Objects are derived from classes—you can interact and do things with objects, but not with classes. So, in OpenLayers, we need to create objects from the built in classes to be able to really do anything. The main thing we need are map and layers objects. If we want to create an OpenLayers map, we need a map object, and we create it in the following manner:
var map = new OpenLayers.Map( … );
The new
declaration means that we want to create a new object from the OpenLayers.Map
class. The ellipsis (...
) in the parenthesis presents things we pass into the class to create our object, called arguments
. Each class expects different arguments to be passed into it. It is similar to the Madlibs example—the noun class accepts only certain words. If we were to create a word object in JavaScript, the code may look something like this:
var my_word_object = new Noun('Ironman');
Here, we pass in 'Ironman'
to the noun class, and now we have our word object. Because the Noun class only accepts nouns, this would work fine. If we try to pass in a verb, for instance, it would not work because the noun class cannot accept things that are verbs. Similarly, the Map class expects different arguments to be passed into it than the Layer class does.
There are also subclasses, which are classes derived from another class and inherit all the attributes from the 'base' class it inherits from. Subclasses can also override properties and methods that they inherit.
For instance, let's say we have a Dog class that is a subclass of the Animal class. The Dog class would inherit all the attributes of the base Animal class—such as, perhaps, a speak method. Now, the Dog class would override the speak method and it would bark (or 'yap' annoyingly) when called. The Dog class might also provide additional methods that weren't in the base Animal class, such as, perhaps, a wag_tail method.
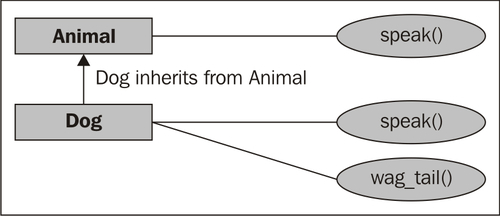
Note
'Base' class and 'Subclasses' are both classes; the terminology just helps to clear up what class inherits from what other class.
There are many subclasses in OpenLayers, for example, the GoogleMap Layer class is a subclass of the base Layer class, and the Navigation control class is a subclass of the base Control class. Subclasses are still classes, and the exact same concept applies; we still need to generate objects from the class to use it.
The previous section was just an introduction to OOP. While you don't necessarily need to know a whole lot more about OOP concepts to use this book, a great resource to learn more about the concepts can be found at http://en.wikipedia.org/wiki/Object-oriented_programming.
Tip
Classes are easy to spot in OpenLayers code. By convention, in OpenLayers (and many other places) class names are CamelCased
, which means the first letter of each word is capitalized, while objects are not. For example, MyClass
would be an example of a class name, while my_object
would be an example of an object.